Cory
Explore posts from serversDTDrizzle Team
•Created by Cory on 2/27/2024 in #help
isNotNull() returning incorrect Type
The following code...
Returns an array of objects where "trashedAt" is a
Date | Null
but this is not possible because I am using where isNotNull()
1 replies
DTDrizzle Team
•Created by Cory on 2/8/2024 in #help
If I pass an object with a property that has 'undefined' as the value, will it be ignored?
If I am updating or inserting data and passing an object, for instance:
Will ID be ignored and not set?
3 replies
DTDrizzle Team
•Created by Cory on 10/20/2023 in #help
How to select all from a table and get the the columns names returned as they are stored?
I want to do a
select()
query on my user table to get emailVerified: boolean('email_verified').default(false).notNull(),
What is returning is emailVerified
as opposed to email_verified
.
I want to return the latter, but also all the rest of the columns.
I know I could do this:
But then if I add anything to the user table, I now have to add it to the select statement, where I might forget.
Is there a way to say get ALL columns but return email_verified
as actually email_verified
? Or return all columns with their actual column names, as opposed to the Drizzle version?6 replies
DTDrizzle Team
•Created by Cory on 9/6/2023 in #help
How to updateNow for datetime?
How can I automatically update a datetime when the row is updated for mySQL?
4 replies
DTDrizzle Team
•Created by Cory on 7/30/2023 in #help
Is there a way to easily convert existing schema's to different DB type?
I have a mySQL schema currently, but wanted to try out libSQL via Turso, but didn't want to have go through my entire schema and queries and change them all manually. Is there some easier way to do this?
1 replies
DTDrizzle Team
•Created by Cory on 7/27/2023 in #help
Difference in using unique() on the column definition vs the index?
What is the different between using something like
name: varchar('name', { length: 256 }).unique()
vs
(t) => ({
unq: unique().on(t.name),
}));)
4 replies
DTDrizzle Team
•Created by Cory on 6/30/2023 in #help
It is possible to have prepared statements inside transactions?
Is there a way to insert prepared queries inside a transaction ?
2 replies
DTDrizzle Team
•Created by Cory on 6/20/2023 in #help
Is there a way to save the generated response Type from a query?
I see that when you hover over the type for the response of a query, you can see what data will be returned. Is there a way to extract, or save that Type somehow, so I can use it elsewhere?
16 replies
DTDrizzle Team
•Created by Cory on 5/25/2023 in #help
Help with this relational query?
I am trying to get all the organizations that a member is associated with.
This code is working:
But it is outputting this:
I know I could just transform this easily with JS, but I wonder if I writing the initial code wrong. I just want an array of organizations.
20 replies
DTDrizzle Team
•Created by Cory on 5/10/2023 in #help
Type error for eq()
I get
Expected 1 arguments, but got 2.
in a statement like this: 4 replies
DTDrizzle Team
•Created by Cory on 5/8/2023 in #help
how to use placeholders for Prepared Insert statements?
Is this possible?
4 replies
DTDrizzle Team
•Created by Cory on 5/7/2023 in #help
Are default values not transferred over via drizzle-zod?
It seems like when I use a
default([])
in my schema
file that when I create a Zod object, I have to re-do the defaults?5 replies
DTDrizzle Team
•Created by Cory on 5/1/2023 in #help
Invalid default value when using defaultNow()
Receiving error:
20 replies
DTDrizzle Team
•Created by Cory on 4/27/2023 in #help
How do I set a column to be unique in drizzle syntax for mySQL?
Would something like this be correct?
wishing there was a .unique()
3 replies
DTDrizzle Team
•Created by Cory on 4/17/2023 in #help
Zod prototype mismatch
Even though the Zod object constructor name created by Drizzle is ZodObject, it is not an instanceof ZodObject. This is causing an error in another library I am using that accepts ZOD objects.
Since I've got Zod as a peer dependency, it should be expected that drizzle-zod is using that version when it creates a Zod objects? I could be off here.
It appears that drizzle-zod has ZOD as a peer dependecy, but drizzle-orm does not?
72 replies
DTDrizzle Team
•Created by Cory on 4/14/2023 in #help
Why is drizzle-zod converting a string to enum?
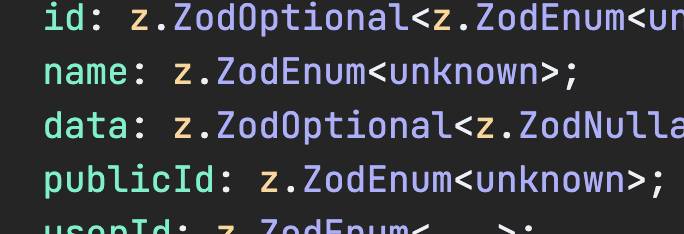
7 replies
DTDrizzle Team
•Created by Cory on 4/10/2023 in #help
Why does drizzle-zod create ZodString's instead of z.ZodStrings for mySQL?
I was testing out drizzle-zod for mySQL, and I know it's still actively being worked on. It seems like it was mostly working but it creates ZodStrings, instead of z.ZodStrings, and is not working with SuperForms for SvelteKit, which accepts a ZOD object.
Should I not even being played around with drizzle-zod for mySQL? Or am I missing something?
3 replies
DTDrizzle Team
•Created by Cory on 4/8/2023 in #help
Is there currently a way to utilize onUpdate?
Trying to run
ON UPDATE NOW()
for a last_updated
column for whenever the row is changed.7 replies