Filip
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Filip on 6/20/2023 in #questions
How to invalidate routes/router in backend/webhook?
Update: the previous solution using a local react component state to store the past user data copy wasn't working as expected. I suspect that the way react batches things together was causing this. I found out that in tanstack that the onSuccess property of the query one can extract the incoming data before it is stored to the variable, and this allows me to make a comparison between the old and new to check for difference ( https://tanstack.com/query/v4/docs/react/reference/QueryCache ). This approach seems to work. See edit in posts above for the updated code. (Also moved the interval state to a context provider and added toast notifications).
8 replies
TTCTheo's Typesafe Cult
•Created by Filip on 6/20/2023 in #questions
How to invalidate routes/router in backend/webhook?
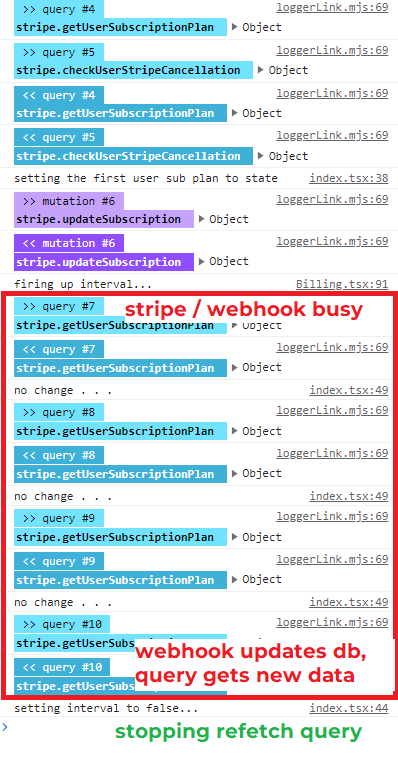
8 replies
TTCTheo's Typesafe Cult
•Created by Filip on 6/20/2023 in #questions
How to invalidate routes/router in backend/webhook?
Ok, so I got an idea looking at one of the examples from Tanstack, and it involves using the refetchInterval option in useQuery. I'll post it here and add explanation of the following code in next post if it can be of help to others.
So on my billing page I have:
In my updateSub component, I have:
8 replies
TTCTheo's Typesafe Cult
•Created by Filip on 6/20/2023 in #questions
How to invalidate routes/router in backend/webhook?
Alright! Thanks! I'll start looking into it and post my solution here once I have something that fits my use case.
8 replies
TTCTheo's Typesafe Cult
•Created by Benja on 4/23/2023 in #questions
Layout management - T3 Stack - Next.js v13
Finally, in index.tsx, I have (which I reckon needs to be replicated for each page that's going to use the layout).
24 replies
TTCTheo's Typesafe Cult
•Created by Benja on 4/23/2023 in #questions
Layout management - T3 Stack - Next.js v13
In my Layout component, I have:
24 replies
TTCTheo's Typesafe Cult
•Created by Benja on 4/23/2023 in #questions
Layout management - T3 Stack - Next.js v13
For anyone who wants to get the layout working with types, I've cobbled together the following through Benja's superb advice above, Nextjs's docs and some other searches. I'm fairly new to TS, take this with a grain of salt but it appears to be working.
Using the pages router, in _app.tsx, I have the following:
24 replies