Řambo
Explore posts from serversTTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
alright thanks
9 replies
TTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
9 replies
TTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
9 replies
TTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
9 replies
TTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
9 replies
TTCTheo's Typesafe Cult
•Created by Řambo on 8/2/2023 in #questions
Improve performance - Avoid Re-renders when changing range input used as a volume slider
9 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
are you the hunter, or the prey?
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
i see, that makes sense. tysm
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
if anyone else knows an easy fix for this, please let me know. otherwise i'll just rename all the files
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
re-named to this
Kai-Sa_Original_FilterOff_MoveFirst_6.ogg
and it works. Wish there was a way for this to work so I won't have to go and change the names29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
idk, maybe
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
so i dont understand why it wouldnt work
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
its using %27 as the single quote
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
cuz looks here. this works just fine
https://static.wikia.nocookie.net/leagueoflegends/images/7/70/Kai%27Sa_Select.ogg
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
is that cloudflare related thing?
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
ya thats what i was thinking.
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
its a single quote
29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
only the ones with
%27
are getting 404 error. the rest work fine29 replies
CDCloudflare Developers
•Created by Řambo on 7/18/2023 in #general-help
Cloudflare R2 public bucket urls with %27 not found
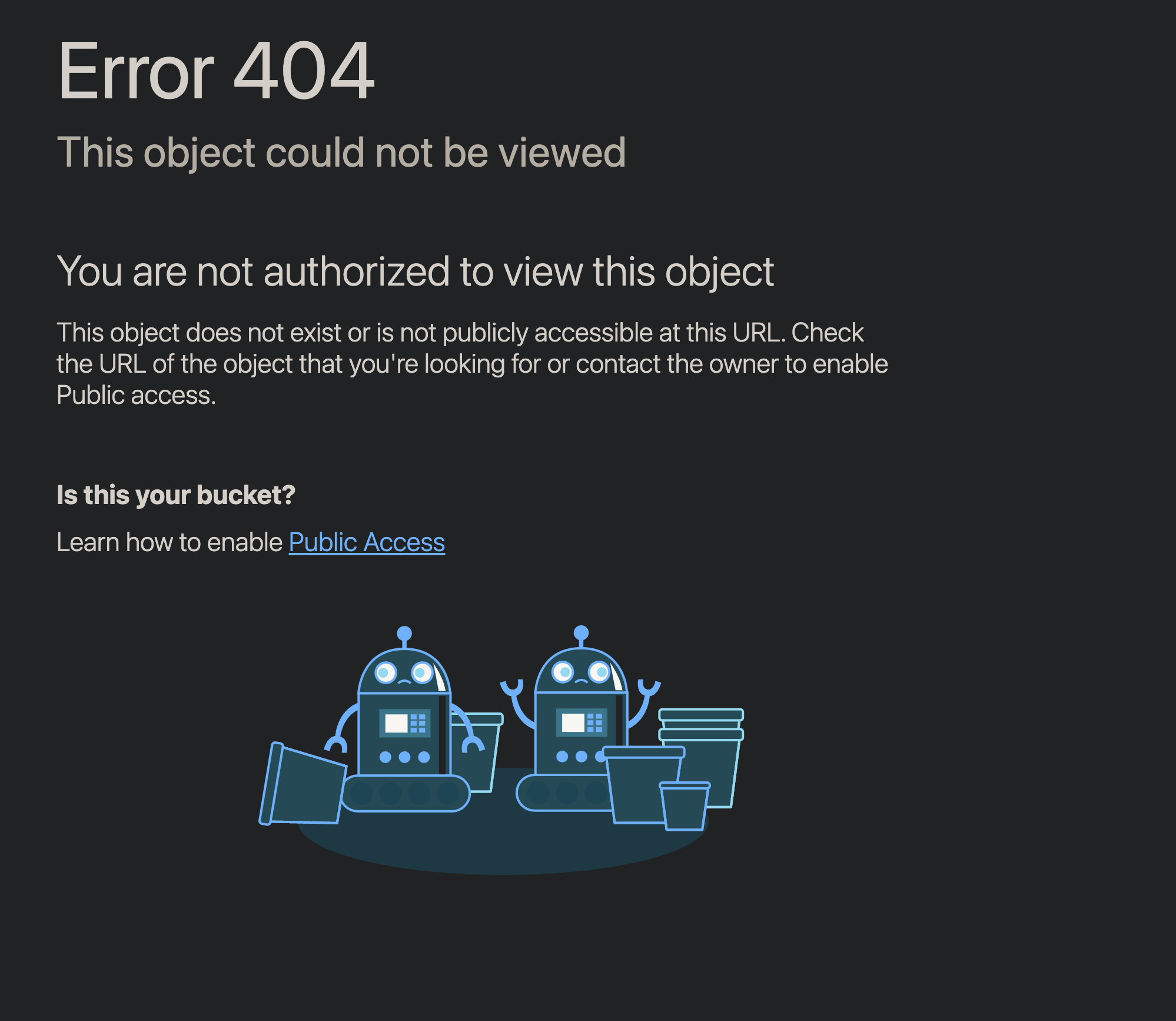
29 replies