rovrav
Explore posts from serversTTCTheo's Typesafe Cult
•Created by rovrav on 9/12/2023 in #questions
Use trpc on nextJS api route?
Is there a way that you can use trpc on a nextJS api route?
7 replies
TTCTheo's Typesafe Cult
•Created by rovrav on 9/8/2023 in #questions
NODE_ENV exposed to the client? Is that okay?
I'm getting ready to test a live app using the t3 stack but basically I want my api routes to switch between localhost for dev and the actual url for live testing.
the env.mjs puts the NODE_ENV as a server variable and I'm not sure why that is the case
below is the code that I'm referring to
If I just make NODE_ENV public will I cause any problems?
18 replies
TTCTheo's Typesafe Cult
•Created by rovrav on 8/24/2023 in #questions
Error Management / Error Tracking tools/service for T3?
I’m working on a SaaS app and am not familiar with the space of error tracking, error handling tools. Is there any that you would recommend?
2 replies
TTCTheo's Typesafe Cult
•Created by rovrav on 7/2/2023 in #questions
Easy way to add rate limiter into t3 stack / trpc?
Is there any recommended way to add a rate limiter into a t3 stack project / trpc?
10 replies
TTCTheo's Typesafe Cult
•Created by rovrav on 5/29/2023 in #questions
useInfiniteQueryBug - queries to the end of page
I set up infinite scroll using the infinite query function in trpc. I have this bug right when the page loads, if you scroll super quick enough to the bottom of the webpage it'll cause a continuous retrigger until it's gone through the entire db. It seems like it's a race condition but I don't know the source of it.
Below is my route
Here is the react code
Any ideas what could be causing the problem?
2 replies
TTCTheo's Typesafe Cult
•Created by rovrav on 10/18/2022 in #questions
Pointing to object in array giving me an undefined error in console.log
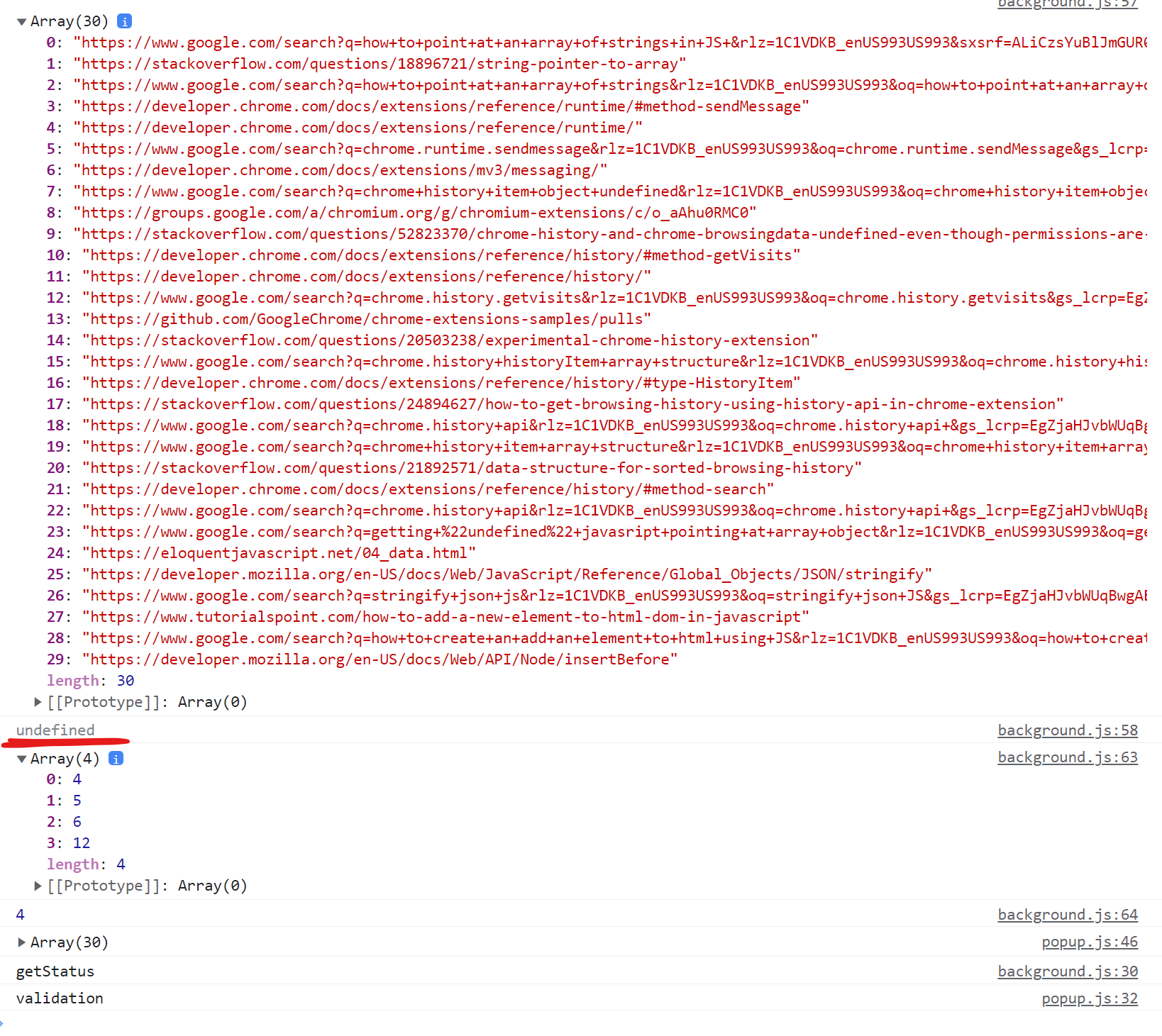
5 replies