theash2473
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
I haven't looked into bare repositories
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
I think I can ignore them then
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
What is git remote add --mirror ? What does mirror do exactly?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
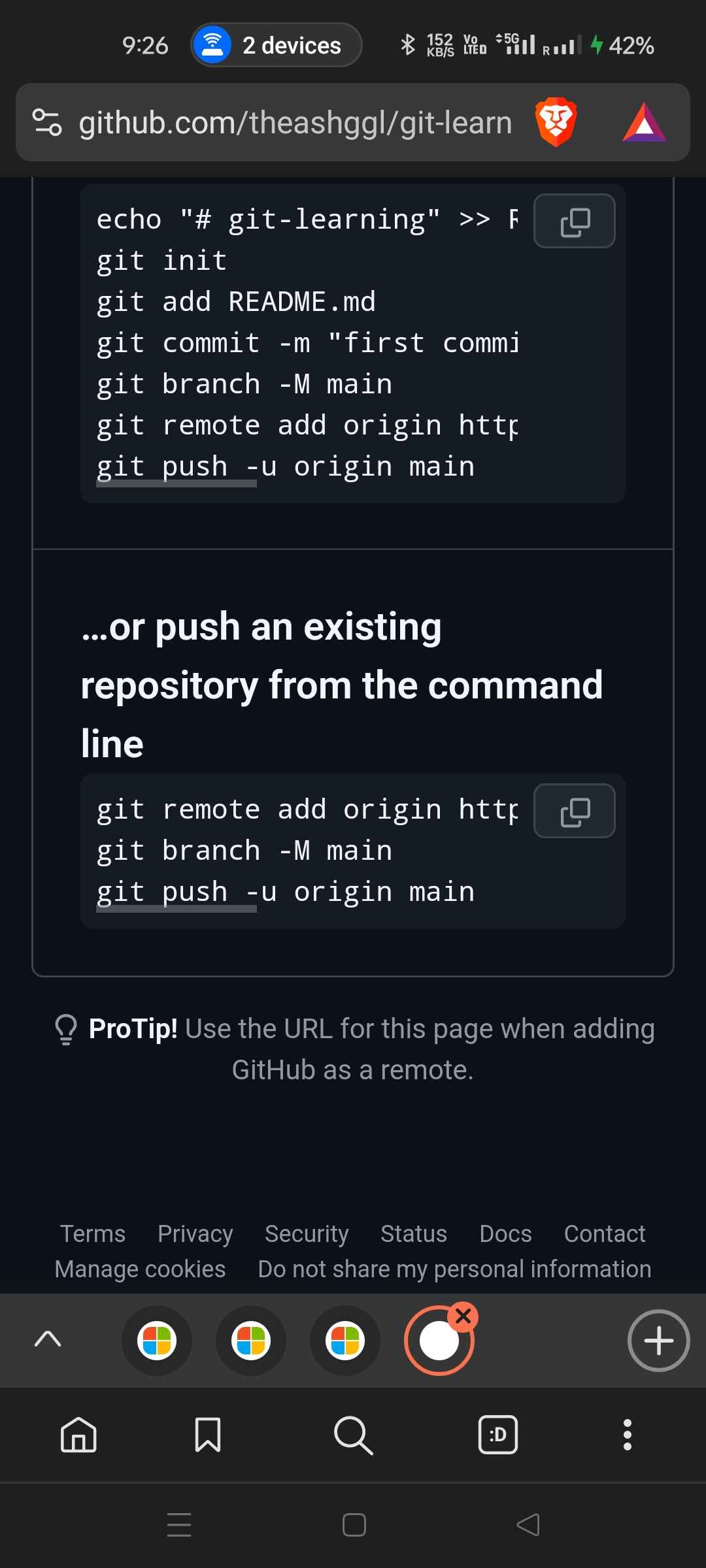
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
On GitHub
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
In the page of a new repository it suggests that command before pushing.
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
no need of renaming right?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
git branch -M main
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
ignore origin. Just talking about the command in a new github repo
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
ohk
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
Also, why does it say in the documentation that -M moves/renames a branch? What does it mean by moving a branch? attaching it to another branch?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
Shouldn't I just upload the repo as is?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
No I don't have any but why do I need to change it?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
What is the use of git branch -M origin if I don't wanna change the names of any branches?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
Will any of the two commands work?
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
It suggests to use set-url instead.
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
Check this out.
https://stackoverflow.com/a/72284434/10841645
73 replies
JCHJava Community | Help. Code. Learn.
•Created by theash2473 on 2/9/2025 in #java-help
github repo deleted. Now cloning local to a new remote repo
Just read this thing
73 replies