garethmoore.
KPCKevin Powell - Community
•Created by garethmoore. on 9/12/2024 in #back-end
How to get around CSP during testing (WebSocket)
I have a simple websocket server that simply alerts each connected user every time a new user joins the server.
I cannot connect to the server because I keep getting CSP violations.
When running this code in the console:
let ws = new WebSocket("ws://localhost:8080");
I get these errors:
Chrome:
Refused to connect to 'ws://localhost:8080/' because it violates the following Content Security Policy directive: "connect-src chrome://resources chrome://theme 'self'".
Firefox:
Content-Security-Policy: The page’s settings blocked the loading of a resource (connect-src) at ws://localhost:8080/ because it violates the following directive: “connect-src https:”
I have tried extensions to disable the CSP. I've tried things like:
connect-src 'self' ws://localhost:8080;
window.open('about:blank').document.write('<script>document.body.style.display="none";</script>');
and more but I've had no luck at all.
I don't know what else to try. Any ideas? Thanks
See server code:
9 replies
KPCKevin Powell - Community
•Created by garethmoore. on 9/28/2022 in #front-end
Odd (to me) behavior of JS objects and console.log()
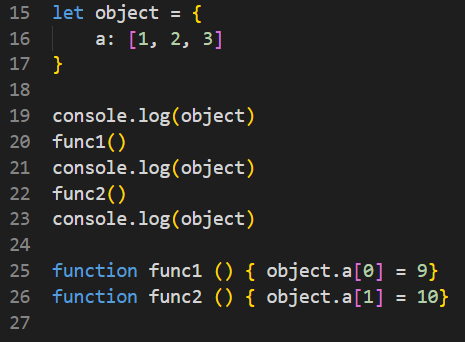
13 replies