eren.
Explore posts from serversJCHJava Community | Help. Code. Learn.
•Created by eren. on 10/3/2024 in #java-help
Help
I am working on a plugin updater / version checker.
I will start by sending the code first so it is easier to explain the issue
Since SkUnityService is loaded before onEnable() the static block errors.
java.lang.NullPointerException: Cannot invoke "me.eren.skriptplus.SkriptPlus.getConfig()" because the return value of "me.eren.skriptplus.SkriptPlus.getInstance()" is null
How would I go about doing this?19 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 7/16/2024 in #java-help
Help with Lombok
I am making utility methods for sending discord webhooks.
my goal is this:
everything works, except for the addEmbed part
my class is defined like this:
so how do i make an embed adder?
14 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 4/5/2024 in #java-help
Help with Gradle
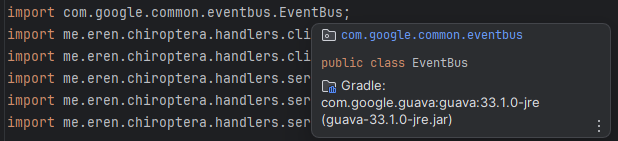
9 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 3/29/2024 in #java-help
Making 2 flavors of a jar
I got 2 folders,
src/core/java/...
and src/bukkit/java/...
I want to setup maven in a way that it will build core first, then use the output as a dependency to build bukkit. The output will be 2 jars16 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 3/21/2024 in #java-help
Quick Help with FileWatcher
I got a filewatcher to detect file changes, but i just cant get
file.isFile()
condition to pass.
the last line throws an exception (I have no permission to edit that class or method)
6 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 3/11/2024 in #java-help
Help with sending a packet
I am making a socket server and trying to send a Server -> Client packet but I am getting a
method ChiropteraServer#broadcast called with (Packet[id=4, data={0=abc, 1=asd}] (Packet)) threw a IllegalBlockingModeException: null
I tried a few stuff but I couldn't get it to work, one of the attempts is the one on github
The sendPacket method: https://github.com/erenkarakal/Chiroptera/blob/master/src/main/java/me/eren/chiroptera/ChiropteraServer.java#L128
!!! ping me if you respond please !!!11 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 3/3/2024 in #java-help
Need help with handling disconnects on my socket server.
I've been looking for a way to properly handle disconnects / timeouts on my socket server for hours. All I wanted to do was log a disconnect message and un-authenticate them if they are authenticated.
Here is my code (see line 79, it currently does nothing):
https://github.com/erenkarakal/Chiroptera/blob/master/src/main/java/me/eren/chiroptera/ChiropteraServer.java
5 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 3/1/2024 in #java-help
Need help with sockets!
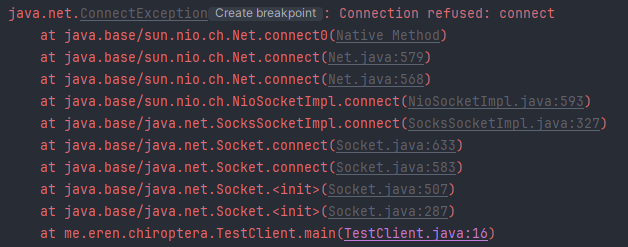
5 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 12/22/2023 in #java-help
Help with registration system
I am making an addon for a scripting language. The way to register a syntax is to make class for the syntax that extends the type of the syntax and call the register method (each register method has different parameters):
it doesn't have to be in a static block and the method doesn't have to be called in that class but I would like to register the syntax in its own class since its easier to manage.
I am looping all classes on enable and registering them using
is there a way to do this better? there has to be
ping me if you respond please!!!
4 replies
JCHJava Community | Help. Code. Learn.
•Created by eren. on 12/13/2023 in #java-help
Feedback on my Registration System
I am making an addon for a scripting language that adds new syntaxes (Skript)
In Skript, each syntax gets its own class and you must call the Skript.registerWhateverSyntax method on server load to use it.
You can bulk register syntaxes using
Skript.loadClasses(package, subpackage)
(assuming you register the syntax on the static block)
but this comes with a problem, you can't create a config file where you can edit what syntaxes are going to be registered, so you need to make your own registration system. I came up with this and I want feedback on it. Perhaps a way without reflection or something cleaner than this?
On server start: https://github.com/erenkarakal/SkCheese/blob/master/src/main/java/me/eren/skcheese/SkCheese.java#L14
Register syntax: https://github.com/erenkarakal/SkCheese/blob/master/src/main/java/me/eren/skcheese/elements/ExprParsedAs.java#L226 replies