Shunrai
[Help] Find Evens or Odds
Bois, I require assisstance.
The test platform for exercises in giving me 3/5 errors and I can't figure out why since they do not provide you with the issue.
Do you have any ideas?
My Code:
You are given a lower and an upper bound for a range of integer numbers. Then a command specifies if you need to list all even or odd numbers in the given range. Use Predicate<T>.
You are given a lower and an upper bound for a range of integer numbers. Then a command specifies if you need to list all even or odd numbers in the given range. Use Predicate<T>.
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
namespace _04._Find_Evens_or_Odds
{
internal class Program
{
static void Main(string[] args)
{
int[] input = new int[2];
input = Console.ReadLine().Split(' ', StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray();
List<int> output = new List<int>();
string command = Console.ReadLine().Trim().ToLower();
Predicate<int> filter = command == "odd" ? new Predicate<int>(x => x % 2 == 1) : command == "even" ? new Predicate<int>(x => x % 2 == 0) : null;
Predicate<int> predicate = i => true;
for (int i = input[0]; i <= input[1]; i++)
{
if (filter(i))
output.Add(i);
}
Console.WriteLine(string.Join(" ", output));
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
namespace _04._Find_Evens_or_Odds
{
internal class Program
{
static void Main(string[] args)
{
int[] input = new int[2];
input = Console.ReadLine().Split(' ', StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray();
List<int> output = new List<int>();
string command = Console.ReadLine().Trim().ToLower();
Predicate<int> filter = command == "odd" ? new Predicate<int>(x => x % 2 == 1) : command == "even" ? new Predicate<int>(x => x % 2 == 0) : null;
Predicate<int> predicate = i => true;
for (int i = input[0]; i <= input[1]; i++)
{
if (filter(i))
output.Add(i);
}
Console.WriteLine(string.Join(" ", output));
}
}
}
13 replies
✅ Attempting to create a method throws CS0116?
Brothers, everything was working fine on the previous projects I started. Now for some reason I am getting CS0116 error on my method that I am trying to create and I can't figure out why. Any ideas?
using System;
namespace _08.MathPower
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
static int OperatorMethod(int a, int b) //This
{
return a+b;
}
}
using System;
namespace _08.MathPower
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
static int OperatorMethod(int a, int b) //This
{
return a+b;
}
}
13 replies
✅ Help with Homework
I have an exercise for homework in order to practice.
I have completed it to the extent of my abilities, however, one of the 10 run tests in the online platform does not provide the required output.
Any ideas? :/
Tony and Andi love playing in the snow and having snowball fights, but they always argue about which makes the
best snowballs. They have decided to involve you in their fray by making you write a program, which calculates
snowball data and outputs the best snowball value.
You will receive N – an integer, the number of snowballs being made by Tony and Andi.
For each snowball you will receive 3 input lines:
• On the first line, you will get the snowballSnow – an integer.
• On the second line you will get the snowballTime – an integer.
• On the third line, you will get the snowballQuality – an integer.
For each snowball you must calculate its snowballValue by the following formula:
(snowballSnow / snowballTime) ^ snowballQuality
In the end, you must print the highest calculated snowballValue.
[Input]
• On the first input line, you will receive N – the number of snowballs.
• On the next N * 3 input lines, you will be receiving data about snowballs.
[Output]
• As output, you must print the highest calculated snowballValue, by the formula, specified above.
• The output format is:
{snowballSnow} : {snowballTime} = {snowballValue} ({snowballQuality})
[Constraints]
• The number of snowballs (N) will be an integer in the range [0…100].
• The snowballSnow is an integer in the range [0…1000].
• The snowballTime is an integer in the range [1…500].
• The snowballQuality is an integer in the range [0…100].
• Allowed working time/memory: 100ms / 16MB
Tony and Andi love playing in the snow and having snowball fights, but they always argue about which makes the
best snowballs. They have decided to involve you in their fray by making you write a program, which calculates
snowball data and outputs the best snowball value.
You will receive N – an integer, the number of snowballs being made by Tony and Andi.
For each snowball you will receive 3 input lines:
• On the first line, you will get the snowballSnow – an integer.
• On the second line you will get the snowballTime – an integer.
• On the third line, you will get the snowballQuality – an integer.
For each snowball you must calculate its snowballValue by the following formula:
(snowballSnow / snowballTime) ^ snowballQuality
In the end, you must print the highest calculated snowballValue.
[Input]
• On the first input line, you will receive N – the number of snowballs.
• On the next N * 3 input lines, you will be receiving data about snowballs.
[Output]
• As output, you must print the highest calculated snowballValue, by the formula, specified above.
• The output format is:
{snowballSnow} : {snowballTime} = {snowballValue} ({snowballQuality})
[Constraints]
• The number of snowballs (N) will be an integer in the range [0…100].
• The snowballSnow is an integer in the range [0…1000].
• The snowballTime is an integer in the range [1…500].
• The snowballQuality is an integer in the range [0…100].
• Allowed working time/memory: 100ms / 16MB
using System;
namespace _11.Snowballs
{
internal class Program
{
static void Main(string[] args)
{
int amount = int.Parse(Console.ReadLine());
double snowballHighestValue = double.MinValue;
int snowballHighestSnow = 0;
int snowballHighestTime = 0;
int snowballHighestQuality = 0;
for (int i = 1; i <= amount; i++)
{
int snow = int.Parse(Console.ReadLine());
int time = int.Parse(Console.ReadLine());
int quality = int.Parse(Console.ReadLine());
if (Math.Pow(snow / time, quality) > snowballHighestValue)
{
snowballHighestSnow = snow;
snowballHighestTime = time;
snowballHighestQuality = quality;
snowballHighestValue = Math.Pow(snow / time, quality);
}
}
Console.WriteLine($"{snowballHighestSnow} : {snowballHighestTime} = {snowballHighestValue} ({snowballHighestQuality})");
}
}
}
using System;
namespace _11.Snowballs
{
internal class Program
{
static void Main(string[] args)
{
int amount = int.Parse(Console.ReadLine());
double snowballHighestValue = double.MinValue;
int snowballHighestSnow = 0;
int snowballHighestTime = 0;
int snowballHighestQuality = 0;
for (int i = 1; i <= amount; i++)
{
int snow = int.Parse(Console.ReadLine());
int time = int.Parse(Console.ReadLine());
int quality = int.Parse(Console.ReadLine());
if (Math.Pow(snow / time, quality) > snowballHighestValue)
{
snowballHighestSnow = snow;
snowballHighestTime = time;
snowballHighestQuality = quality;
snowballHighestValue = Math.Pow(snow / time, quality);
}
}
Console.WriteLine($"{snowballHighestSnow} : {snowballHighestTime} = {snowballHighestValue} ({snowballHighestQuality})");
}
}
}
38 replies
❔ Cannot simulate keystroke using InputInterceptor
Hello,
I am trying to learn how to work with this particular library: https://www.nuget.org/packages/InputInterceptor
I have managed to figure out how to create mouse movements, however, I can't figure out how to send keystrokes, I only get the idea on how to swap keyboard keys.
Line 35 - 36 is the issue I'm having.
Any help is welcome.
'KeyStroke' does not contain a constructor that takes 2 arguments
'KeyStroke' does not contain a constructor that takes 2 arguments
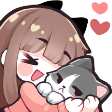
using InputInterceptorNS;
using System;
class Program
{
static void Main(string[] args)
{
if (InitializeDriver())
{
KeyboardHook keyboardHook = new KeyboardHook(KeyboardCallback);
Console.WriteLine("Key simulation started. Press 'V' key to simulate 'H' key. Press 'C' to exit.");
while (true)
{
if (Console.ReadKey(true).Key == ConsoleKey.C)
{
break;
}
}
keyboardHook.Dispose();
}
else
{
InstallDriver();
}
Console.WriteLine("End of program.");
}
static void KeyboardCallback(ref KeyStroke keyStroke)
{
if (keyStroke.Code == KeyCode.V && keyStroke.State == KeyState.Up)
{
InputInterceptor.Send(new[] { new KeyStroke(KeyCode.H, KeyState.Down) });
InputInterceptor.Send(new[] { new KeyStroke(KeyCode.H, KeyState.Up) });
}
}
static bool InitializeDriver()
{
if (InputInterceptor.CheckDriverInstalled())
{
Console.WriteLine("Input interceptor seems to be installed.");
if (InputInterceptor.Initialize())
{
Console.WriteLine("Input interceptor successfully initialized.");
return true;
}
}
Console.WriteLine("Input interceptor initialization failed.");
return false;
}
static void InstallDriver()
{
Console.WriteLine("Input interceptor not installed.");
if (InputInterceptor.CheckAdministratorRights())
{
Console.WriteLine("Installing...");
if (InputInterceptor.InstallDriver())
{
Console.WriteLine("Done! Restart your computer.");
}
else
{
Console.WriteLine("Something... gone... wrong... :(");
}
}
else
{
Console.WriteLine("Restart program with administrator rights so it will be installed.");
}
}
}
using InputInterceptorNS;
using System;
class Program
{
static void Main(string[] args)
{
if (InitializeDriver())
{
KeyboardHook keyboardHook = new KeyboardHook(KeyboardCallback);
Console.WriteLine("Key simulation started. Press 'V' key to simulate 'H' key. Press 'C' to exit.");
while (true)
{
if (Console.ReadKey(true).Key == ConsoleKey.C)
{
break;
}
}
keyboardHook.Dispose();
}
else
{
InstallDriver();
}
Console.WriteLine("End of program.");
}
static void KeyboardCallback(ref KeyStroke keyStroke)
{
if (keyStroke.Code == KeyCode.V && keyStroke.State == KeyState.Up)
{
InputInterceptor.Send(new[] { new KeyStroke(KeyCode.H, KeyState.Down) });
InputInterceptor.Send(new[] { new KeyStroke(KeyCode.H, KeyState.Up) });
}
}
static bool InitializeDriver()
{
if (InputInterceptor.CheckDriverInstalled())
{
Console.WriteLine("Input interceptor seems to be installed.");
if (InputInterceptor.Initialize())
{
Console.WriteLine("Input interceptor successfully initialized.");
return true;
}
}
Console.WriteLine("Input interceptor initialization failed.");
return false;
}
static void InstallDriver()
{
Console.WriteLine("Input interceptor not installed.");
if (InputInterceptor.CheckAdministratorRights())
{
Console.WriteLine("Installing...");
if (InputInterceptor.InstallDriver())
{
Console.WriteLine("Done! Restart your computer.");
}
else
{
Console.WriteLine("Something... gone... wrong... :(");
}
}
else
{
Console.WriteLine("Restart program with administrator rights so it will be installed.");
}
}
}
8 replies
Communication between variables in Multiple Threads
Hello,
I am kinda new to this and I've never really worked with multi threads, decided to finally learn but I can't figure out how I can read the variables of one thread in the other.
C#
void Kekw1()
{
while (true)
{
string image1 = (@"C:\rumblered7.PNG");
string[] results1 = UseImageSearch(image1, "10");
if (results1 == null)
{
MessageBox.Show("null value bro, sad day");
}
else
{
int bananaX1 = Int32.Parse(results1[1]);
int bananaY1 = Int32.Parse(results1[2]);
MessageBox.Show("Banana Results:" + bananaX1 + ", " + bananaY1);
}
}
}
void Kekw2()
{
while (true)
{
string image2 = (@"C:\rumblered2.PNG");
string[] results2 = UseImageSearch(image2, "15");
if (results2 == null)
{
MessageBox.Show("null value bro, sad day");
}
else
{
int bananaX2 = Int32.Parse(results2[1]);
int bananaY2 = Int32.Parse(results2[2]);
MessageBox.Show("Banana Results 2:" + bananaX2 + ", " + bananaY2);
}
}
}
C#
void Kekw1()
{
while (true)
{
string image1 = (@"C:\rumblered7.PNG");
string[] results1 = UseImageSearch(image1, "10");
if (results1 == null)
{
MessageBox.Show("null value bro, sad day");
}
else
{
int bananaX1 = Int32.Parse(results1[1]);
int bananaY1 = Int32.Parse(results1[2]);
MessageBox.Show("Banana Results:" + bananaX1 + ", " + bananaY1);
}
}
}
void Kekw2()
{
while (true)
{
string image2 = (@"C:\rumblered2.PNG");
string[] results2 = UseImageSearch(image2, "15");
if (results2 == null)
{
MessageBox.Show("null value bro, sad day");
}
else
{
int bananaX2 = Int32.Parse(results2[1]);
int bananaY2 = Int32.Parse(results2[2]);
MessageBox.Show("Banana Results 2:" + bananaX2 + ", " + bananaY2);
}
}
}
8 replies