Kiel
Enabling debug/trace logging only for certain parts of code as needed?
Let's say I have services A, B, C, and D, each of which log at different levels (Error, Warn, Info, Debug). By default my logging is set up so that if DEBUG is set (Debugging in Rider/VS), the minimum logging level is set to Debug, and it's not if it's not. When published, services A, B, C, and D will never log their Debug level messages.
Now let's say I determine that service D is producing issues in a way that I could only reasonably diagnose with debug logs. How do I go about enabling Debug-level logging for ONLY service D? I'd like to avoid having to re-compile. Ideally, maybe something I could include in a config file - I am already utilizing a config.json and
AddConfiguration
pattern for other services.
Part of the reason I can only properly diagnose these issues is that some of these chance encounters with bugs only occur as infrequently as once every 21 days, so just running it in Debug mode on my PC for that long would be...very annoying. And if I simply have it logging even in production, I can search the generated logs for debug output and see if I can catch on to anything.
I'm using Serilog with MS.Ext.Logging's strongly-typed(?) loggers if it matters.8 replies
How to publish/deploy a project which has dependencies on a private MyGet feed
Hi all, I've been experiencing issues publishing my project via docker. It fails to restore packages for one or more projects because they take a dependency on a NuGet package hosted on a private MyGet feed. What's the correct and/or best way to alleviate this? I think in the past I directly included the MyGet feed URL in the .csproj file(s) somewhere to indicate where the package was referenced from...but I don't know if that's the best, recommended, or easiest way to do it.
The errors (during
docker build
) all read to the tune of error NU1102: Unable to find package Disqord.Core with version (>= 1.0.0-alpha5.75) [/src/Administrator/Administrator.csproj]
4 replies
✅ How to bulk compress a directory of images until each individual image is under a set size?
I have a directory of 1200 images, some of which are and some of which aren't under 256KB. I need all images that are to be compressed, resized, etc until they are under 256KB with as minimal changes as possible...
I currently have Magick.NET in my project so I'd prefer to use that if possible, but I don't even know where to begin. Should I prefer compression? Resizing? Both? Something else?
3 replies
✅ Creating a collection similar to Channel/Queue that does not "remove" elements from the collection
I have a service in my bot which stores audit logs from Discord as they are relayed across the gateway. I want to store these in memory so that they can be accessed at any point, but I also want to preserve the audit logs as they come in (via a method that waits for an audit log meeting criteria). What are some options available to me?
I don't think I can use Channel or (Concurrent)Queue because they both "remove" elements from themselves as I consume them...unless there's a way to not do that?
2 replies
How to move an item in a "sorted" list above another item, preserving the sorting?
This is less of a C#-specific question, but my brain is really struggling to comprehend this.
I'm operating on a double-sorted collection, where items are sorted first by their
Position
, and then by their Id
if Position
s are equal.
Take the following list (P=Position,I=Id):
Let's say I want to move C between D and E. What do I need to do to ensure that C is now sorted between D and E, given:
- Id
cannot be modified
- Position
can be modified, but cannot go below 1
- Sorting order should be preserved for all other items if possible (IE, E
and above should still be in the same order as before)
- Position
and Id
have no relation, it's just how what I am working with sorts them
Sorry, I know this sounds like some stupid programming class homework, but it's a real life issue I'm dealing with my Discord bot right now with re-ordering roles and I wanted to try to simplify it as much as possible.18 replies
Coupling attachments/files to the database in a portable way.
I understand it's not best practice to store (especially large) attachments/files in a database (PostgreSQL in my case) as it could tank performance depending on how your queries are formatted. I would like to instead maybe store a pointer to the file location on disk, but this isn't portable between devices long term. I don't really have the means or manpower to run my own CDN for a project where only the project itself needs access to the files....what should I do?
One option that might maybe work is to devise and run an API for storing and retrieving attachments on the same network, but I'd be worried about testing and prod referencing the same set of files leading to potential collisions or other potential don't-touch-prod-data issues
27 replies
Concern over inheritance with static interface methods
Consider the following interface:
I have two classes, LuaUser and LuaMember. When I implement the classes like so, I get the following error:
If I add the suggested fix for this, which is creating an implementation of the non-generic
SetUserDataDescriptor
...Will this cause any issues? What should this method do exactly? Currently I am utilizing reflection to get all ILuaModel
implementations, to call SetUserDataDescriptor
, and I just want to know what I should do here to make sure both LuaUser
and LuaMember
have their methods called appropriately.1 replies
More efficient or performant way of serializing permission nodes?
As a personal project, I'm designing a chat platform similar to Discord. Discord's permission system uses a simple bitfield, which is great as it's a small size and simply expressed, but terrible as I'm limited to
n-1
possible permission values where n
is the bit size of the field (I believe Discord internally uses a 64-bit integer, so 63 total possible permissions). I consider this bad for futureproofing and I would like to make sure I'm not painted into a corner and can have any arbitrary number of permission nodes/values. Anyone have any great ideas for alternative ways to format arbitrary permissions in a JSON-serializable-friendly way?
Right now my only genius idea is still using an enum
, but making it essentially an array instead of a bitfield. This would greatly increase the size of the generated JSON most likely, but now I have a massively increased number of permission values. even using just a humble uint
, I'd now have billions, which is more than enough for me.
IE:
strings are probably slower, and obviously take up precious bandwidth at the scale of potentially hundreds or thousands of permissions
arrays, so I may opt for the less-readable but identical int value format...but I'd like to know if anyone with more experience or knowledge has a brighter idea. Maybe something silly like multiple bitfields across multiple field names?16 replies
Real-time chat platform frameworks: SignalR, WebSockets, ???
As a project I want to task myself with to learn some new technologies, I want to develop a chat app a la Discord. I'm curious what technologies are out there that can allow clients to connect to the server and receive "events" for things like messages, etc. etc. I know of WebSockets, which are probably the most common - but I'm curious what other tools or frameworks I could utilize on the server side that can still be easily accessed by cross-platform (mobile, desktop) clients. I'm looking for backend-only systems if preferable as I will likely try to build the clients (once I get to that point) in something else entirely.
I would also like to maybe do a little bit of premature overengineering and build it so that it could easily scale beyond just running it on one random computer for the entire world. I'm trying to be realistic and knowing that this is just a pet project I don't plan on trying to release it in a state to scale or compete with huge platforms, but I'd like to learn as maybe it could be something I could add to my portfolio. I have a feeling if I design it for small-scale, that upscaling it down the line will be a huge pain.
51 replies
Using reflection to build a dictionary of methods -> attributes at runtime
I'd like the following setup to work:
Is this possible? While trying to find out how to do this at all I found that I could use something similar to
new StackFrame(1).GetMethod()
to retrieve the calling method, adjusting the 1
to the level of nesting in the method, but this is 1) extra hacky IMO and 2) seems like it has serious negative performance impacts.
Something I have considered is using [CallerMemberName]
to make it a lookup based solely on method names, but as I've just started defining all these methods I have no clue if I will be making methods with overloads, IE ending up with two or more methods with the same name, making the lookup not possible.3 replies
Deserializing 3rd-party JSON into a base (abstract) class in System.Text.Json
What is the most performant way to accomplish this? The most common solution to this problem (in Json.NET anyway?) is to implement a custom JsonConverter which first converts the object to a JObject, then checks for a discriminator property (or properties) before deciding how to deserialize/convert the JObject. This works, clearly, but I have doubts/concerns about its performance at scale as far as speed is concerned. I really, REALLY would like to avoid anything involving shoving every single possible field from the derived classes into one massive model abomination. Changing the JSON is out of the question as this is third-party data coming in from a websocket.
The data is pretty much
followed by SomeType-specific event fields.
type
is guaranteed to exist. Could I use this field as a discriminator somehow?
My concern is that solutions involving JsonConverter delve into solutions almost always including a switch statement on the discriminator property. My issue is that this type
field can have...almost 35 values, and I'm sure even more as the events this websocket can send to me will expand with time as well. The idea of a 35-case switch statement doesn't sit well with me4 replies
ClientWebSocket hangs on ReceiveAsync, never continues
I will concede I am brand new to working with websockets. I'm following this Establishing a connection guide which uses websockets for connection. One of the recommended ways for initiating a connection is to connect normally, and then send an Authenticate event to the server upon which you will receive either an Error or Authenticated event.
In an obviously rough draft attempt to accomplish this feat:
my
_ws
is a class which wraps ClientWebSocket, and I can confirm my data is being sent (at least, no exception is thrown), but then when I try to receive, it never advances. Even stepping into it and breakpointing the actual ClientWebSocket#ReceiveAsync
method, it never advances beyond this point. Is there a trick I'm missing?29 replies
❔ Reducing Result-pattern annoyances
I know Result patterns can be a bit controversial as far as their benefits, but I'm giving them a try in my project.
Anyways...I've outsourced some work to a scoped service which returns a Result<T>. My methods which call this outsourced code have this exact same structure:
Are there any ways to reduce this into a simpler form to further reduce the amount of code needed to perform the "try action, return error message if error else get success value" process? I certainly will concede I think I'm splitting hairs here and this is already simple enough for some, but I feel like I'm...missing something to make this more streamlined - maybe pattern matching could be utilized here?
9 replies
❔ Automatic discovery of scoped services without a marker interface
In the past, I had an
IService
interface which defined a (singleton at the time) service and a contract for certain service-level init functionality etc.
This was coupled with an extension method which used reflection to find all IService
implementation types and register them with my service provider at startup.
I'm trying to do the same thing with scoped services, but I'm struggling to think of ANYTHING to put on this prospective IScopedService
interface, and I always feel like marker interfaces are...smelly. Any suggestions? Or should I just ignore my gut feeling and be happy with a marker interface for this extremely specific usecase?8 replies
❔ AuthZ/AuthN with ASP.NET Minimal APIs
What's the correct way to do authz/authn with minimal APIs in asp.net? I'm doing both of those things based on the provided Authorization header, which is an API token of the format:
AuthZ will be treating the hashed ID like a username and the cryptographic portion like an auto-generated password to validate a user's identity.
AuthN will be simply validating that the (unhashed) ID matches the ID in the route the user is requesting.
What's the simplest (but still correct) way to do this? the article I read went wayyy over my head and looked designed for much more complicated solutions so maybe if someone else were to explain it I'd hopefully understand.
I'm unsure if it's just as simple as me implementing my own 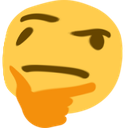
IAuthorizationService
/IAuthenticationService
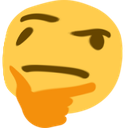
2 replies
❔ Avoiding duplicating code between DB models and DTOs
Hi there. I don't know if "DTO" is the right word to describe what I'm talking about, but...
I have a Discord bot which is also hosting an API to allow other bots/platforms/applications to interact with the bot for some features in a TOS-abiding way. At the present moment, my DB models also have logic attached to them, which I plan to separate in some way to keep the models themselves tidy and readable.
I've been told it's not a best practice to return/serve DB models directly as JSON in API calls, so I am looking to avoid doing that via, what I believe would be DTOs. How do I avoid the unnecessary code duplication in what would effectively be a copy of the model class(es) in the API? My main concern is, even if I couldn't avoid it, any changes to DB models would require me to remember to make an identical modification where appropriate to the API DTOs. This is not something I'd prefer as my current setup already suffers from that issue.
Any suggestions or ideas are welcome. At the moment, I don't see a huge need for DTOs on my bot side of things as I'd be doing the data manipulation behind the scenes and Discord bots don't exactly return data like APIs do...but I do have some pretty complicated logic attached to the current models that I'd either have to extract as extension methods or DTOs...for a second time (bot DTOs / API DTOs)
15 replies
✅ JSON serializing Optional type throws an exception(?)
Newtonsoft.Json.JsonWriterException: Token PropertyName in state Property would result in an invalid JSON object. Path ''.
Here's my JsonConverter:
I'm not quite sure what's going on here as it's difficult for me to debug what JSON is being serialized by the Serialize call or what Json.NET is trying to validate later on. Serializing to a StringBuilder instead of the writer yields a simple string property is being serialized, and yet this exception is being thrown. Google was very unhelpful as one of the only results for this exact error message was for something completely different, and the exception message doesn't really yield any clues.
I found out about ITraceWriter, and here's what it was able to output:
2 replies
❔ A safe scripting language for a Discord bot
Hi there. I'm looking into a system where server owners can design fully custom commands for their server without requiring their own bot, or the main bot to implement it. I've thought about perhaps utilizing Lua for this usecase, but I'm of course concerned about things like privilege escalation or finding a way out of the sandbox, resource exhaustion, and any other issues that would come with letting users effectively run their own code.
Are there any solutions out there that account for this? Lua isn't the only option obviously, I just know it's a common "I want to let users script things without them needing to know the same language the [x] is written in". Some things of note I'd be looking for:
- limiting execution steps or memory usage by the sandbox
- being able to pass in "context" to the sandbox (IE, the channel the custom command was run in, the user executing the command, etc)
17 replies