EXILE
Explore posts from serversTTCTheo's Typesafe Cult
•Created by EXILE on 4/11/2024 in #questions
What is a correct API route validation flow
I'm currently creating a learning platform similar to udemy just for some practice using Next.js (pages), Prisma, auth0, TypeScript, Postgres, Zod and I'm trying to create an API route for creating a new course.
What should the flow look like in this API? (Idk if flow is the right word or if my title makes sense. correct me if I'm wrong)
In order possibly?:
- Check req method
- Check req body w/zod
- Check if session exists
- Check if user exists in db using session user email
- Check if user has correct role (only allowing admins to create courses atm)
- Compare user email to session email
- Create course
I think my requirements seem okay. A user can only make a course if admin. They must have a session. They can't create a course for another user.
2 replies
TTCTheo's Typesafe Cult
•Created by EXILE on 3/20/2023 in #questions
Could I get some help for this Prisma schema?
I'm currently creating a "guess the rank" web-based game where you choose a competitive game, watch a short clip of gameplay and then guess the rank of the player in that clip. It's pretty simple and there's a few websites out there already.
Each game can only have a single clip for that day. I reset the clip at midnight via a cron job and that all works fine. But I'm not sure if the way I'm storing the daily clip for each game is good. I originally thought I could have my Game model contain a featuredClip Clip but game also contains clips Clip[] and I can't figure out how to get this relationship to work. I've tried naming them but it didn't work. Still new to this.
I'm currently trying to clean up my database and I wanted to know if the way I'm currently doing it is fine or should I go for the seconds approach, or even something different.
First approach:
- A clip that is user submitted.
- Contains data about the submitted video file that's stored wherever, the rank, the youtubeId which is only set if I accept the clip and then hit the youtube api to upload the video, acceptedDate, isAccepted etc... You can see this table below.
Then I have CurrentClip which stores the current clip for each game. There can only be one row for each game:
I would prefer if I could just fetch the games, include the featuredClip as mentioned in my first paragraph and then in nextjs simply be able to get the game and do a game.featuredClip to get the current featured clip. I'm just struggling to get that to work if it's possible.
Second approach:
- FeaturedClip is the same as the CurrentClip
Now with this approach is a WIP. I don't know if it's correct so far. In order to fix some relationship errors I've needed to add duplicate rows from the tables. You can see multiple game fields etc..
3 replies
TTCTheo's Typesafe Cult
•Created by EXILE on 11/14/2022 in #questions
Are these Prisma models okay?
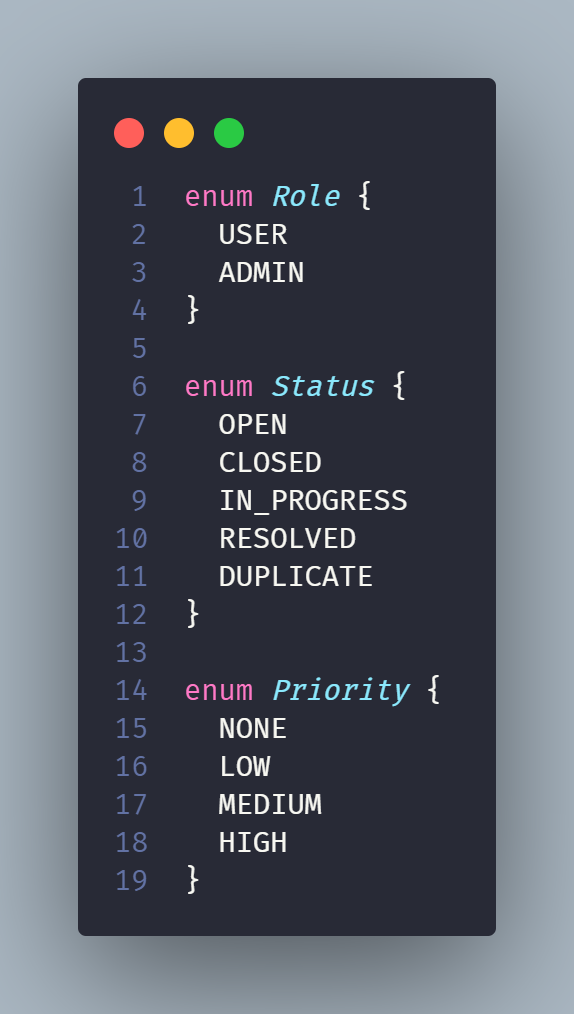
3 replies