webdevkaleem
TTCTheo's Typesafe Cult
•Created by webdevkaleem on 2/12/2025 in #questions
Renaming a file but on download it shows the file's key instead of it's name
Main tech stack:
Nextjs
: 15v, Drizzle orm
: 0.39v, Clerk
: 6v, Uploadthing
: 7v.
Github
: https://github.com/webdevkaleem/zip4you
Description:
So when i upload a file, i generate a random name using cuid2
. After upload is completed, a UI pops up where you can rename
the media. This works flawlessly as the new name is updated inside Uploadthing
and Postgres
. The issue is that when i download
the media from the client UI
, it shows the key
instead of the name
for the media.
But
There is one special case where something else happens. I have a tRPC
procedure (/api/trpc/media.gmail) which gets a request
from zapier
and does it's own thing. Here's the catch. When this is procedure runs, it takes the subject
from the header and set that as the file name
. Now when i download the file from the client UI
it shows the name
correctly.
However
In both cases the updated name isn't reflected when i start a download on the client.37 replies
TTCTheo's Typesafe Cult
•Created by webdevkaleem on 1/23/2025 in #questions
Twitch Re-sub confusion
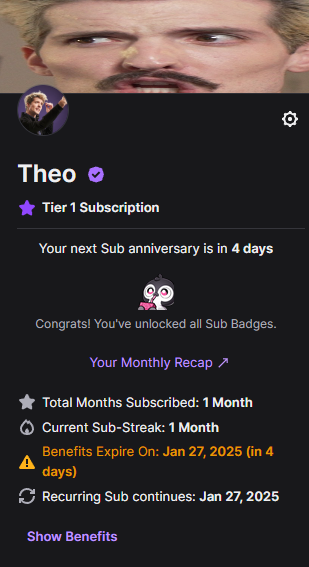
8 replies
TTCTheo's Typesafe Cult
•Created by webdevkaleem on 1/10/2025 in #questions
Uploadthing: Type-error when sending custom field data to the client
So i want to send some data down to the client upon image upload.
api/uploadthing.core.ts
type-error
The imagesArr
is what i want on the client as I loop
over imagesArr
to render all the images.
[Important Point]: In the backend all the images are uploaded successfully and in the right size as well
-------------
12 replies
TTCTheo's Typesafe Cult
•Created by webdevkaleem on 1/10/2025 in #questions
Uploadthing muti image generation from a single image
I want to upload a single image and have 2 copies generated which are smaller than the original image (for mobile 400x400 and tablet 600x600). I want this process to be automatic, when the original image (1000x1000) has been done uploadthing.
Any help on how should i complete this task
(T3 stack: App router, tRPC, uploadthing)
Thanks
2 replies