Online/offline colour menu colour change
Hi all, as the title states I am trying to get the menu items to change colour to green if pingable, red if not pingable. An online/offline thing if you will.
I am very new and still learning. Currently this is what I have, im trying to use the code in the converter.cs and the main functions are in the MainViewModel.cs
any advice is more than welcome!
https://paste.mod.gg/mxpnkbwidxks/3
BlazeBin - mxpnkbwidxks
A tool for sharing your source code with the world!
31 Replies
What constitutes "pingable"? That you can send a
HEAD
or whatever request and get 200 OK
back?
In any case, you probably want to do that on a timer
Short polling, in other wordsOh i see
umm pingable i guess would be if the ipaddress status returns
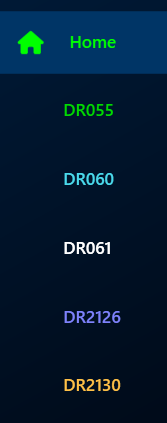
so this is what the menu looks like, forgive the colours i was just mucking around
essentially, they are radio buttons
they would each have their own ip to ping
for example dr055 would be 8.8.8.8 for giggles
60 would be 192.168.0.1 as an example
61 something ridiculous just to fail
the idea was that isPingable would see if it can be pinged, if it can change colour to green
in very simplistic terms
if it can't be pinged be red
So, for each IP address you would have to send the ping on a timer
Ideally, you'd do something like
Somewhat pseudocode-ish, but you get the idea
Then, you'd bind to that list
Things
, instead of manually creating each individual elementyou give me too much credit, i have no idea how to do that.
For the most part i've been following a really old outdated tutorial and it stopped
and i feel like i haven't really learnt anything as its too advanced for me
Microsoft docs are quite great
https://learn.microsoft.com/en-us/dotnet/desktop/wpf/data/how-to-bind-to-a-collection-and-display-information-based-on-selection?view=netframeworkdesktop-4.8 here's on binding to a list
may i ask what goes inside the //properties
The properties 😛
ahh i see
i had this
but i like yours better
it makes sense
Mid you it's still fairly pseudocode-ish, you might need to do the
OnPropertyChanged
thing inside of the Thing
, not 100% sure
MVVM Toolkit is super useful here, though: https://learn.microsoft.com/en-us/dotnet/communitytoolkit/mvvm/wouldn't even know how to use it
im struggling to get this, to be honest
Perhaps start from easier projects, then?
UI apps are fairly complex
this is the final thing
then im done
thats why i wanted it to work
Understandable
so should i modify my code
to match yours
or can i just tweak mine a little
As I said, my code is not copy&paste
It's only to give you a general idea
yeah
Have a list of things, loop over it, check each thing, update the state so it's reflected in the UI
That's the general idea
yup
so i had something like that
that was my little list
and this was my foreach
That seems fine too, yeah
All you're missing is a timer, then
yeah which i can use your way of doing
but the problem was
its not changing colours
not getting any errors either
so im kinda lost
Do some debugging
$debug
Tutorial: Debug C# code and inspect data - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
im thinking my update menu function may be the problem
Place a breakpoint, run the debugger, and see
i'll give it a go
if u match his code u lose OnPropertyChanged which is an impotant piece of code for WPF
it notifies the ui of changes
and you could use a XAML datatrigger that changes from green to red with the IsPingable information
https://wpf-tutorial.com/styles/trigger-datatrigger-event-trigger/
the 2nd example
u could bind an converter as well to the button
I have the converter
@taner. i thought thats what i was trying to do
@Angius you around
Does it implement the IValueConverter interface?