Running into issues with registerApplicationCommands
So I am running into an issue with registerApplicationCommands, for some reason it happens only when the
SlashCommandBuilder
has Subcommands or Group of subcommands. I console logged the data of the SlashCommandBuilder
but still same problem.
Edit: I extended the Subcommand
class to my own liking and added automatic Builder creating upon creating the class within commands. I'll add the screenshot at the bottom cuz i forgot to show it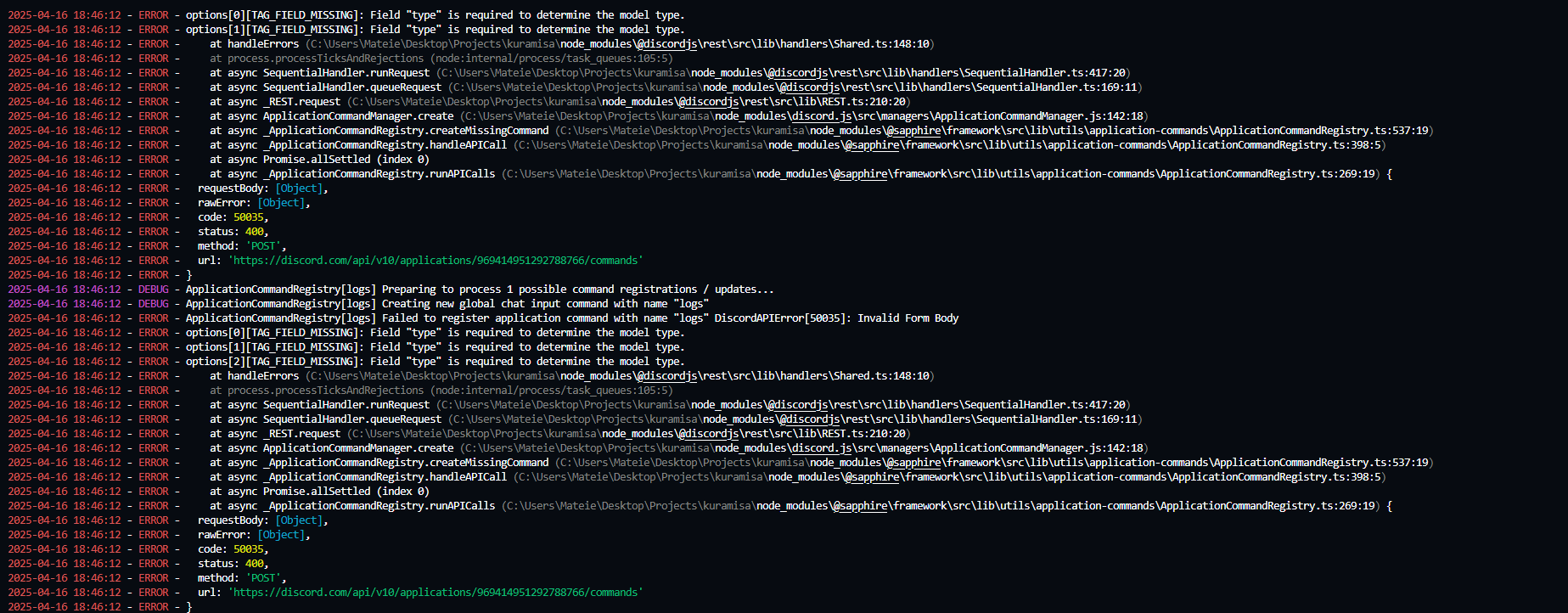
Solution:Jump to solution
This problem is similar to https://discord.com/channels/737141877803057244/1358068581711220797
5 Replies
export abstract class AbstractSlashSubcommand extends Subcommand {
readonly data: SlashCommandBuilder;
readonly contexts: InteractionContextType[];
readonly integrations: ApplicationIntegrationType[];
readonly opts: SlashCommandOption[] = [];
constructor(
content: Subcommand.LoaderContext,
options: Subcommand.Options,
) {
super(content, options);
this.contexts = options.contexts ?? [InteractionContextType.Guild];
this.integrations = options.integrations ?? [
ApplicationIntegrationType.GuildInstall,
];
this.data = new SlashCommandBuilder()
.setName(this.name)
.setDescription(this.description)
.setContexts(this.contexts)
.setIntegrationTypes(this.integrations);
if (options.subcommands && options.opts)
throw new Error(
"You cannot use subcommands and options at the same time.",
);
if (options.opts) {
this.opts = options.opts;
for (const opt of this.opts) {
this.addOption(this.data, opt);
}
}
if (options.subcommands) {
const subcommands = options.subcommands.filter(
(cmd) => cmd.type === "method",
) as SubcommandMappingMethod[];
const groups = options.subcommands.filter(
(cmd) => cmd.type === "group",
);
for (const subcommand of subcommands) {
const subcommandBuilder = new SlashCommandSubcommandBuilder()
.setName(subcommand.name)
.setDescription(subcommand.description);
if (subcommand.opts) {
for (const opt of subcommand.opts) {
this.addOption(subcommandBuilder, opt);
}
}
this.data.addSubcommand(subcommandBuilder);
}
for (const group of groups) {
const groupBuilder = new SlashCommandSubcommandGroupBuilder()
.setName(group.name)
.setDescription(group.description);
for (const subcommand of group.entries) {
const subcommandBuilder =
new SlashCommandSubcommandBuilder()
.setName(subcommand.name)
.setDescription(subcommand.description);
if (subcommand.opts) {
for (const opt of subcommand.opts) {
this.addOption(subcommandBuilder, opt);
}
}
groupBuilder.addSubcommand(subcommandBuilder);
}
this.data.addSubcommandGroup(groupBuilder);
}
}
}
override registerApplicationCommands(registry: Subcommand.Registry) {
registry.registerChatInputCommand(this.data);
}
export abstract class AbstractSlashSubcommand extends Subcommand {
readonly data: SlashCommandBuilder;
readonly contexts: InteractionContextType[];
readonly integrations: ApplicationIntegrationType[];
readonly opts: SlashCommandOption[] = [];
constructor(
content: Subcommand.LoaderContext,
options: Subcommand.Options,
) {
super(content, options);
this.contexts = options.contexts ?? [InteractionContextType.Guild];
this.integrations = options.integrations ?? [
ApplicationIntegrationType.GuildInstall,
];
this.data = new SlashCommandBuilder()
.setName(this.name)
.setDescription(this.description)
.setContexts(this.contexts)
.setIntegrationTypes(this.integrations);
if (options.subcommands && options.opts)
throw new Error(
"You cannot use subcommands and options at the same time.",
);
if (options.opts) {
this.opts = options.opts;
for (const opt of this.opts) {
this.addOption(this.data, opt);
}
}
if (options.subcommands) {
const subcommands = options.subcommands.filter(
(cmd) => cmd.type === "method",
) as SubcommandMappingMethod[];
const groups = options.subcommands.filter(
(cmd) => cmd.type === "group",
);
for (const subcommand of subcommands) {
const subcommandBuilder = new SlashCommandSubcommandBuilder()
.setName(subcommand.name)
.setDescription(subcommand.description);
if (subcommand.opts) {
for (const opt of subcommand.opts) {
this.addOption(subcommandBuilder, opt);
}
}
this.data.addSubcommand(subcommandBuilder);
}
for (const group of groups) {
const groupBuilder = new SlashCommandSubcommandGroupBuilder()
.setName(group.name)
.setDescription(group.description);
for (const subcommand of group.entries) {
const subcommandBuilder =
new SlashCommandSubcommandBuilder()
.setName(subcommand.name)
.setDescription(subcommand.description);
if (subcommand.opts) {
for (const opt of subcommand.opts) {
this.addOption(subcommandBuilder, opt);
}
}
groupBuilder.addSubcommand(subcommandBuilder);
}
this.data.addSubcommandGroup(groupBuilder);
}
}
}
override registerApplicationCommands(registry: Subcommand.Registry) {
registry.registerChatInputCommand(this.data);
}
private addOption(
builder: SlashCommandBuilder | SlashCommandSubcommandBuilder,
option: SlashCommandOption,
) {
switch (option.type) {
case ApplicationCommandOptionType.Boolean:
builder.addBooleanOption(option);
break;
case ApplicationCommandOptionType.Attachment:
builder.addAttachmentOption(option);
break;
case ApplicationCommandOptionType.String:
builder.addStringOption(option);
break;
case ApplicationCommandOptionType.Integer:
builder.addIntegerOption(option);
break;
case ApplicationCommandOptionType.User:
builder.addUserOption(option);
break;
case ApplicationCommandOptionType.Channel:
builder.addChannelOption(option);
break;
case ApplicationCommandOptionType.Role:
builder.addRoleOption(option);
break;
case ApplicationCommandOptionType.Mentionable:
builder.addMentionableOption(option);
break;
case ApplicationCommandOptionType.Number:
builder.addNumberOption(option);
break;
}
}
}
private addOption(
builder: SlashCommandBuilder | SlashCommandSubcommandBuilder,
option: SlashCommandOption,
) {
switch (option.type) {
case ApplicationCommandOptionType.Boolean:
builder.addBooleanOption(option);
break;
case ApplicationCommandOptionType.Attachment:
builder.addAttachmentOption(option);
break;
case ApplicationCommandOptionType.String:
builder.addStringOption(option);
break;
case ApplicationCommandOptionType.Integer:
builder.addIntegerOption(option);
break;
case ApplicationCommandOptionType.User:
builder.addUserOption(option);
break;
case ApplicationCommandOptionType.Channel:
builder.addChannelOption(option);
break;
case ApplicationCommandOptionType.Role:
builder.addRoleOption(option);
break;
case ApplicationCommandOptionType.Mentionable:
builder.addMentionableOption(option);
break;
case ApplicationCommandOptionType.Number:
builder.addNumberOption(option);
break;
}
}
}
registerApplicationCommands
, that fixed it i think
Nvm, im getting "Expected" - "Got" errors nowMakes no sense?
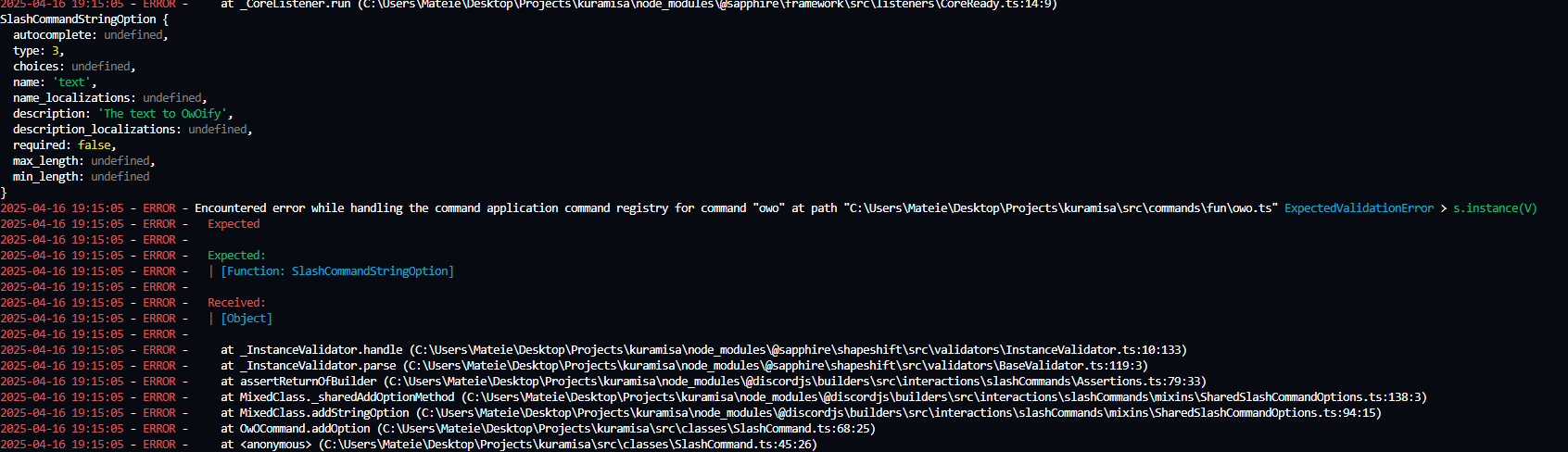
Even thought the Builder is perfect
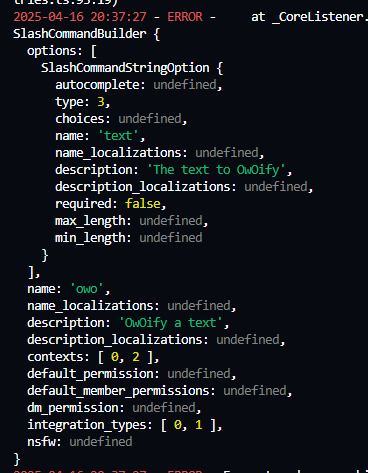
Solution
This problem is similar to https://discord.com/channels/737141877803057244/1358068581711220797
Still cant find a fix