cannot import 'useApollo' composable from another composable
in my nuxt app, i decided to use
@nuxtjs/apollo
as the apollo client because it sets up things for me.
to make usage of it more conveneint, i made a composable useGlobalApollo
which exposes the default client.
then, i use it in my pinia stores to fetch using graphql. here is an example auth store:
then i just use the users store whenever needed during auth. currenlty im using it in a page for login and default layout.
17 Replies
Hi @captivator. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
so my problem is that i get this random 500 error:
the file exists, i checked. i tried importing it manually but it also doesnt resolve.
i can import other composables as usual, but not this one.
i am stuck with this problem for 2 days now.
(i had to split the context behind the problem and the actual post)
----------------------
I see you're having an issue with importing the
useApollo
composable from another composable in your Nuxt application. This is a common challenge when working with composables in Nuxt.
The error you're experiencing is likely related to how Nuxt composables work with the Nuxt context. As explained in the Nuxt documentation, many composables rely on being called in the right context during the component lifecycle:
When you are using the built-in Composition API composables provided by Vue and Nuxt, be aware that many of them rely on being called in the right context.Nuxt documentation The issue in your code is that you're trying to use the
useGlobalApollo
composable (which itself uses useApollo
) at the top level of your store file, outside of any function:
Solution
You should move the composable call inside the store's setup function:
If you're using the options API for your store, you can use the actions
property:
This approach ensures that the composable is called within the proper context during the component lifecycle.
The Nuxt documentation specifically warns about this issue:
You cannot use them outside a Nuxt plugin, Nuxt route middleware or Vue setup function. On top of that, you must use them synchronously.Nuxt documentation If you need to maintain a singleton instance, you might consider using a Nuxt plugin instead, as shown in this Stack Overflow answer: Then you can access it in your composable using
useNuxtApp().$yourApi
.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@739834419598065694> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai
so my problem is that i get this random 500 error:
the file exists, i checked. i tried importing it manually but it also doesnt resolve.
i can import other composables as usual, but not this one.
i am stuck with this problem for 2 days now.
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you're facing an issue with importing the
useApollo
composable from the @nuxtjs/apollo
module. This appears to be related to how Nuxt handles auto-imports and module resolution.
The error you're seeing suggests that Vite is trying to resolve the import using an absolute file path rather than through the module's proper export mechanism. This is not how Nuxt's auto-imports are meant to work.
Possible solutions:
1. Use the auto-imported composable directly
Since you're using @nuxtjs/apollo
, the useApollo
composable should be auto-imported by the module. Try removing the explicit import and just use it directly:
2. Use the #imports alias
If you need to make the import explicit, use the #imports
alias which Nuxt provides for all auto-imports:
As mentioned in the Nuxt documentation:
Nuxt exposes every auto-import with the #imports
alias that can be used to make the import explicit if needed.
Auto-imports documentation
3. Check module configuration
Make sure the Apollo module is properly configured in your nuxt.config.ts
file. The module should be setting up its auto-imports correctly.
4. Check for third-party package auto-imports
If the above solutions don't work, you might need to explicitly configure auto-imports for the Apollo module. You can do this in your nuxt.config.ts
:
As described in the Auto-import from Third-Party Packages documentation.
This issue might be related to how Vite resolves imports or a potential bug in the auto-import system. If none of these solutions work, you might want to check if there are any reported issues with the @nuxtjs/apollo
module or consider using a different approach to access the Apollo client.
Let me know if any of these solutions help resolve your issue!
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@739834419598065694> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
the most puzzling thing about this problem is that its really weird and out of place
it just doesnt want to import the composable
i tried it in a fresh project and it imported everything fine
i reinstalled node_modules freshly and deleted .nuxt, and it didnt help
the file is there but it just doesnt get imported
proof of that
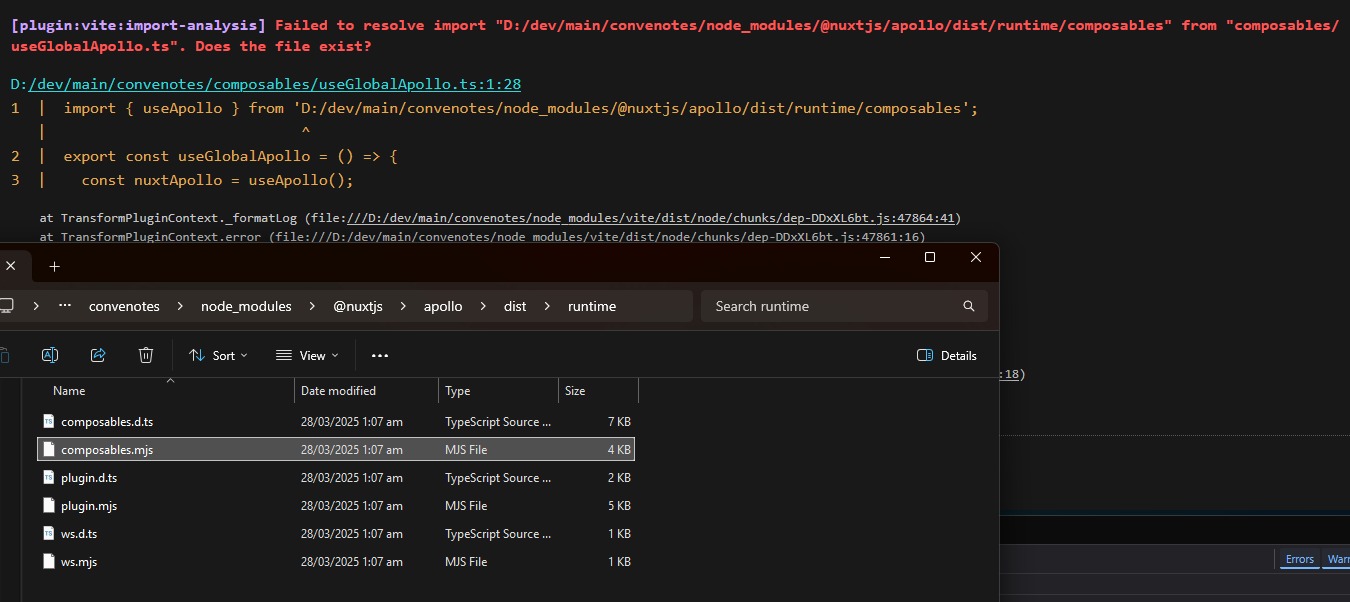
i have decided to make a post here because i genuinelly have no idea how to solve this
if i dont fix this them im just going to write my own wrapper over apollo client instead of relying on alpha versions of nuxt modules or vue packages
@kapa.ai so the "composables.mjs" and "composables.d.ts" exist in the folder, but vite keeps on saying me that "maybe the file doesnt exist"?
it just doesnt want to import useApollo from the library, even though its THERE and it finds the path.
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand your frustration with this import issue. This appears to be related to how Vite resolves modules in Nuxt, particularly with dynamically imported modules.
Based on the information in the knowledge sources, there are a few potential causes and solutions for this issue:
Possible causes:
1. Chunk loading errors: This could be similar to the issue described in this GitHub issue where Nuxt fails to resolve file paths correctly.
2. Vite import conditions: There was a fix in Nuxt related to Vite import conditions as mentioned in this PR, which resolved issues with import resolution.
3. Path aliases: The error shows an absolute path being used instead of module resolution, which suggests an issue with how the module is being imported.
Solutions to try:
1. Use auto-imports instead of direct path
Instead of importing directly from the module path, rely on Nuxt's auto-import system:
2. Configure explicit imports in nuxt.config.ts
You can explicitly tell Nuxt to import from the Apollo module:
As shown in the Auto-import from Third-Party Packages documentation.
3. Try transpiling the module
If the module is having issues with ESM compatibility:
4. Check for Nuxt/Apollo version compatibility
This could be related to a compatibility issue between Nuxt 3.16.0 and the Apollo module, similar to what was reported with Algolia in this GitHub issue.
5. Use the #imports alias
If none of these solutions work, it might be worth checking if there's a specific issue with the version of
@nuxtjs/apollo
you're using, or if there are any reported issues in their GitHub repository.
The fact that the file exists but Vite can't resolve it suggests this is more of a module resolution or bundling issue rather than a missing file problem.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@739834419598065694> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
oh
oh...
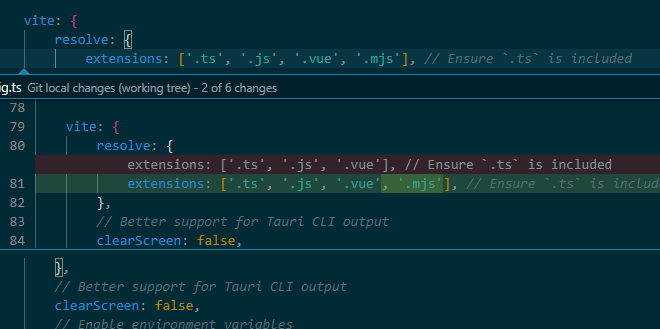
oh
of course
OF COURSE
@kapa.ai so the answer was simple: i just didnt include .mjs in vite.resolve
i am so angry rn
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand your frustration! This is indeed a common issue with Vite and ESM modules in Nuxt. The
.mjs
extension is crucial for proper ESM resolution.
This relates directly to how Nuxt and Vite handle ES Modules. As mentioned in the ES Modules documentation, sometimes you need to manually alias libraries to their correct file paths with extensions.
For your case, you needed to specify the .mjs
extension when importing from the module. This is a common pattern when working with ES Modules in Node.js environments.
You could have resolved this by adding an alias in your nuxt.config.ts
:
Or by directly importing with the correct extension:
These small details with file extensions can be incredibly frustrating to debug, especially when the error message isn't entirely clear about what's wrong. At least now you know for future reference!
I'm glad you found the solution, even if it was a seemingly simple fix after two days of troubleshooting.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@739834419598065694> Kapa.ai is still learning and improving, please let me know how I did by reacting below.