In String constructor `String(Char*)`, `Char*` is character pointer or character array pointer?
I was exploring
System.String
class here: https://learn.microsoft.com/en-us/dotnet/api/system.string?view=net-9.0
And one of the constructors is String(Char*)
Its description says: Initializes a new instance of the String class to the value indicated by a specified pointer to an array of Unicode characters.
However, I got error, when I tried following code to initialize string using pointer to an array of characters:
So, my question is:
1. Is String(Char*)
takes character pointer as argument instead of character array pointer?
2. Or am I doing some mistake in declaring and initializing character array pointer?String Class (System)
Represents text as a sequence of UTF-16 code units.
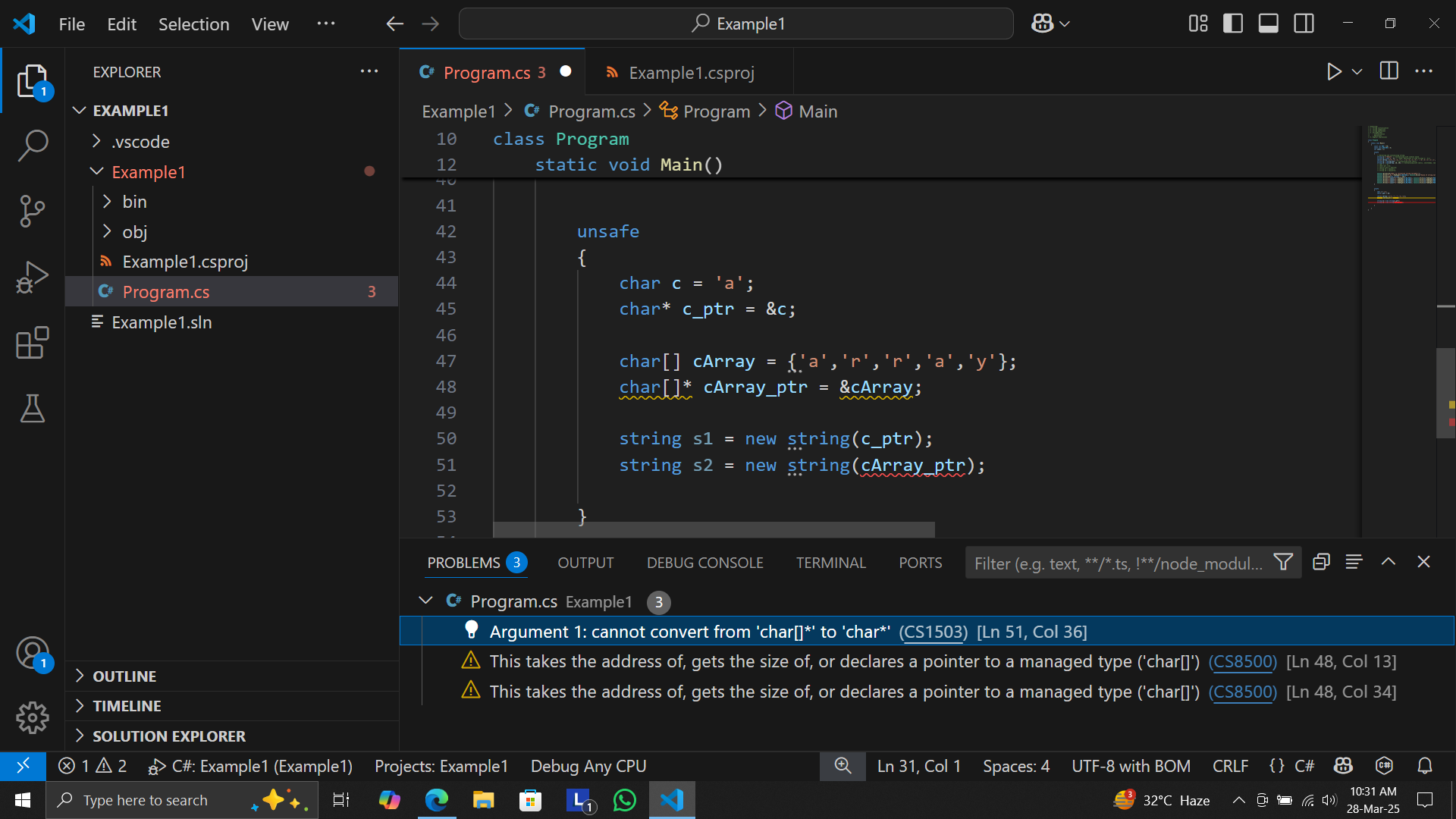
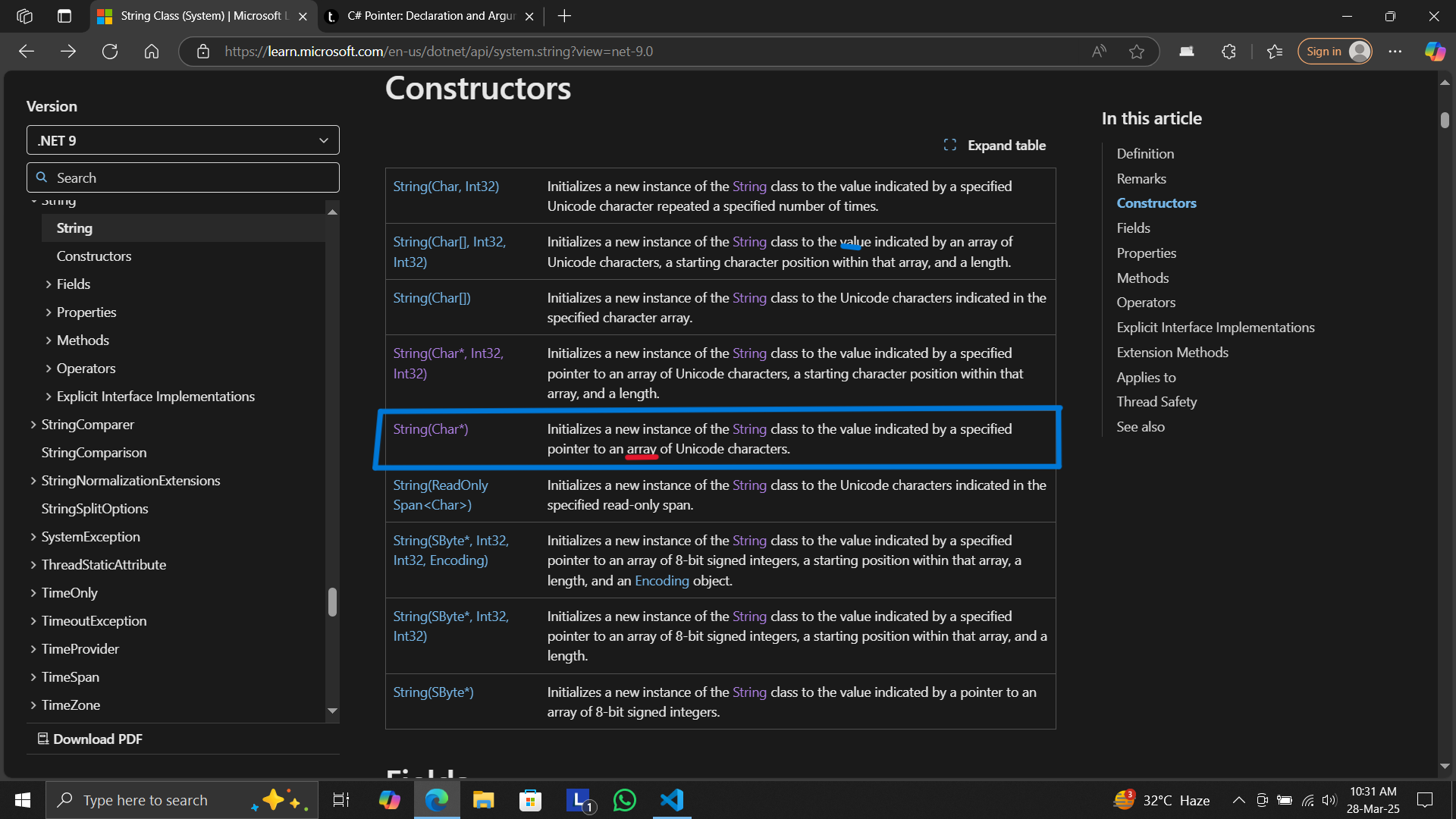
8 Replies
it's a pointer to an array in a C kind of sense
the "Pointers and Arrays" section of The C Programming Language would be a good read if you can get a copy of it
anything you can do with an array can be done with a pointer, so a
char*
is a char[]
, in a way. in other ways it's notin terms of the number of indirections, a
char[]*
is the same as char**
, not char*
what you want is to get the char*
of a char[]
. you need to use the fixed
statement for thisfixed statement - pin a moveable variable - C# reference
Use the C#
fixed
statement to pin a moveable variable and declare a pointer to that variable. The address of a pinned variable doesn't change during execution of the statement.It's always interesting to me how people take a language that lets you avoid all that pointer tomfuckery, and decide to use pointer tomfuckery anyway :KEKW:
Ok, so
Char*
in String(Char*)
is pointer of first element of character array. If I understand right.
Hence, both way declaring and initializing pointer works
Thanks, the link was very helpful 🙂right. well, it's a pointer to whichever element you want to be the first
you can give a pointer to
&cArray[2]
and it will create a string starting from there
but you have to be careful because this is not a valid usage of new string(char*)
the way it calculates the length of the string is by searching for the first character which is '\0'
in the array your pointer is pointing to, and stopping there
you do not have a null character in your array. so your code only works because the memory after the array happens to be empty. it may not always be the case
you should include a '\0'
in your array. or, you can use new string(char*, int, int)
to provide a start offset and length, so it does not have to search for the '\0'
👍
also, like, never do this
perfectly fine to play around and explore but
unsafe
is for when you're experienced enough to know how to break the rules the right way in the name of performance