Is there a better way to write this?
import { type } from "arktype";
export const nonEmptyStringOrNull = type("string | null | undefined").pipe(s => !s ? null : s);
84 Replies
And for using it is there a better alternative than this everywhere?
This looks good in terms of the implementation. There is an issue tracked here that could add a config option for how values like empty string are treated in terms of defaultability:
https://github.com/arktypeio/arktype/issues/1283
GitHub
Allow null to be treated as not-present for optional fields · Issue...
Request a feature (Related to #1191) I wish to be able to have a type with an optional non-null field that accepts null as being equivalent to not present: import { scope } from "arktype"...
You can use a generic if you want to apply that union + transformation to an arbitrary string input like
string.email
in a reusable way:
https://arktype.io/docs/genericsArkType Docs
Optimized runtime validation for TypeScript syntax
@ssalbdivad thank you David! I decided to use Arktype for my work project so my fate is tied to it lol. Two questions, how can I make a key in my type optional but if you don't include it it falls back to the value null? Can I do that in a generic way?
When you take a look at OpenAPI stuff can you please make sure it works with Hono OpenAPI? Thanks!
https://github.com/rhinobase/hono-openapi
GitHub
GitHub - rhinobase/hono-openapi: A plugin for Hono to generate Open...
A plugin for Hono to generate OpenAPI Swagger documentation - rhinobase/hono-openapi
Sounds like a normal defaultable property
https://arktype.io/docs/objects#properties-defaultable
ArkType Docs
Optimized runtime validation for TypeScript syntax
I couldn't figure out the syntax, could you show by making this defaultable to null?
Pretending I had done "email?"
Hmmmmm LOL Ill try it when I'm next at my computer but I could have sworn default was not a property on to()
I mean I guess the whole
.to()
thing doesn't really makes sense TBH I just copied that haha but .default
should be on every type
Or I'll rephrase that- it does make sense it just not the cleanest way to do it, and is especially confusing because nonEmptyStringOrNull
sounds like a validator but actually also transforms
With a generic you should just be able to validate string.email
in place of where string
was in the original typeI'm brand new to Arktype, could I see how you would make that type? Also is there a playground link you use to play around with Arktype?
No playground for 2.0 yet unfortunately. Here's one way to do it:
I'm not at my computer right now, does the default imply the type of email would now be "email?" ? Thank you for the help!
Not sure exactly what you mean but since TS does not have an email type it would still be inferred as
string
, it would just be enforced as emailI mean if I pass in an empty object would that type assert successfully and give me back an object with an email field of null
I tried to try it on codesandbox but it's hell to use that site on a mobile device lol
Yeah
Tried it out in Valtown and it worked! Have a great day!
Sweet! Hope the adoption continues to go well 😊
My only real complaint right now is wow LLMs are terrible at Arktype, but I think I saw there was a GitHub issue for that
Yeah will be sure to add llms.txt which hopefully will help. Plus as newer model released obviously will just improve with more training data
The more it has context on your own valid definitions though the better it should do
Im so sorry for more questions I am struggling to figure out the syntax for combining multiple keyword validations into a generic
Compare to something like:
What you want there is body of the generic. it should reference
s
(whatever string you pass in) and then apply more constraints to it. In my original case, I applied a morph to it with the morph tuple expression =>
How would I add a string.trim to this?
You could do something like this but at this point it may be easier to just create your own external generic function (there's an example of this in the generic section of the docs) that creates a type:
Actually I guess you probably want the string constraint (e.g. email) to apply after it is trimmed, so you could do:
A lot of string subtypes would already be guaranteed non-empty anyways though
When I pass in a string literal to
stringParserOrOptional
it its typed as string instead of the literals https://discord.com/channels/957797212103016458/1350886854844612678/1350886854844612678This looks like a limitation of how TS handles generic inference in a case like this.
Probably best to just write the generic manually like this:
😬 THANK YOU BUT WOW I WOULD NEVER HAVE GOTTEN THAT LOL
Oh wait sorry, I meant I was having trouble with this function
Writing TS generics and especially integrating them with library types gets hard fast haha. That's why we try to get as many APIs to work out of the box as possible without wrapping.
This kind of pattern can be reused for other cases though
Maybe a good exercise then to see if you can adapt what I wrote for that case 😛
Im using Valtown as a playground, do you have a prefered alternative?
ArkType Docs
TypeScript's 1:1 validator, optimized from editor to runtime
It's not finalized/announced yet but the types seem to be working
Really sorry to bother you but I think syntax might be wrong, in the playground its not showing a type if you hover over timeToNotEmptyEmail, and on valtown its interpreting it as JSX https://www.val.town/v/arjunyel/arkTypeTest
Haha seems like a JSX parsing bug... the syntax is definitely right I wrote it in my own editor!
As soon as arktype llm.txt I promise I wont be bothering you 😄
There are some cases like this where I've seen .jsx files fail to parse because they get confused about generics
You can probably fix it by changing
const def
to const def extends unknown
Haha unfortunately issues like these that have more to do with ts generics in general I don't think it will help much withconst def extends unknown works if you run it but typescript still cant read it https://www.val.town/v/arjunyel/arkTypeTest
Maybe ping val.town about their parser?
Definitely works in pure ts
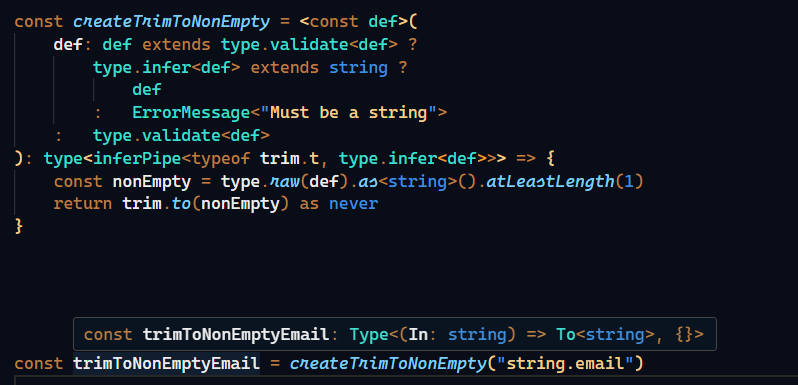
Im trying to get closer but its saying 'trim' only refers to a type, but is being used as a value here.
Trim should be this:
const trim = type.keywords.string.trim.root
Thank you so much I think Im almost there!!!!!
const test: string | (Type<null, Ark> & string)
but im expecting string | null
I would just change the return to
Assuming you always want to accept a
string
initially, you can also remove a lot of the null | undefined
logic and just add that to the piped union at the beginning e.g. :
Im still super struggling
Can I pay you for help or something?
This should output
{ email: null }
This works perfect, just doesnt keep string literals in the output type
What exactly are you trying to achieve here?
A value which is a string, or if not specified defaults to
null
?This but it also works with string literals
Last 3 lines of this is a test case
Where does
type.keywords.string.trim.root
come into it?That was extra but basically it would be nice if the string was trimmed before we check it it's empty
Right
Well this doesn't do "
test.from({})
should output { email: null }
"
This still requires whatever key to have an explicit valueIt works when I ran it, did it not work for you?
So do you want the key to be required, or should it default to
null
?Key is not required, if it's missing it's set to value null
this errors (both types and runtime)
So "if input's value is an empty string, or null/undefined, or not provided, the output's value should be null. Otherwise use the provided value as the output"?
Hmmmmm I swear it was working.... Not sure what happened.. maybe I'm imagining things.
Basically I pay you if I can get some function that supports strings and literals, if the key is missing or empty or empty after trimming I want the value set to null
Correct, it would be nice if it handled the Arktype string types like email
I mean this sounds simple enough.... Give me a minute...
And I'm assuming you also need the generic inference so the type is
s
rather than string
?
Where s
comes from the validator definition and not the usageSorry friend I am new to Arktype, the generic part was so I could pass in string or string subtypes like email or uuid
Right, so in theory you just want
email: nonEmptyStringOrNull
, where nonEmptyStringOrNull
does the validation I mentioned above (you don't strictly need the ("string")
part of it)?But how would I do nonemptystringornull("string.uuid") for example? I think that's why David recommended a generic
Oh wait I see. You might want
("string.email")
or ("string.uuid")
Yeah
Okay
I think I understand
If we do nonEmptyStringOrNull("string.email")
, and the user provides "foo"
(i.e. an invalid email), should the result be an error or null
? @SchmimeError
Okay, I think I've got it
does this seem good for test cases? @Schmime
That looks amazing
I'm just figuring out the correct things for the types then will send
👀👀👀👀
There's also this when you do
nonEmptyStringOrNull("number")

Hopefully this is good for you :)
Oof, discord syntax highlighting doesn't like that lmao
Looks like the
type.raw
is needed actually (have edited it back in) 🙈Module '"arktype"' has no exported member 'Out'.
Ah, I guess my PR adds that export 😅 https://github.com/arktypeio/arktype/pull/1159
GitHub
feat: support JSON Schema as input for a Type by TizzySaurus · Pul...
Closes #729.
What
This PR adds @ark/jsonschema, which is a library enabling users to convert a valid JSON Schema schema into an ArkType type.
API
Below is a basic sample-use of @ark/jsonschema:
imp...
Let me find the code for it...
@Schmime Add this to your file instead of the
type Out
import. It hopefully won't be necessary in ArkType 2.2+ (the next release), but it is for nowTHANK YOU SO MUCH!!!! I think we are super close, last test case should be r2 should return { email: null }, right now its throwing an error
const test = type({ email: nonEmptyStringOrNull("string") });
const r1 = test.from({});
const r2 = test.from({ email: "" });
const r3 = test.from({ email: " [email protected] " });
console.log(r1); // {email: null}
console.log(r2); // TraversalError: email must be an email address (was "hi")
console.log(r3); // {email: "[email protected]"}
You said here r2 should error 😅
I guess because it was non-empty
Okay... that's easy enough... one sec
Sorry I changed "string.email" to just "string" as a test
Hmmm
So should
""
be null, and "a"
be an error for string.email
?Yes exactly
Example usecase is in a frontned form if a feild is empty itll send an empty string, but id want to store as null in database
Okay... I mean honestly the easiest thing is just explicitly check for
""
Check has to be after trim
So this is soooo close, just I need to do the === "" check after the trim is applied
Yeah, it's easy enough to do
I'm just seeing the best way
I have to leave my computer to go, I appreciate your help so much, can you DM me the best way to pay you?
I think this is what you want
Hmm I guess maybe not... let me think
Ill test whatever you come up when I get home. Btw I insist on paying you lol
This should be good :)
AHHHHHH I THINK ITS ALL WORKING
YAY THANK YOU SO MUCH I SPENT SO MUCH TIME ON THIS LOL
@ssalbdivad GIVE @TizzySaurus A RAISE PLEASE
Lmaaooo
All my work here is volunteer-based :)
Honestly it's just great to help people out
Naw Im paying you, I insist
Let's go DMs :)