customizing error messages
Hi, I'm just trying this library and so far looks very promising. I cannot, however, find anywhere if it is possible to have different error messages for different validators (for a single field)? For example in Zod I could do sth like this:
z.array().min(2, 'choose at least 2').max(3, 'choose no more than 3')
Is there an equivalent in ArkType?...Arktype pros and cons
Hey, we all know how great arktype is, currently I'm about to convince my CTO to use arktype for new projects, is there any indefeasible points for Arktype to win the debate apart from what already found on the web? 😂
Partial parse
Once in a while we encounter some data that's been stored away and the schema has changed over time. I'd like to parse it but also be forgiving enough to return the partially parsed schema. Something like this:
```typescript
import { type } from 'arktype';
...
functions that take in types
I have certain patterns i would like to make simpler like default arrrays or unique checks but I can't seem to figure out how to type them
this is an example I would like to streamline
```ts...
Type script Error
Can anyone please help on how to fix this type script error below 👇 ??
Type 'Tokens' is missing the following properties from type '{ chainId: any; contract_decimals: number; contract_name: string; contract_ticker_symbol: string; contract_address: string; supports_erc: ["erc20"]; logo_url: string; last_transferred_at: string; ... 8 more ...; nft_data: null; }': contract_decimals, contract_name, contract_ticker_symbol, contract_address, and 11 more.ts(2345)...
Loose string schema
Does arktype have loose string support? though the schema can accept any string, the infered type can be something like
...
type Thing = "literal" | "or" | "any" | (string & {})
type Thing = "literal" | "or" | "any" | (string & {})
zod .refine() equivalent? min/max validation
Hello!
I'm currently exploring arktype to replace zod and was wondering if it was possible to replicate the functionality that zod provides via
.refine()
in arktype.
eg, performing a validation using multiple fields that relate to one another in an object. in this case, letting a user set a custom min/max range in two different inputs:...string.integer.parse < 100
Here's an actual validator I'm trying to build
...
const decimals = type('string.integer.parse | number.integer < 28')
const decimals = type('string.integer.parse | number.integer < 28')
Key validation on arbitrary number of properties.
I'm working on validating an object where:
- Some property keys are known and expected.
- Other extra/unknown keys are allowed only if they follow a specific pattern.
...
spread and generics
I'm wanting to define a generic, then another generic wrapping and passing through the generic
```ts
const apiResponse = type('<t>', {
data: 't',...
How can I validate a `Set` of certain item type?
Hello,
I am trying to create a schema that validates that a property is a set of strings:
```typescript
const schema = type({...
Translations /i18n
I’ve seen that it’s possible to edit the messages directly on each “type” but is there an easy way of seeing that you’ve edited every possible error message? And is there some way to edit it “globally” for every instance of “type”?
Zod Interop?
I'm seeing a lot of MCP tools exposing Zod-based APIs, which is annoying. I'm sure this has been discussed before, but is there a way to use an Arktype schema in place of Zod?
Here's an example from https://github.com/punkpeye/fastmcp where Zod is used but I'd like to use Arktype:
```typescript...
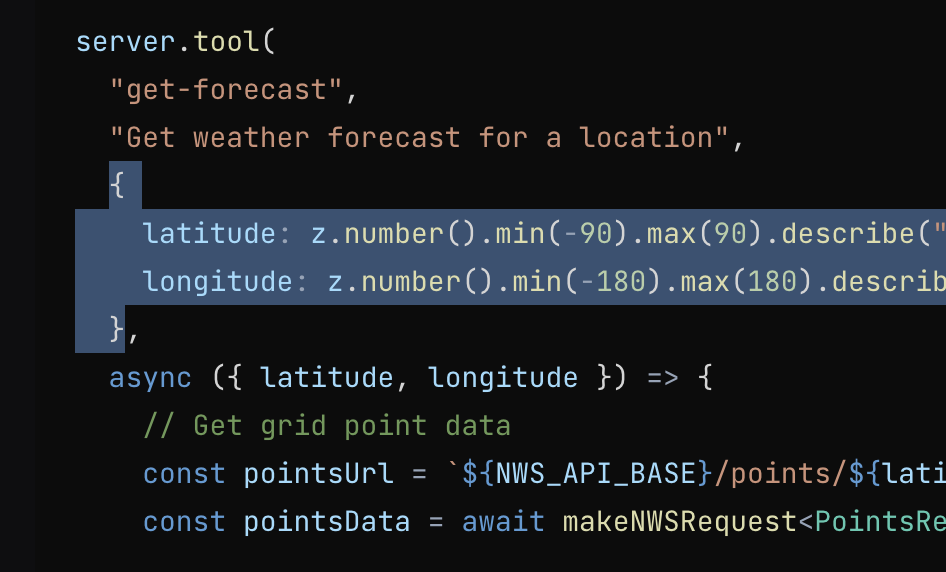
How to use reject correctly?
Hi I want to validate input file in a form. It can be either a single file or an array of files, if it is a single file then validate its maximum size, if it is an array of files then validate their total size.
I want a custom message, actual.
With this code I get an exception.
```ts
"files[]?": type("File | File[] < 6").narrow((data, ctx) => {...
Issue with Default<> in Type as generic.
I'm trying to create a generic function that accepts type returns other type.
```typescript
export const generic = <t = unknown, $ = {}>(filter: Type<t, $>) =>
type({...
Type instantiation is excessively deep and possibly infinitely deep
Hello,
I have some TRPC endpoint definition which contain some complex type
```ts...
Trying to index an array type with .get(...path)
const schema = type('string[]');
console.log(schema.get(0).expression);
const schema = type('string[]');
console.log(schema.get(0).expression);
string[][0]
equals string
....Reference another schema type as default?
Hello, I'm relatively new to Arktype. I've managed to figure out most of our Zod migration, however I have a couple scenarios where I would like to reference other types as default values -or- compose a 'computed' property.
In this scenario I'd like to use the provided 'createdAt' value as the default 'modifiedAt' value:
```typescript
import { type } from 'arktype';...
string.numeric.parse accepts empty strings and returns a NaN
console.log(type("string.numeric.parse")(""));
console.log(type("string.numeric.parse")(""));
NaN
. Is that the expected behavior? What is a nice way to achieve the behavior I'm looking for?...