ArkType `toJsonSchema` returning not open-api compatible schema.
Hi, I am trying to get my arktype schemas working with fastify (was previously using zod but editor performance was tragic) so I started with setting OpenAPI.
Zod tools use https://www.npmjs.com/package/zod-to-json-schema for this but since ArkType has a method built in I decided to use it the output however is different and zod's one actually uses enums/unions in a way that openapi can handle.
18 Replies
This is the generated JSON for both.
You can see that zod has
and ArkType has anyOf
I guess this is naive but what is the difference?
The difference is the interpretation of such a schema by open api generators
I actually didn't know this syntax existed, but it's actually closer to ArkType's internal Json representation
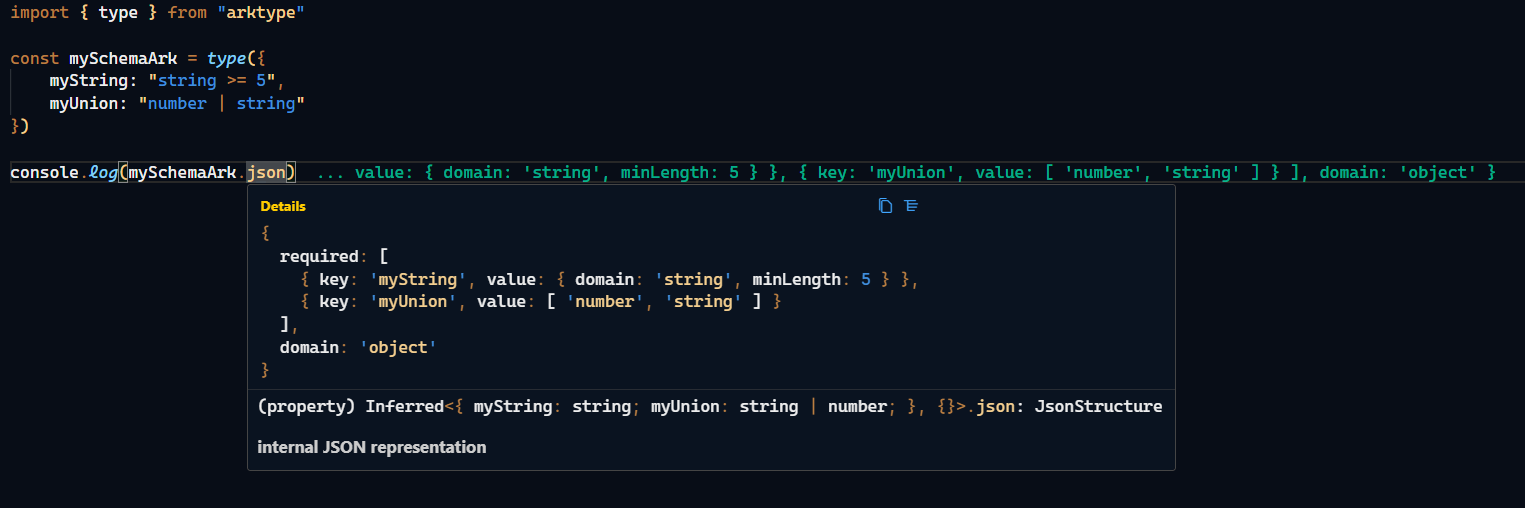
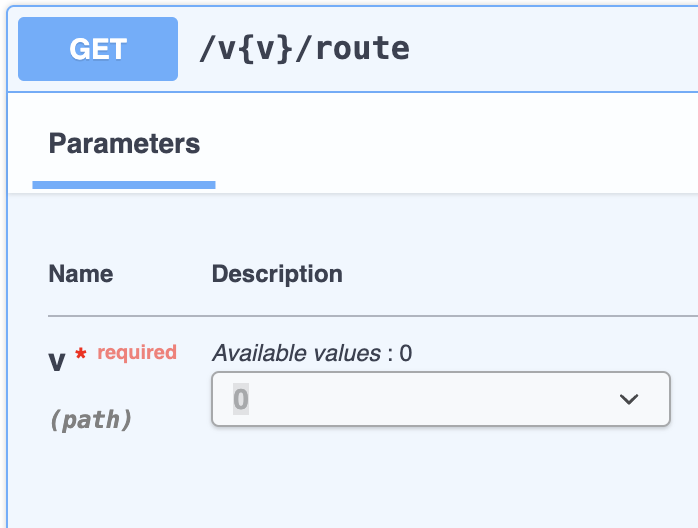
then u can see that value is not an enum
it's just 0
where with zod its correct 0 or 1
I am planning some JSON schema work in 2.1.0. If you want to create an issue I can simplify these cases where possible
My only goal in releasing to
toJsonSchema()
was to generate valid JSON schema. I didn't look at OpenAPI or anything similar, which I guess accepts a subset of JSON schema? Happy to see where I can align with thathttps://github.com/fastify/fastify-swagger
I am using this internally
(not that this issue might not be related to arktype I just noticed the difference when I was migrating and it's a pretty big deal)
GitHub
GitHub - fastify/fastify-swagger: Swagger documentation generator f...
Swagger documentation generator for Fastify. Contribute to fastify/fastify-swagger development by creating an account on GitHub.
That makes sense because I haven't looked at OpenAPI directly at all
and there is a transform
But I know it would be valuable to a lot of users so next release seems like the right time if you want to create an issue!
Just be sure to include the cases that currently are generated in a non-compatible format and could be generated in a comptable one
sure thanks for help
It would be useful to look at this zod to json schema as it looks battle tested and make the outputs similar so the tools like open api work properly
I mean I don't think the issue is JSON schema AFAIK the issue is OpenAPI compatability (or maybe something to do with Fastify not 100%).
So I'd probably just want to look at whatever that spec was and make sure what we're generating conforms to it
@stanisław Are you able to create a GH issue for this summarizing the cases you expect to work? Otherwise I will try to create one but would be useful to get it from someone using this tooling
I will do it @ArkDavid if not today then on the weekend
GitHub
Make toJsonSchema method compatible with OpenAPI spec / @fastify/sw...
Request a feature Make output of toJsonSchema method on enums/unions compatible with OpenAPI spec and make the output look the same to other popular tools like zod-to-json-schema for zod. 🤷 Motivat...
Thank you!