✅ Solved! :D
Heya! I was getting help yesterday and I feel like it's almost fixed, but the calculate should display the sum of everything in the array but it keeps giving the wrong number :/
Any help would be greatly appreciated!
Here is the old thread if you'd like context of where we left off:
https://discord.com/channels/143867839282020352/1341809906256449597
32 Replies
Here is the code:
I believe this is the problem area specifically:
You're not really using the rows you're iterating over
What do you mean?
You never use the
r
inside of the loop
So your loop does "for each row in the grid, do nothing with that row"dataGridView1.Items was not a valid thing, so I tried to do it a different way
ah
Presumably, you want to get your values from that row
The values should be stored in the array
that's what I made it for
inputCostArray
only ever contains a single value, thoughAll I'm trying to do is get the sum of the array and display it
mmm
then why iterate the data grids rows?
that was not the intent
Was a suggestion made yesterday
Perhaps with each save button click you want to add an item to that array?
yes?
It would be best to use a list then, not an array
You would have a
List<decimal> inputCosts
field, then you would inputCosts.Add(value)
new values to it
Then you'd loop over it and sum it up like you're doing now
Without the datagrid rows, that is:PepeS:
Okay I'll try
Under the save button?
calculateBtn_Click
does the calculations right now
So, presumably, hereoh man
I have to undo like everything?
Also, would (value) be substituted for inputCosts?
No, you wouldn't need to undo much
And yes
value
would be whatever you want to addAngius
REPL Result: Success
Result: List<int>
Compile: 282.535ms | Execution: 28.624ms | React with ❌ to remove this embed.
List<decimal> inputCost = new List<decimal>(inputCost);
inputCosts.Add(inputCost);
Like that?
oh wait
List<decimal> inputCost = new List<decimal>(inputCost);
List.Add(inputCost);
?
No
List<decimal> inputCost = new List<decimal>();
would be the field
You would use inputCost.Add(...)
in the save button code
And you would loop overt inputCost
to calculate the sum in the calculate button code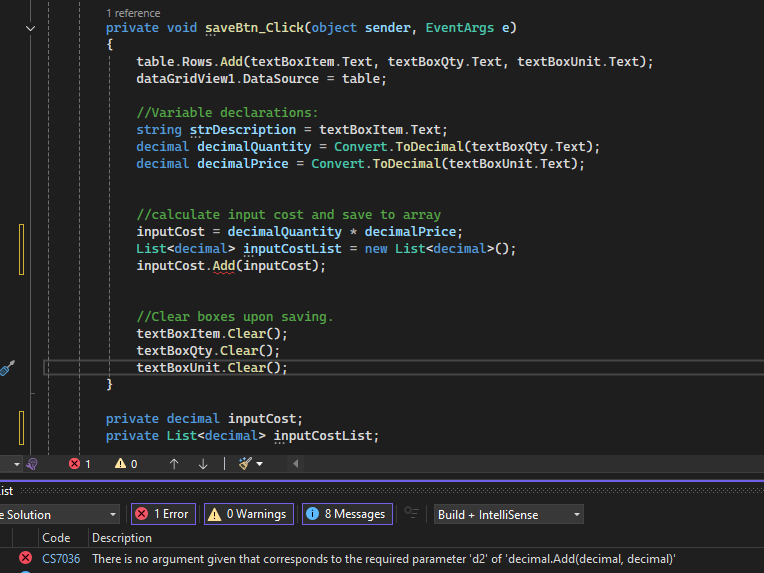
Which part of "would be the field" did you miss?
We went over fields and scopes yesterday
oh, I misunderstood sorry
Also, note that you're trying to
.Add()
to your existing field that's an array
Arrays don't have .Add()
It worked! :D
Thank you so much!!!!
:Ok:
It fully works now!!!
You have no idea how much you've helped me, this was supposed to be a 4 person project and none of my partners showed up
Thank you so much for real
Make sure to sign the project with your name and your name only lol
Yeah, 100% I already told my teacher, and the only consolation was that they would not get any points. Still had to do it all alone in the same timeframe so I've been STRESSED
Nice, a decent teacher it seems
$close
If you have no further questions, please use /close to mark the forum thread as answered