✅ Extreme Newbie - Can't Call Class Element Properly.
Hello! I'll start off by kindly asking you don't laugh at my lack of knowledge. I am doing a 4 person group project alone because my teacher is a horrible person and I need help badly.
I am trying to store variables in an array, and then allow the user to calculate the total cost of the stored variables by pressing that calculate button. I feel like I've gotten it all, I just can't get the button to call the sum properly and display it in the table. Any help at all would be appreciated more than you could possible imagine~
I'll post my full code below, as well as an image of what it currently outputs
80 Replies
Code:
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/Not sure why you have that
Globals
class... inside of another classWhat happens:

Complete transparency, I've been working on this for like 10 hours, and I've been taking a lot of suggestions from google
Well, then, your
DisplayAndSum()
method does, effectively, nothingI feel very out of my depth here, but I have no one to really ask for help, Like I said my teacher isn't very... helpful
It takes an array of decimals, sums them, and... does nothing with the sum
ah
var totalSum = new Globals();
table.Rows.Add (totalSum);
That's what I was trying to do here, but clearly I am not knowledgable enough to make it happen lmfao
I am trying to display it to a new row basically
I was trying to get the button to call the sum itself, but I was running into so many issues, this is the only way I could get it to work, so I thought I did it, and then it put this out lol
Is there a way to properly call it that I'm missing? Or am I just doing it way wrong?
Well, you will have to get the array into this method
Easiest way to have any data available to multiple methods is using a private field
For example,
I think that's what I was trying to accomplish but I wasn't able to pull the information out of the save area without an error
Bar
here would be saveBtn_Click
that creates the data, and Baz
would be calculateBtn_Click
that uses itThat's why all the calculation stuff is there
mmm okay, I think I understand
So, Like this?
Or would I declare the variables outside calculate?
Where are you getting
decimalQuantity
and decimalPrice
from?
They're not local variables, not parameters, not fields
The text boxes
They're local variables to
saveBtn_Click
//Variable declarations:
string strDescription = textBoxItem.Text;
decimal decimalQuantity = Convert.ToDecimal(textBoxQty.Text);
decimal decimalPrice = Convert.ToDecimal(textBoxUnit.Text);
They only exist within the
{}
of saveBtn_Click
mmm
how do I fix that?
That's what fields help with
I think that's probably the root of my issue
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
Braces create scopes. Things are only available in their scope and all child scopes
right
My confusion is how I would acomplish this
Savebtn {
inputs;
CalcBtn {
doing things w those inputs;
}
}
Whenever I try to edit the set voids it breaks everything and I basically have to start over
unless it's way more simple than I think and I'm just dumb which is possible lol
Because from what I understand you can't nest voids into eachother
Because they have to enter the information by pressing save
Wym "voids"?
private void calculateBtn_Click(object sender, EventArgs e)
That's a method
Sorry
$structure
I am starting to get lost I think. They are defined as methods by the program, I can not change that or it breaks everything
Never told you to change the methods
Introduce a field
To make data available in any method in the class
Presumably, you would like to have those two values in your
calculateBtn_Click
method, right?
Yeah
Turn them into fields
Local variables are that: local
Available only ever inside of the method they're in
Not in any other method
Fields are available in any method in the class
So you can give them values inside of
saveBtn_Click
, and then use those values in calculateBtn_Click
Okay, so
Like that?
No, not whatsoever
oh :keksob:
sorry
Your code is currently equivalent to
a
and b
are not available in calculateBtn_Click
Right
Now they are
oh
Okay,
So, like that?
Closer
You still declare local variables instead of assigning to the fields
Look at my examples and note the differences
How
int a = 3;
turned into a = 3;
I don't think I understand. Are you saying that's too specific or too broad?
oh wait
I think I see what you meant
instead of marking it as a decimal in the method, do it outside
Yep
:D
I got it to kind of work!!!
It just displays 0 now though
decimal sum = 0;
foreach (var element in inputCostArray)
{
sum += element;
}
table.Rows.Add (sum);
This would add together all the items in the array right?
I am also getting this error, so I'm wondering if I didn't do this right
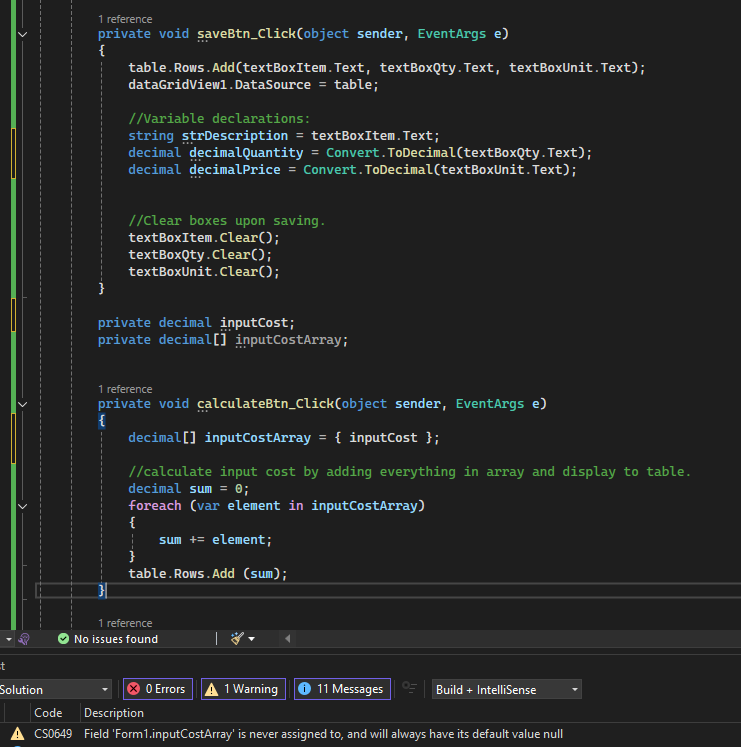
I think I'm moving in the right direction? I am having an issue here though
decimal[] inputCostArray = inputCost;
it says it can't convert decimal to decimal[]
Means you never give this field any value
Yeah, I'm trying to now, I'm just struggling
Correct,
inputCostArray
is an array, stores multiple decimals
inputCost
is a single decimalHow do I add the input to the array?
Here's how you used to have this code, and it was correct

That was giving an error though
Ah, well, might have to create the array explicitly then
new decimal[] { inputCost }
Or maybe [ inputCost ]
Depending on the version of C#/.NET used, the latter is probably the better optionI can try that
Nope :/
C# 7.3
says I'd have to use version 12 or higher for the latter
And then the first give this error
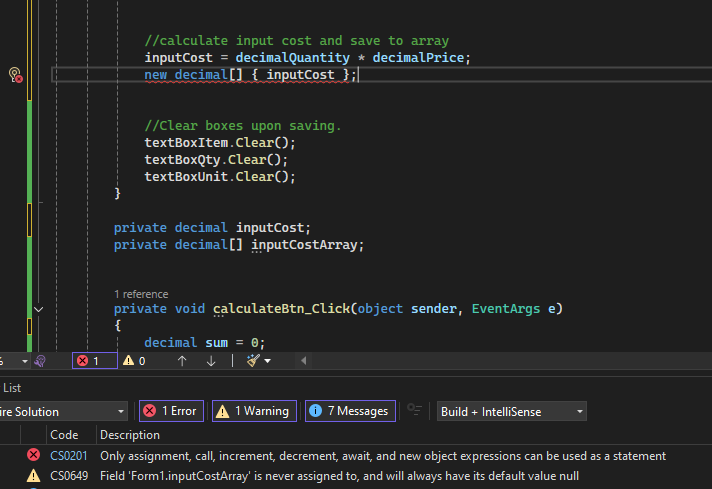
inputCostArray = new decimal[] { inputCost }
We're currently on C# 13, btwIdk how to upgrade, my teacher sent out a specific version for us to use
But duly noted for once the class is over!
Yeah, if the teacher requires this one, use this one
I'd like to learn more of this on my own with no strict timeframe
Chances are they wouldn't know the new features and mark them as errors lmao
It looks like that worked, but still says will be null
Honestly, 100%
Ah, it warns about
null
because, before the value gets assigned to this array, that's what it's going to be
null
, nothing
Accessing elements of this array would be an error
An easy way to handle it is just give the field a default value, empty array
should work
If not,
What is an initializer?
In what context?

Ah, duh
Try
Fixed the error, but still has the warning
Show it
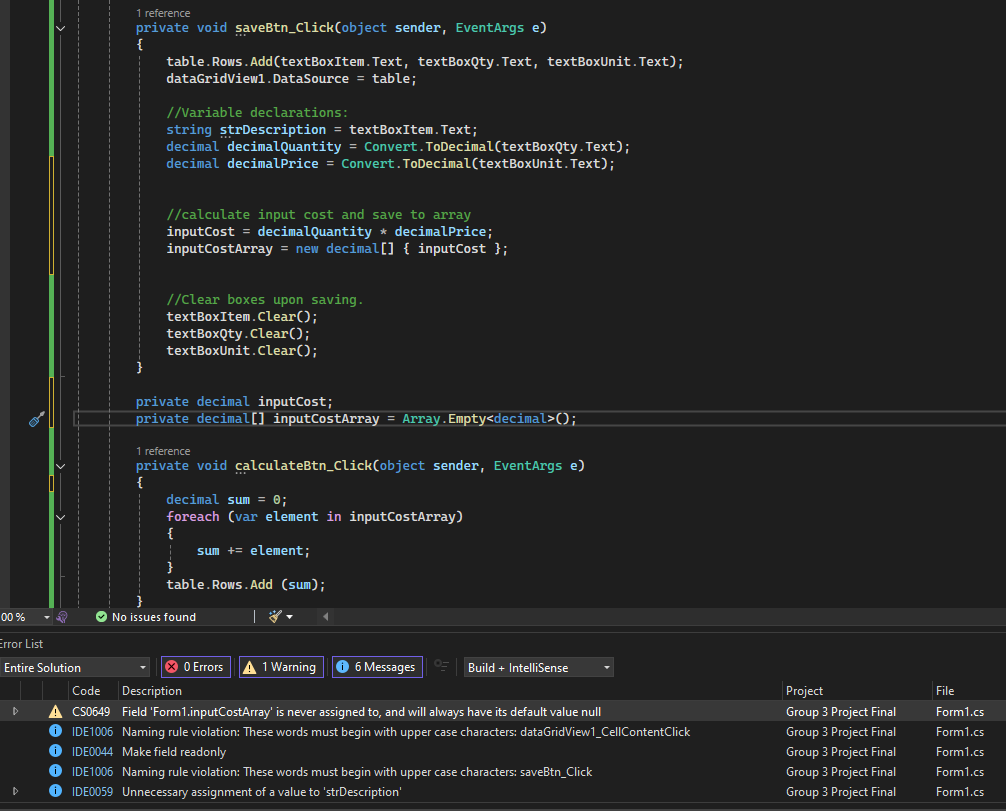
Weird, the warning should not be there
Maybe just VS being confused
Happens
I can try running it and see what it does
Yeah, it should build and run fine
Give it a shot
Let's you calculate, but gives the wrong answer
so, half win lmfao
Okay, looks like it only does the second calculation
Okay, I think I know what's heppening
I start by setting sum to 0, so it basically ignores the first thing entered
I thought I knew how to fix it but I am once again stuck, sorry
Well, right now you're only calculating a single row
Presumably, you want to calculate a total sum, right?
If so, you will have to go over all rows of the datagrid
if I'm not mistaken
Ah, that makes sense
dataGridView1.Items?
It says items isn't valid
would it be .element?
nope
ayy
I think I did it? :D
mm
maybe not, because it should have given me 6 and I got 12 lol
oh wait
Nope, wasn't user error
On the bright side, I think I've completed the final aisde from getting the wrong number 🤣
I am so close, but it just keeps giving me double. I feel like it's because of how I did this, but idk how to fix it
I have to hop off for the day, but if you are back before me, I'd love to know what I'm doing wrong :KEK:
This should hopefully be the last hurdle in coding before I finish it up!