How to create new user using drizzle to better-auth users schema
hey, im using tRPC, drizzle and better auth to handle my authentication ,
i want admin to be able to add new users to the system
// Add a new user
//there is an error here !!
but im facing this error in the screenshot, im not sure if this is the right way to do it
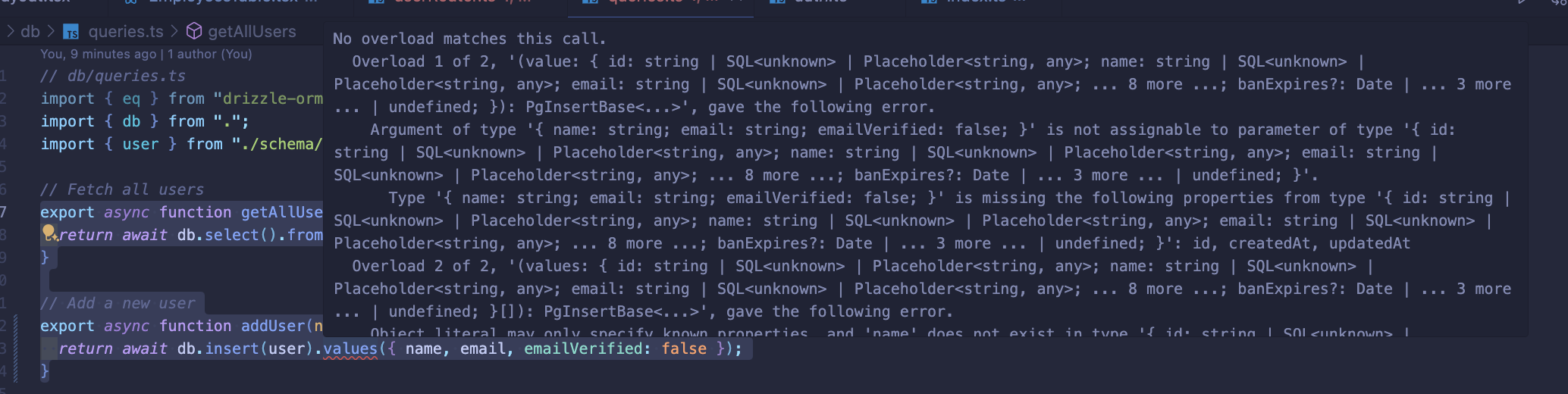
23 Replies
you're missing setting the id
Also why not just use the admin plugin?
i was thinking of this but it sounded weird because i had to call authclient inside my trpc route? im not sure if it's the right approach or not
its basically just a fetch. so it works client and server side
but you could also call it from auth.api
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
i still dont understand why such an error occurs
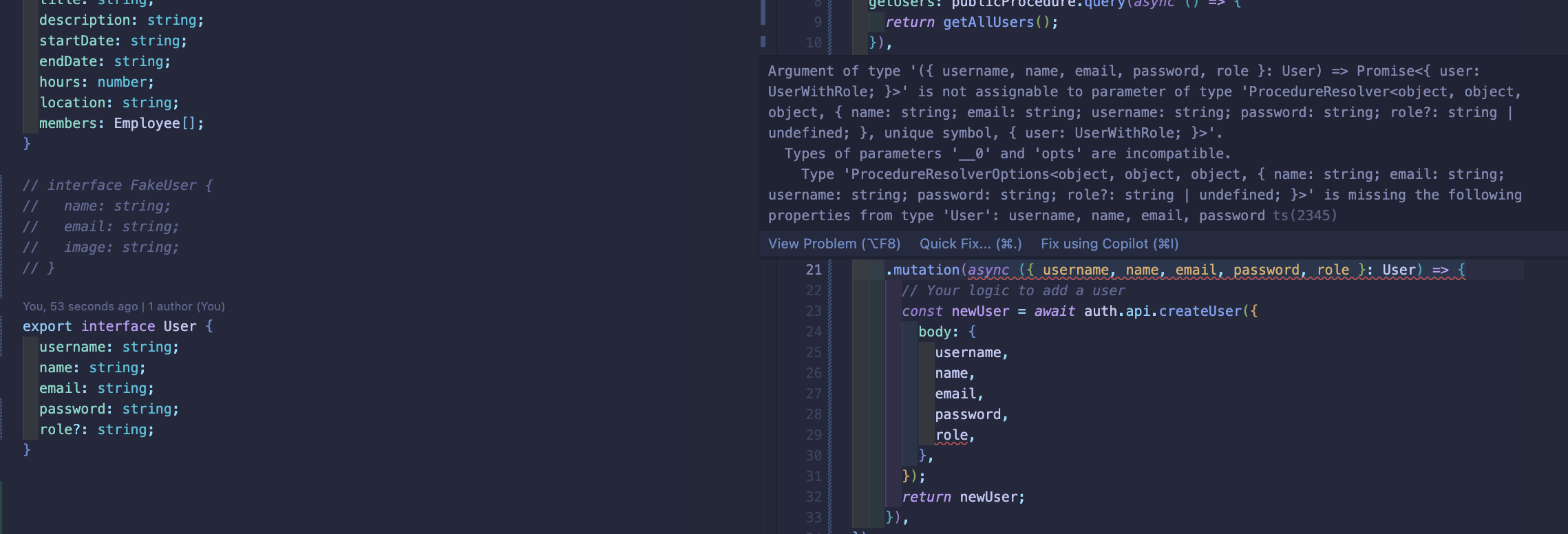
role must be inside data
Object literal may only specify known properties, and 'username' does not exist in type '{ password: string; email: string; name: string; role: string; data?: Record<string, any> | undefined; }'.ts(2353)
body doesnt take in
username
do u use the username plugin?
try also adding it to data
oh no
i had it on my auth client tho
oh yea i have it !!
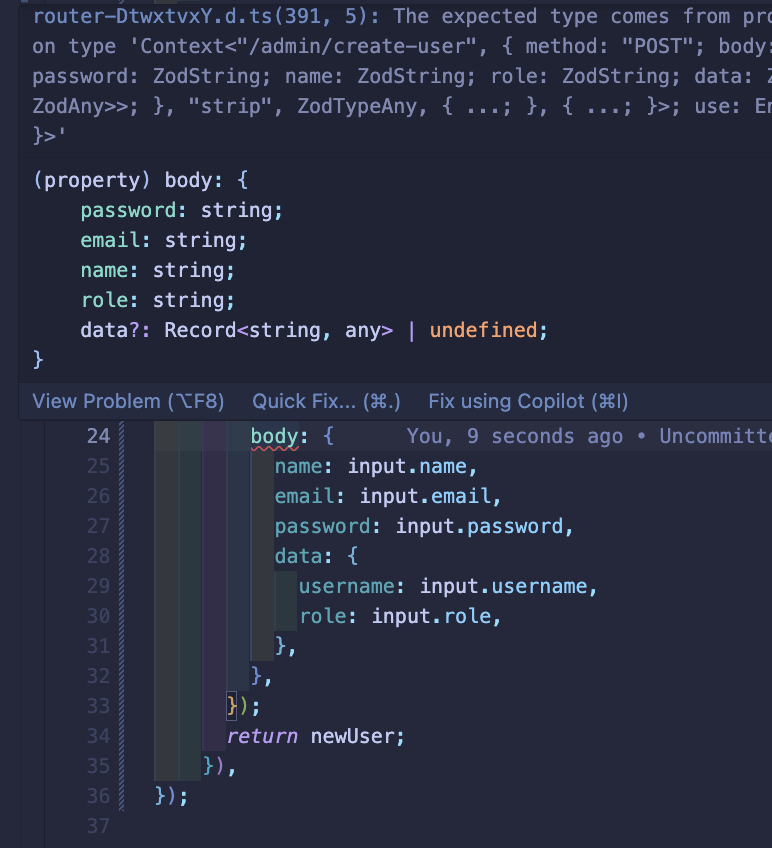
i think i should fill it right?
i have been struggeling with typescript
yes u have to define
role
that's true ,
it worked after
changing
to
let me try
to do my request
yes and you have to specify
role
inside body
gosh
role has to be in
body
not data
yea thanks i did this
although i have logged in using my admin account
try passing the headers on createUser
is it the right way i dont think so
do u use nextjs?
if so, you have to
headers: await headers()
yes, it worked thanks
when redirecting to all employees page it doesnt get added, until i refresh the page , i have to do revalidation in some way i guess
when listing users?
u can write a hook to add your additional fields to the response
Hooks | Better Auth
Better Auth Hooks let you customize BetterAuth's behavior