✅ 2 Databases Identity.ef + my CustomDb FK INSERT issue
So i have webapp with webapi, I've made Controllers, Services etc... all looks correct
I've debugged it and the data passed to models look correct to what i have in SSMS, like the userId.
but somehow when i try to create new TodoList it fails with error:
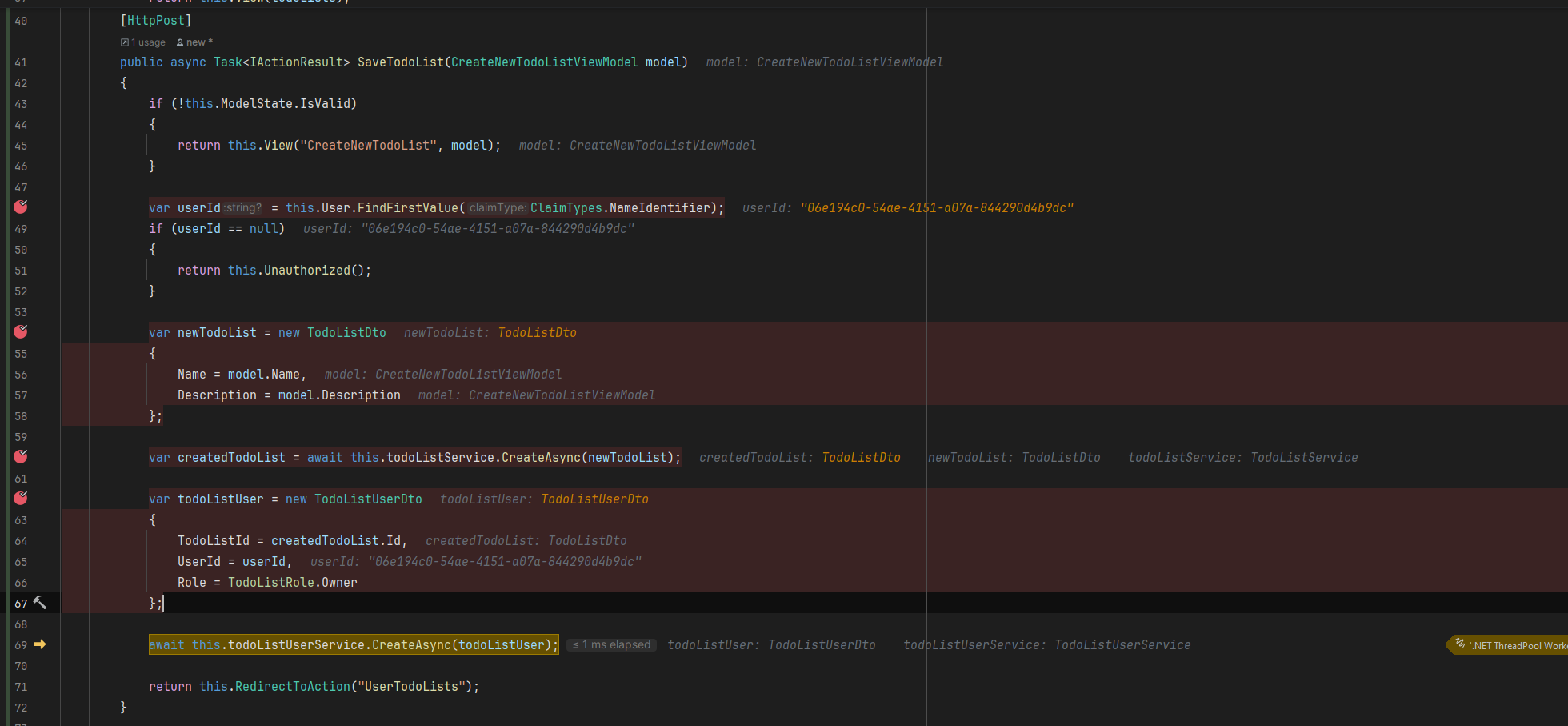
13 Replies
what is the body of
todoListUserService.CreateAsync
?
So im checking this because in DTO i added extra field that tdoes nothing, i will remove it and see
the error is basically saying the user ID you're trying to use isn't a user in the database
have you looked at the SQL being generated to confirm what EF is trying to do?

looks the same to me
so i checked TodoLists table and looks fine
but TodoListUsers table is all null
So after further investigation i am even more confused
why is there dbo.Applicationuser in my TodoListDb, am i not refferencing the UsersDb correctly somewhere?
have you defined a relationship between a user and a todo list item?
you can't have relationships between 2 databases so both tables will be in the same database
is there a reason you have 2 databases?
it was the requrement to use Identity.entityFramework to make register/login
and then i made 2nd db for actual entities
you can use the same database for identity and your app specific tables
you actually have to if you want to have relationships to identity related entities
Oh
ye at first i was really confused to use 2 dbs
so i just merge it all to one db and should be fine?
probably, i'm guessing the issue is you reference an identity user so a table is being created for them with no rows in your second db, which leads to the user id not existing where it expects it to
ye when i register the user data is only in UsersDb, and not in TodoListDb even tho it creates a table for user
yeah if you put everything in the same db it should work properly
okay thanks, will update here if all works
So after fixing 91234812 (maybe more) other problems it works just fine with one db. My code for services was ok.
Just had to make one DbContext