Using useListOrganization() causes API to call `/api/auth/t-o-j-s-o-n`
I am trying to design an org select screen in react:
But doing so causes the page to call
/api/auth/t-o-j-s-o-n
about a dozen times rather than the list organizations API:
Copied as CURL:
Relevant client code:
Any ideas why?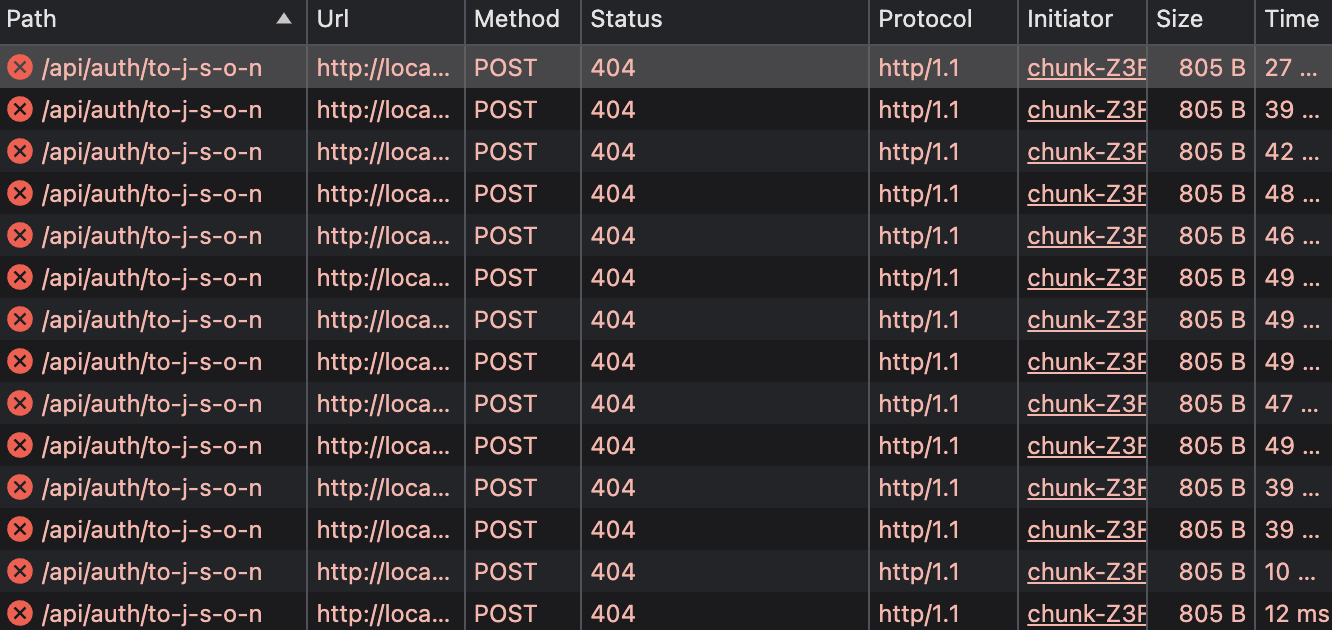
2 Replies
somewhere in your app it's trying to call
authClient.toJSON
maybe you're passing authClient from a server componentI am on non-SSR SPA, so I shouldnt have that issue but I'll double check
solved: it turns out this is caused by tanstack router's dev tools trying to serialize the authclient