✅ Newtonsoft JSON - file not found
i have this code inside a Song.cs class
and my main
I get this error when i should get a patch relative to my project, right?
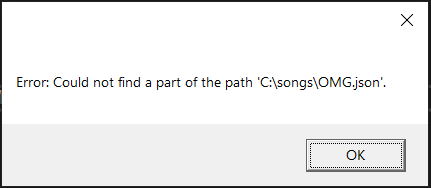
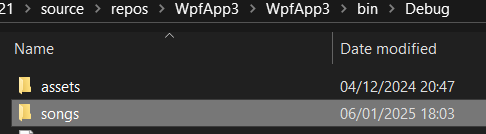
65 Replies
A path relative to the project would be more along the lines of
./songs/OMG.json
or sonds/OMG.json
/
or \
implies root, in case of Windows it's the root of the current drive
Ah, wait, you're using CurrentDirectory
...
Try Path.Join
instead of Path.Combine
.Combine
discards previous root if a new root is found somewhere in the path parts, so it's possible it discarded .CurrentDirectory
when it encountered a part that starts with \\
Path.Join
just joins it straight
uh
Are you using some ancient version of .NET?
You must be, Path.Join exists since Core 3.0
.net framework 4.8.1
So yes, you are using an ancient version of .NET
That's probably why you also needed Newtonsoft instead of using the built-in JSON serializer
$newproject
When creating a new project, prefer using .NET over .NET Framework, unless you have a very specific reason to be using .NET Framework.
.NET Framework is now legacy code and only get security fix updates, it no longer gets new features and is not recommended.
https://cdn.discordapp.com/attachments/569261465463160900/899381236617855016/unknown.png
For reference
is it possible to upgrade the code instead of making a brand new one and copy pasting?
You can try to continue using .Combine, but with a path without any slashes in front, so
It's not always easy, but it is possible
Upgrade Assistant can be helpful: https://learn.microsoft.com/en-us/dotnet/core/porting/upgrade-assistant-overview
.NET Upgrade Assistant Overview - .NET Core
Learn more about .NET Upgrade Assistant for .NET-related projects. This tool helps you upgrade from older versions of .NET, including .NET Framework, to the latest version of .NET. Code incompatibilities can be fixed as part of the upgrade.
also i was told to use .net framework because im also using teh kinect sdk
Erich21
Hello, I'm currently trying to create a project using kinect for windows (I know that it's dicsontinued, but I find it interesting).
However, just trying to follow a basic tutorial I ran into this, and I don't know how to fix it.
When searching it up online, I don't understand what I'm supposed to do to resolve it.
I'm using Visual Studio 2022, and I made a .NET 6.0 WPF project
Quoted by
<@155030370868396032> from #kinect for windows (version 1/ x 360) help setting up (click here)

React with ❌ to remove this embed.
Ah, well, RIP
well now its just
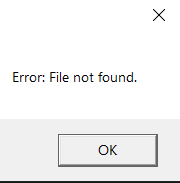
altho im just very confused
See what
newPath
ends up beingbecause the message box right above the readalltext is correct
Place a breakpoint and check it in the debugger
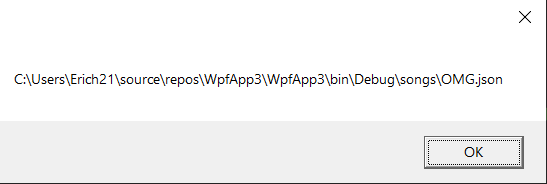
Is it correct?
If so, then you're simply not copying
OMG.json
In the solution explorer, right-click it, open properties, and mark it to be copied alwaysC:\Users\Erich21\source\repos\WpfApp3\WpfApp3\bin\Debug\songs\OMG.json
is the json file there?
but if i want to add more files in the future, shouldnt the code already work like this? In cases where I end up adding lots more, do i have to manually add them to the project too
go into the exact path and check
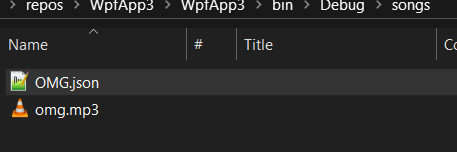
IIRC you can set the whole directory to be copied
if i go to "add existing item" it makes me select only one file
u can do it twice
put this into your .csproj and it copies on each build everything inside to your Build dir
this worked
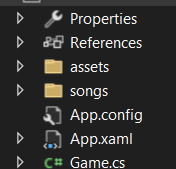
ty
however still no luck :,)
whats the issue now?
.
wdym by "it worked" then?
as in, my folders are properly organised and copied in the output directory
oh, ok
try adding the files to the project
they are
both in the project directory and the output directory
can you show?
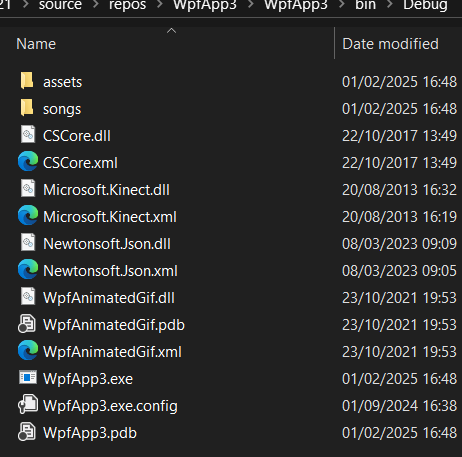
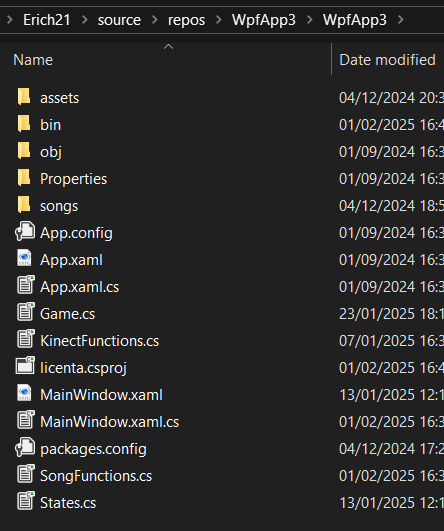
i ment inside solution explorer
Project>Add existing elements
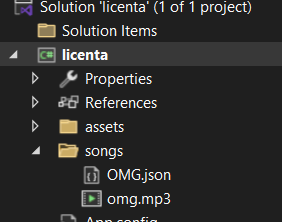
hmm
then you can just do songs/OMG.json
try it
same "file not found" message
wierd
right click file and show propertie
s
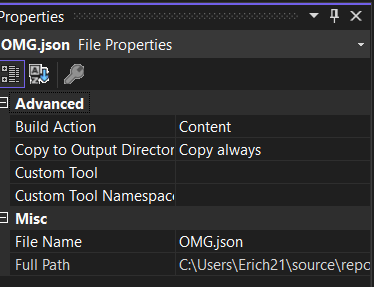
try changing Build Action to Resource
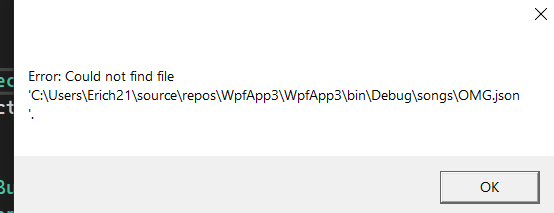
no clue then
bump
@Erich21 Is that path correct?
What happens when you paste that into Windows-Key + R, followed by hitting the enter key?
And can you show the current code again?
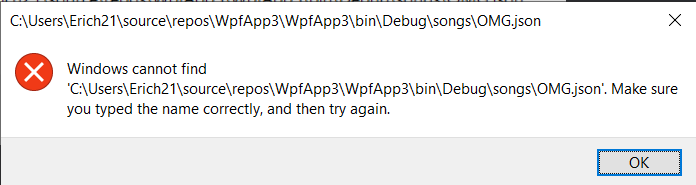
hold on my file got deleted???
ok i put it back
it opens notepad to let me edit the file
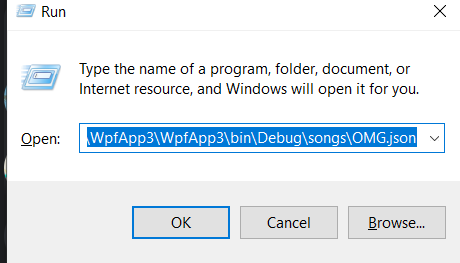
I have no clue why it is throwing
Sth must be wrong obviously
Can you debug and show us the value of
newPath
?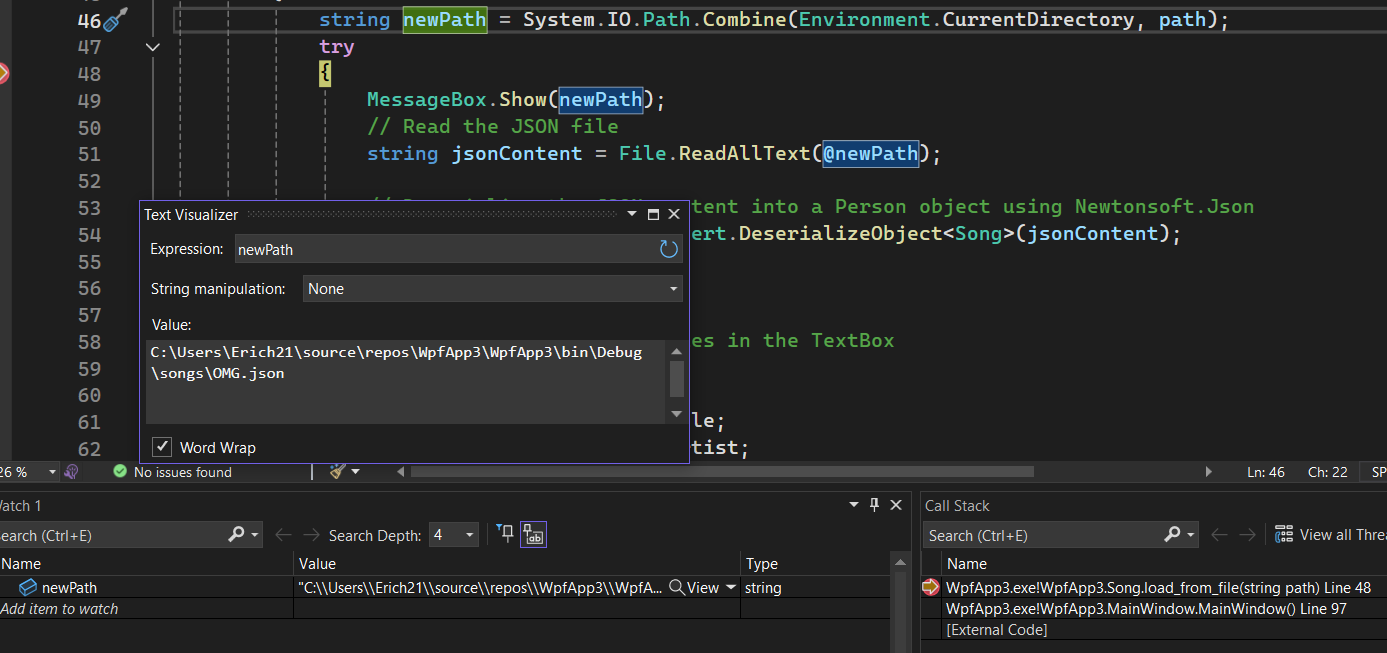
And you are still sure that the file exists? Because you mentioned that it was deleted which sounds interesting to me
yes
doube checked rn
i have no problem going on voice chat or something
ok update: and this is something very weird
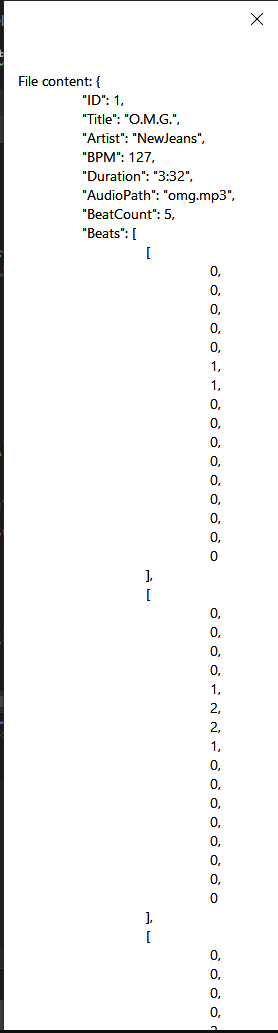
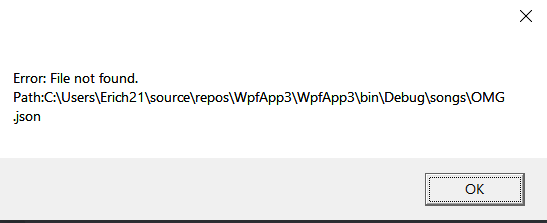
how come is it giving a file not found error/exception if it just managed to read its contents?
and the usage of these functions comes from the actual documentation
https://www.newtonsoft.com/json/help/html/DeserializeWithJsonSerializerFromFile.htm
Where does it actually throw the exception?
Did you debug that?
Because we have to make sure that the code after the deserialization is not causing it
The handling of the exception is a bit misleading because it might not be
newPath
which is causing it here
I usually tend to log ex.ToString()
because that also includes the stack trace which is often times really helpful. Especially when dealing with rare exceptions
you were right
it was trying to find the audio path, not json path
it was such a small detail i didnt even notice after so many tries
this helped so much
tysm
im so sorry for not noticing it before
You are welcome!
Happens to the best :when:
!close
Closed!