useContext returns undefined
Hi there! I am making a game launcher wich looks like an OS in frutiger aero style with Solid JS. I have a ContextProvider wrapping my whole app like so:
And i use the context inside a
DraggableWindow.tsx
here:
The context works inside the DraggableWindow
, but when I try to use it in it's children it returns undefined
. I use components to spawn a window with content.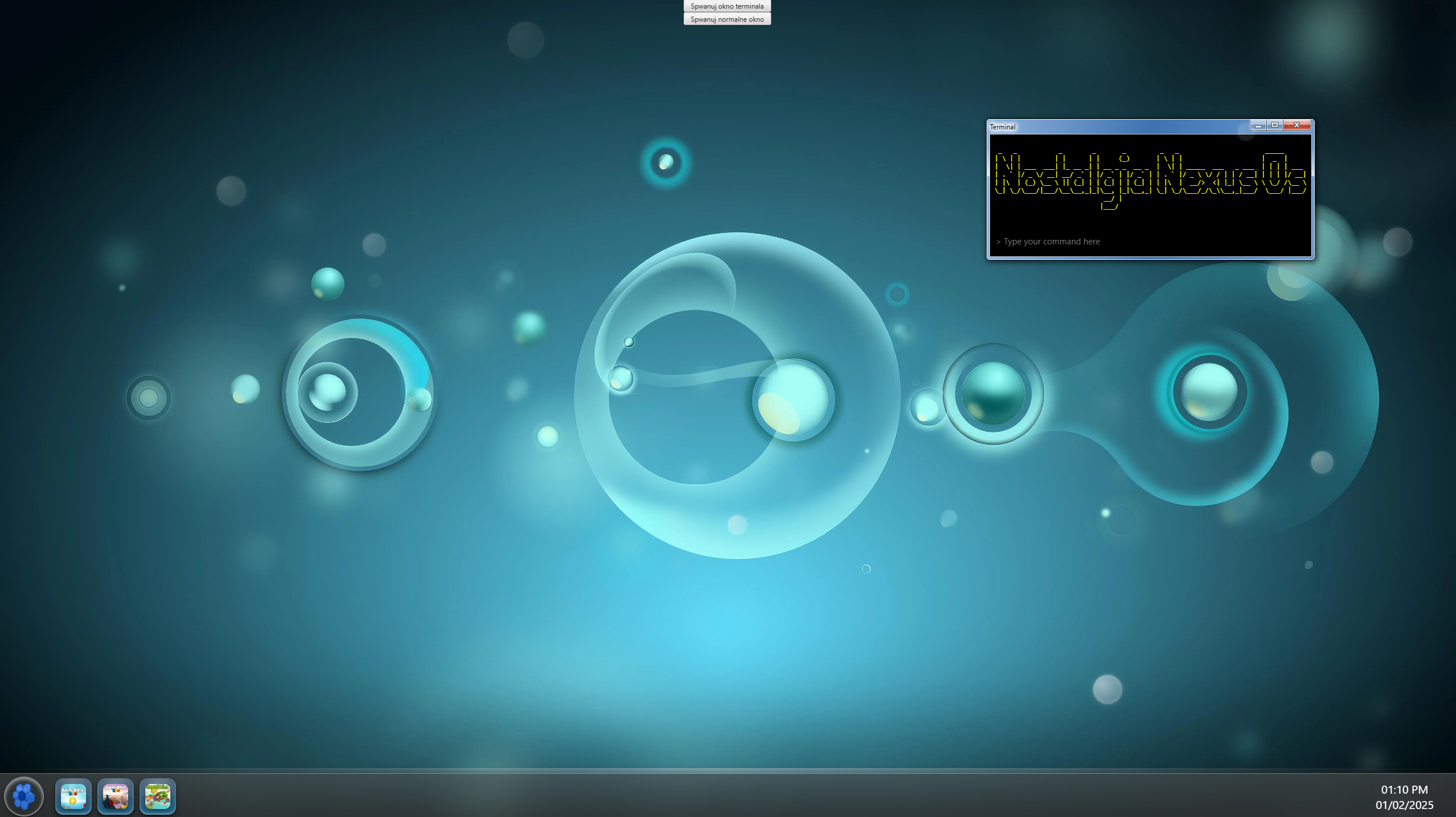
32 Replies
Full context code:
this is where the problem is
i believe the
createRoot
wrapping was to silence the computation created outside of a root
warning but that's not its purpose.
doing content: <TerminalWindow/>
runs that component at that point inside the event handler which is outside of a root and context won't work because of that, you might work around it by using runWithOwner(owner, () => {...})
but that also means you need to know the owner ahead of time.
instead, do content: () => <TerminalWindow/>
, so that in a sense you're storing a component (components are essentially just functions in solid), then you can use <Dynamic component={...} {...props} />
at the consuming sideOh cool so I can just use
content: () => {}
to spawn a new window?
Leeme try itonly doing this will work but I'd still recommend handling with
Dynamic
oh my bad
i forot to remove createRoot
This sollution worked thanks man! š
Ahhh now I can see why do you prefere
<Dynamic component={...} {...props} />
over () => {}
. It's much cleaner. šhmm, I meant at the consuming side, which is inside For children callback in original example
Are there any performance differences between those two?
it's still
content: () => <TerminalWindow />
or () => { ...; return .... }
as long as it doesn't execute immediately
Dynamic might have a little overhead
you can also directly call it
window.content()
oh ok ok
I read docs now I didn't know about it before
Anyways thanks man
which part?
I mean I didn't know about
<Dynamic>
Cuz I've never used it beforegot it
you can also pass tag name in
component
like component="div" ...
very useful for dynamically constructing dynamic contents šš
Project looks cool!
Thanks! I use
7.css
boot screen
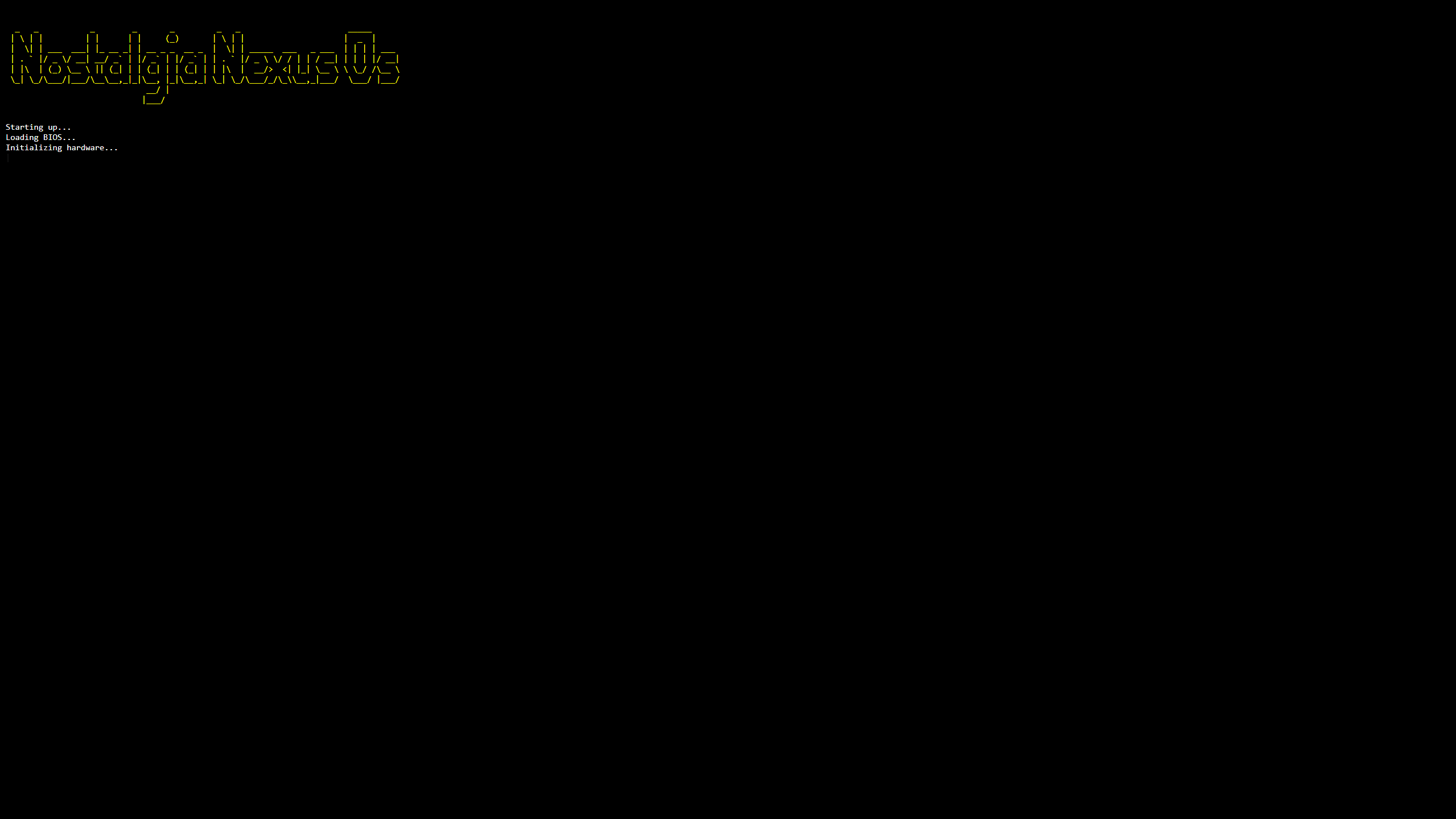
and a login page
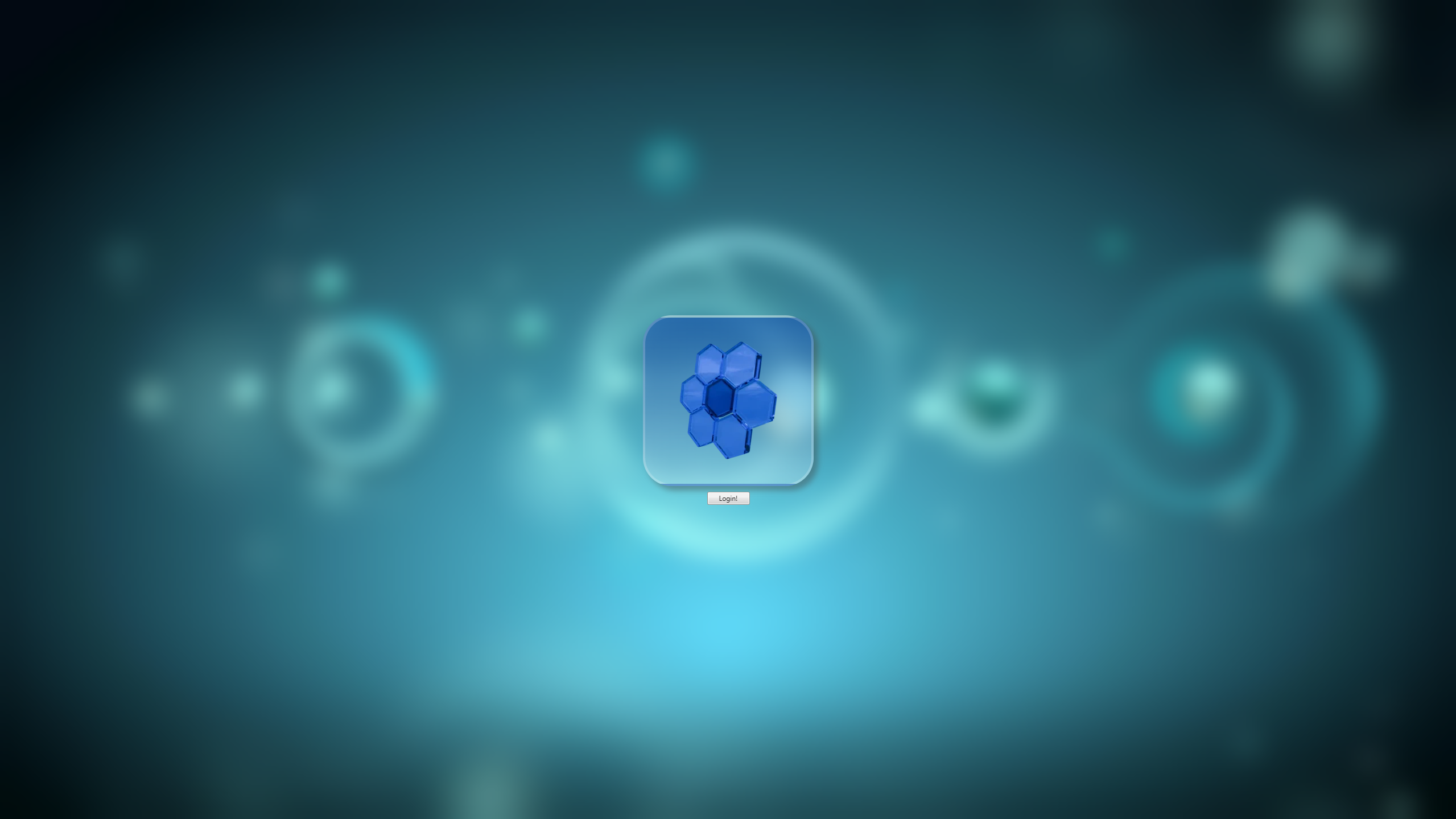
I am trying to match
frutiger aero
style.Our promised future š
Here is a video wich I already made about it
Idk if I can send it here šØ
But discord limit is 8 MB only so...
Here is how it looks like so far
š
another look I am making an api to be able to extend it with api os calls
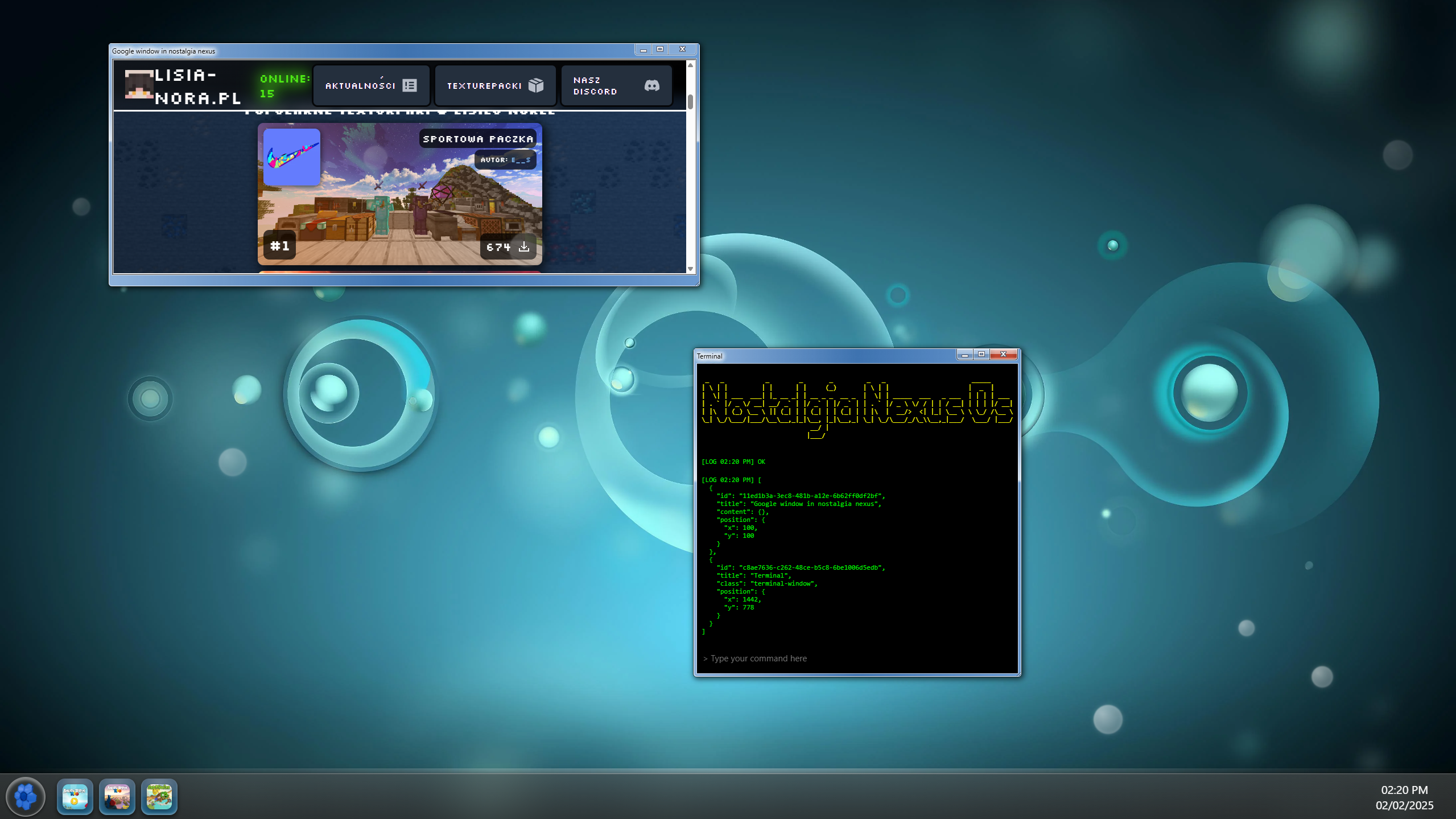
example
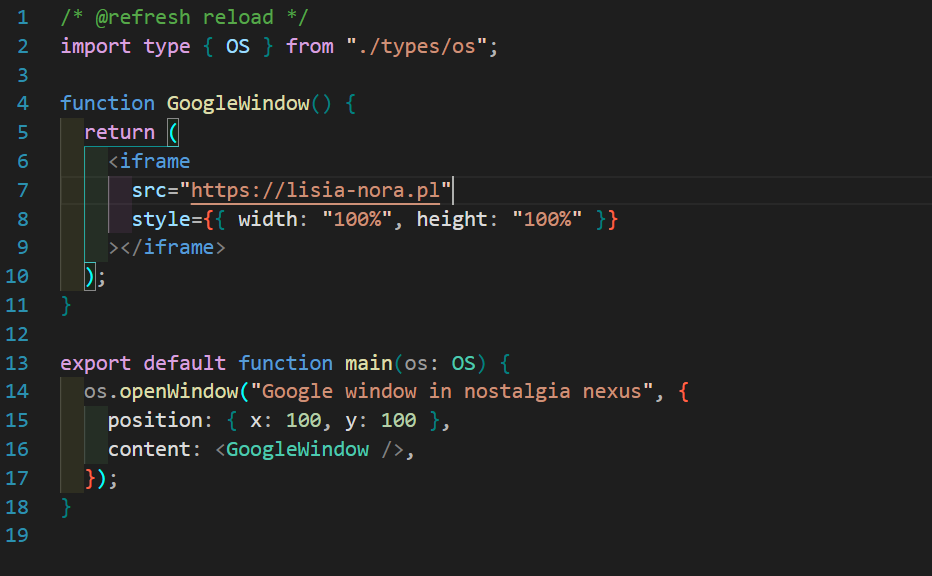
fuun!
Yup I am thinking about making it extendable by using os api like so
You just write a default function wich accept os as argument
On load it just provides the object
To call
example:
And now after reboot we got:
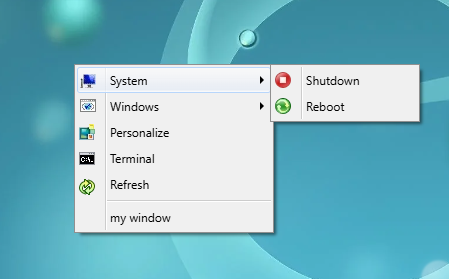
result:
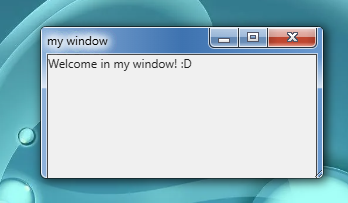
and by calling
console.log(os.windows())
you can see all windows in os.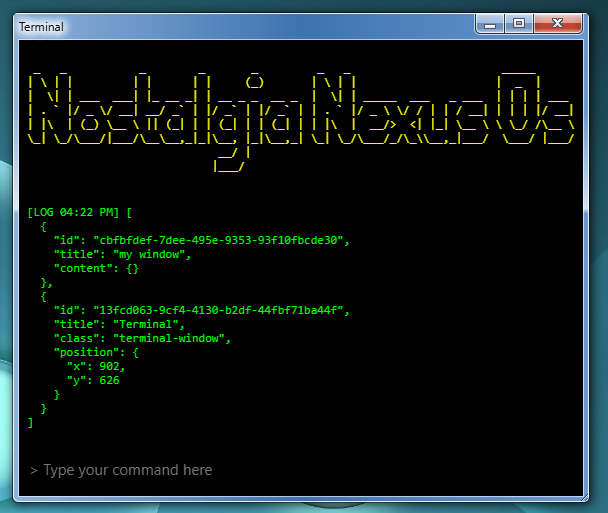
package compiler
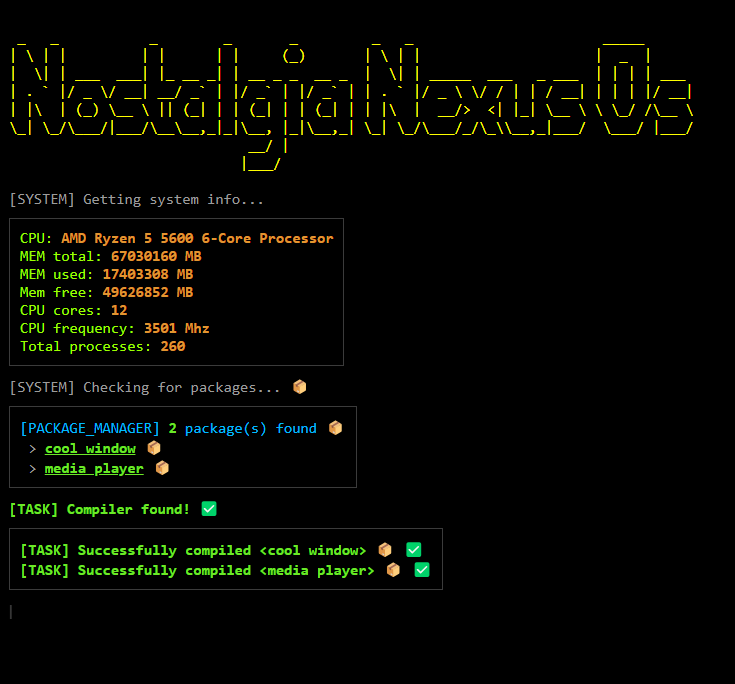