CRUD webapp Form is accepting empty responses after applying validations
I am stuck in making CRUD app first i Downloaded packages of EF then i create model class and db context and create migrations then i start creating my first view for creating/inserting data but the problem is when i run the program and try to insert nothing happens it only redirects me to index and when I open database to check wheather it is enter or not nothing was shown and also validations are not working
#web
16 Replies
Gonna need to see some code
yeh just 1 min
posting
visual studio is crashed
HomeController.cs
@Angius which part i first show here is the controller code now sharing view codeI think I can already see the potential error
ok and what is that
Presumably, your form doesn't fill all the properties of
Student
, since it's a database entity and the form wouldn't have fields for things like ID or foreign keys
You should be using a viewmodel here
Make a class or a record that contains only what the form sends
Then you can even skip the [Bind]
wait
AddStudent.cshtml
see here is my viewYeah, and
Student
model?
and here is the dbContext
Yeah, the form does not fill the
Id
Hence the validation error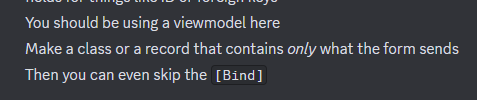
ok i am getting confused can u describe a little bit where to create this class for all my records
Wherever
and what to write in it
can u share a tutorial for that please
Just the properties that the form sets
ok
@Angius
now i have added my viewModel
but i am thinking what to do with this becuase my Sir didn't tell me this thing i saw this thing for the first time so i check online tutorials
now what to do
You use that to construct your database model