Compare Strings better way.
Ok so i am in need of a program that will generate a string that i can copy and paste that string on Dedicated Server for a game called Arma 3.
Now i have a file with all of the Mods currently installed on the server witch is a simple txt file.
Also i have a HTML file that is a current modpack for the mission we want to play.
Now i would want to get the HTML and TXT file and compare there strings.
Example: HTML has a mod, I check if that mod is on server if it is get the name of the mod from the server and add it to ouput string and carry on to the next mod.
But I have encounterd a problem where if one of the mods would contain part of the name it would give that mod.
Example: I have mod in html called @ace.
Now i would like to grab that name from the server file in this case it would be same @ace. but it grabs
@[GM] Placeable Bridges;
because it contains ace in the name Placeable now how would i fix this:
Code:
https://pastebin.com/brMBLpabPastebin
String Comperison - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
46 Replies
Here is also Server mods txt and Modpack html:
you are checking with Contains, maybe StartsWith or match the whole name instead
I cant match with the hole name becouse in Html file the mods is called like this:
@LAMBS_Danger.fsm
But on the server is called like this:
@LAMBS_Dangerfsm
And there are couple of mods that dont mach the nameing convetion.
Basicly i was comparing them with the name in html and geting the value name from server mod.if they're that different, not even your current approach will work
Yea basicly i am asking what would be best aproach to this.
maybe if you normalized the names, all lowercase, strip punctuation etc. but always a risk for false positives
That would be fine but There are just to diffrent to compare like some mods have () some dont.
Could do fuzzy string matching
Levenshtein distance, or some existing library
is the only difference they have that on one side they can end with
.fsm
and on the other it's always fsm
?
are there other differences? other extensions?No here are couple of examples i mange to catch that are diffrent:
HTML:
Server Mods:
Seems like lowercasing and stripping non-alphanumeric characters would be enough to find matches
one adds punctuation though :/
Strip non-alphanumeric chars from both sides of the comparison
oh maybe
worse for perf
but yeah like sehra said it has the potential for false positives
we are parsing html, perf may not be an issue
low potential though
but also look at the ABS one
maybe if you also normalize whitespace
i'd keep letters and digits and match on that. not end of the world if there is a false positive match. best effort
ye
How would i do that sry i am still new to coding 😄 ?
Whoops
Angius
REPL Result: Success
Result: IEnumerableWhereIterator<char>
Compile: 329.588ms | Execution: 30.828ms | React with ❌ to remove this embed.
Then
new string()
that, or string.Join()
itConcat
Right, yeah
ero
REPL Result: Success
Result: string
Compile: 292.653ms | Execution: 58.315ms | React with ❌ to remove this embed.
'Ere you go
.ToLower()
it for good measure too
Or compare with StringComparison.OrdinalIgnoreCase
I tried to do it like this:
But i have error:
Value cannot be null.
Parameter name: text
C:\Users\jorda\source\repos\TestConsole\TestConsole\bin\Debug\TestConsole.exe (process 24124) exited with code 0 (0x0).
To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically close the console when debugging stops.
Use the debugger to see what was
null
So matchingElemts is:
matchingElements {string[0]} string[]
And Outputstring is null
But output string should just be collecion of matchingElements joined with ;
So i tried doing this:
And it returns like this:
Checking 'CBA_A3' -> Match Found: False
Checking 'ace' -> Match Found: False
Checking 'Enhanced Movement' -> Match Found: False
Checking 'KAT - Advanced Medical' -> Match Found: False
Checking 'Enhanced Movement Rework' -> Match Found: False
Checking 'DUI - Squad Radar' -> Match Found: False
Checking 'Task Force Arrowhead Radio (BETA!!!)' -> Match Found: False
Checking '3den Enhanced' -> Match Found: False
Checking 'Better Inventory' -> Match Found: False
Checking 'cTab NSWDG Edition' -> Match Found: False
Checking 'Better CAS Environment (BCE)' -> Match Found: False
Checking 'Zeus Enhanced' -> Match Found: False
Checking 'BackpackOnChest - Redux' -> Match Found: False
Checking 'Ghost recon 3rd Person Camera' -> Match Found: False
Checking 'Immerse' -> Match Found: False
Checking 'Suppress' -> Match Found: False
Checking 'RHSAFRF' -> Match Found: False
Checking 'RHSUSAF' -> Match Found: False
Checking 'RHSGREF' -> Match Found: False
....
But there should be there.
Since you're not using the debugger, print the
txtItem
as well, to see if they are or aren't equalCould it be becouse of the @ simbol ?
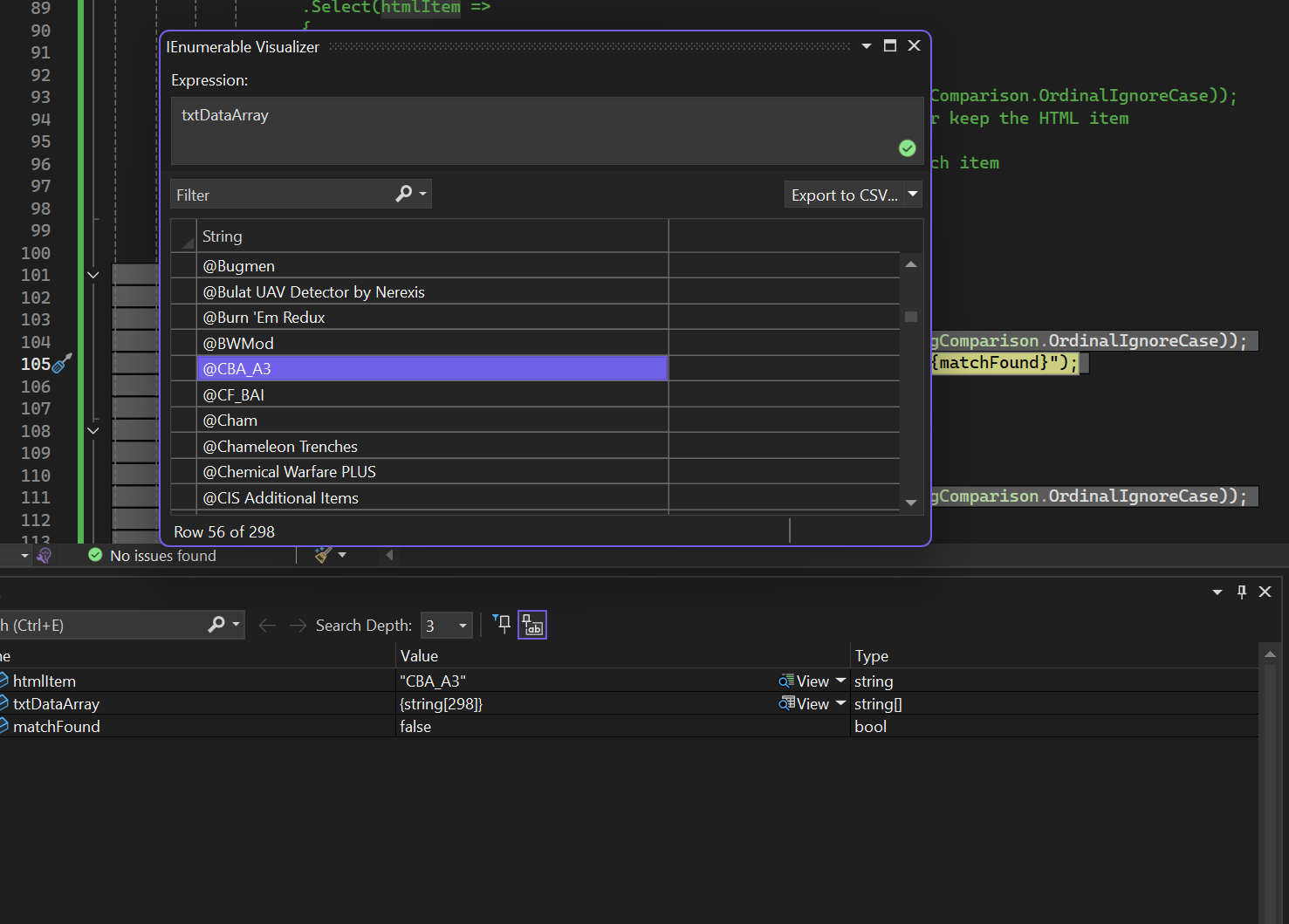
Our
.Normalize()
should be getting rid of thatOk yea i was using diffrent normalize. Now the only problem i am facing is that the output string is basicly getting spamed with first element in serverMods:
Ok so this is my code currently:
https://pastebin.com/yjeAYvw2
So my matchingElements string array is getting filled with only with a first element in ServerMods .txt how would i go and get all of the mods that mached in that array ?
Pastebin
String Comperison 2 - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
what do you want it to output? the mods present in both html and txt?
No i just want it to output the mods on server txt
i don't understand what you mean by that
So basicly
txt - list of mods on server.
html - list of mods for current mission.
get the list of mods from html and txt compare them
and only output the list of mods that are in txt that match the html list of mods.
so those are are in both txt and html
yes
something like that
Where would i put that in this code ?
sec, didn't work as i thought it would
ok, so NormalizeTest should have
str.Where
, not the example string
and the whole matchingElements
calculation can be replaced with the above twoI think i might fixed it i did it like this:
basically what you are doing is set intersection. hence the shorter way using
.Intersect()