Freeze on await client.login()
The log before firing the login function is displayed, but after that nothing happens.
I have set up a debug log, but nothing is displayed.
85 Replies
Hardcoding a token or giving an appropriate string to a function as a token does not change the result
Adding the file “ヘルプ.ts” to the commands folder seems to cause this condition.
I think this is a bug and will file an issue.
I’ll investigate this after I get back home (in around 6 hours)
@Lami that's interesting, failing with Katakana but working with Kanji
Does it also work with Hiragana?
Seems to be working for me with Katakana
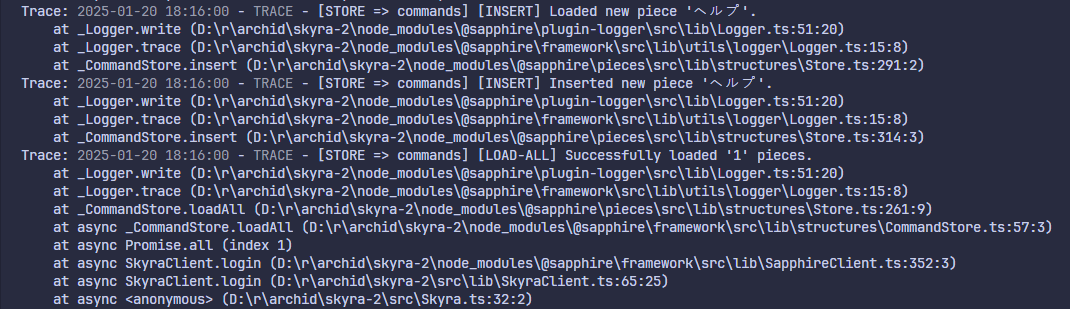

Can you provide me the source of the command? See if it has something that may stop it from working
If you rewrite the Kanji command's file name to Katakana, does it work?
https://github.com/c7e715d1b04b17683718fb1e8944cc28/my-sapphire-bot
I uploaded a project that does not work to Github as public!
GitHub
GitHub - c7e715d1b04b17683718fb1e8944cc28/my-sapphire-bot
Contribute to c7e715d1b04b17683718fb1e8944cc28/my-sapphire-bot development by creating an account on GitHub.
This is only a guess, but Japanese Windows uses Shift-JIS instead of UTF-8.
I just re-tested “Hiragana” and “Kanji” and it stopped working on both.
I'll check regardless
It's still loading for me @Lami
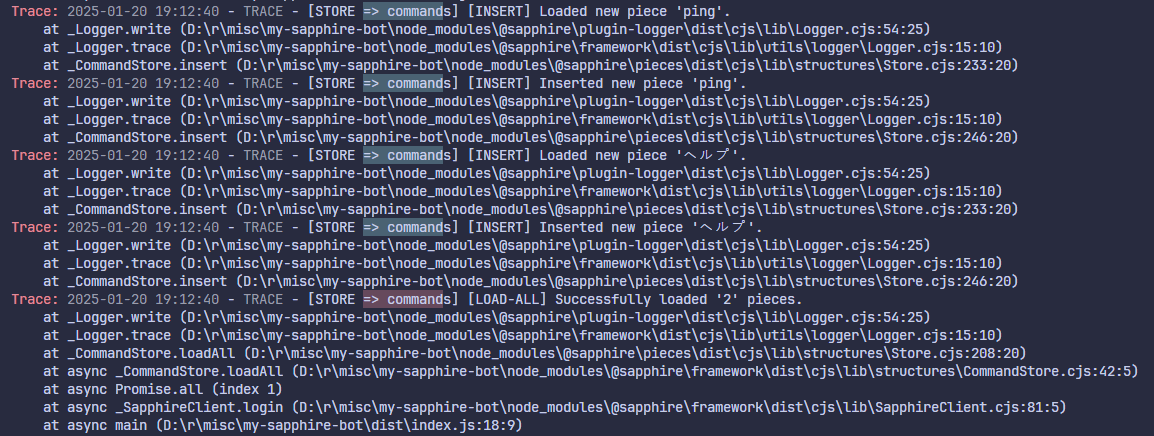

And firing ready
Uhm... I have no idea how to test that, sadly 😅
I do see this tho
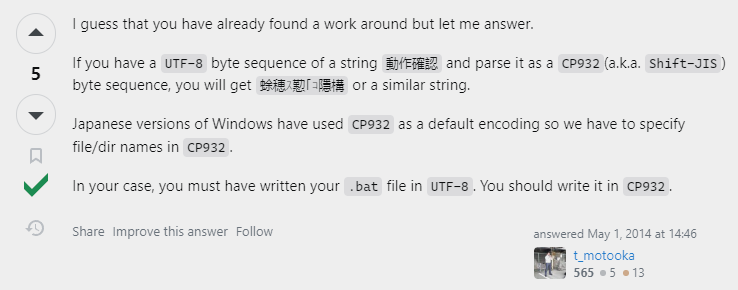
I did find this, however: https://answers.microsoft.com/en-us/windows/forum/all/file-explorer-not-displaying-japanese-characters/c6f8dbb6-bf89-4bc0-9c9e-ca7b4c3ef542
Nevertheless, can you change line 10 in your index.tsAnd check the logs searching for
=> commands
in the terminal?Is that where it ends at?
It doesn't even show the lines for the JS files
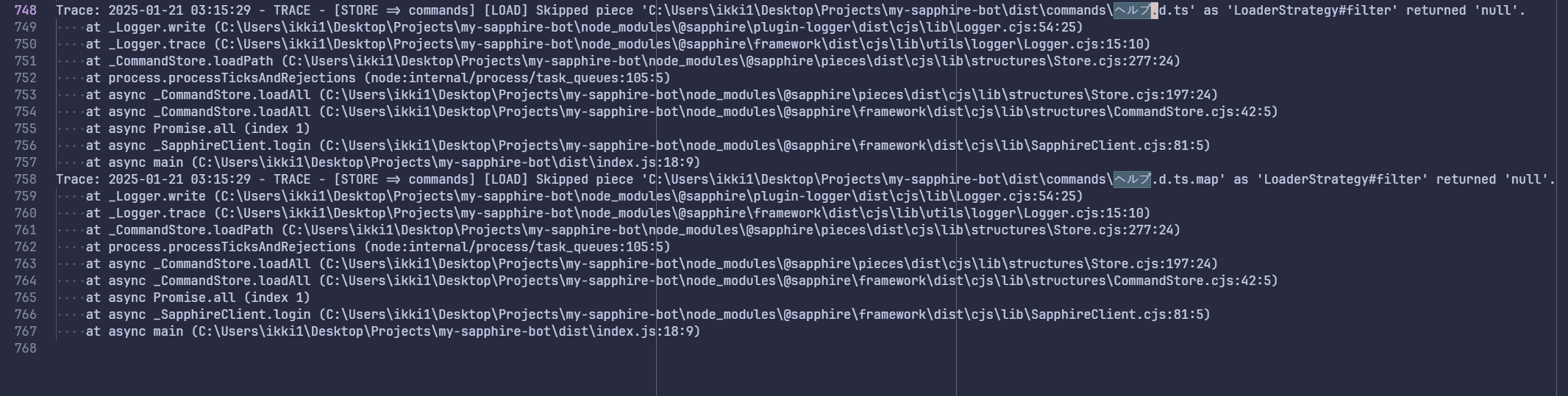
Yes, this should be all.
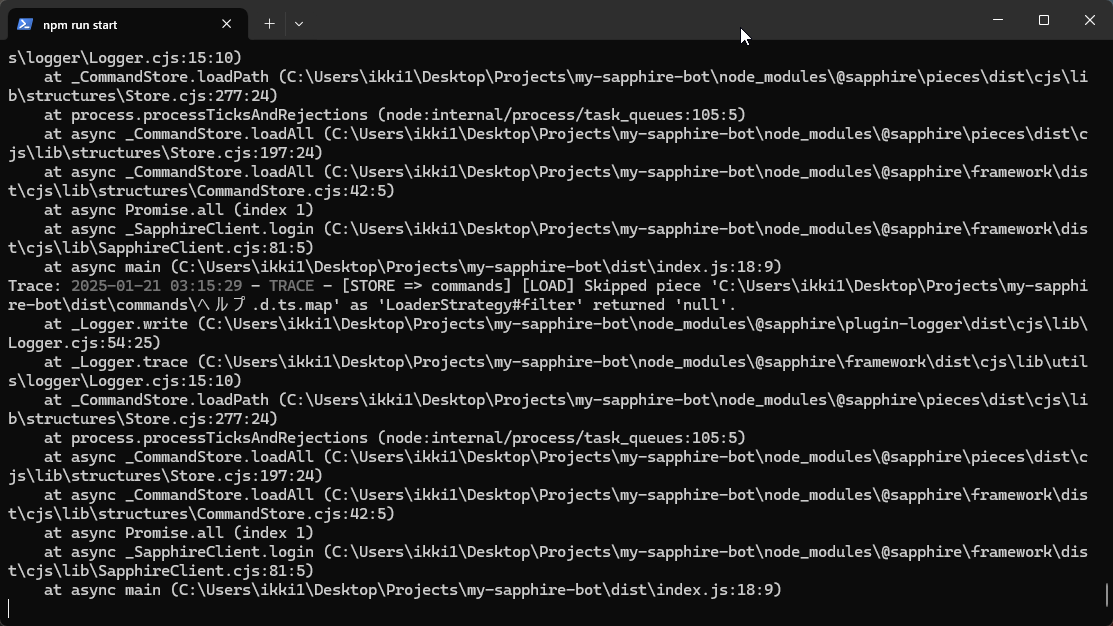
Uh
I can't think of a reason why that shouldn't work
But like, this isn't returning: https://github.com/sapphiredev/pieces/blob/main/src/lib/strategies/LoaderStrategy.ts#L38-L51
If it threw an error, this would return an error:
- https://github.com/sapphiredev/pieces/blob/main/src/lib/structures/Store.ts#L357
- Bubbling here: https://github.com/sapphiredev/pieces/blob/main/src/lib/structures/Store.ts#L256
- Bubbling here: https://github.com/sapphiredev/framework/blob/main/src/lib/SapphireClient.ts#L352
- Which would call the logger: https://github.com/c7e715d1b04b17683718fb1e8944cc28/my-sapphire-bot/blob/main/src/index.ts#L21-L25
Are you familiar with the debugger? I'm afraid you'll have to use it 😅 @Lami
I know of it, but have never used it.
I am sorry.
For this, you would have to set a breakpoint in the line 40 from LoaderStrategy, then fire the bot with F5/Ctrl+Shift+D
Then skip a few times until you reach the command that's written in Katakana
For reference: https://code.visualstudio.com/docs/editor/debugging
Debugging in Visual Studio Code
One of the great things in Visual Studio Code is debugging support. Set breakpoints, step-in, inspect variables and more.
Thank you. I'll try.
I'm also down for screen sharing on Discord, since VSCode's sharing won't work on gitignored files (such as node_modules), and enabling it would allow me to see .env, so I wouldn't recommend it 😅
Does this seem to be working?
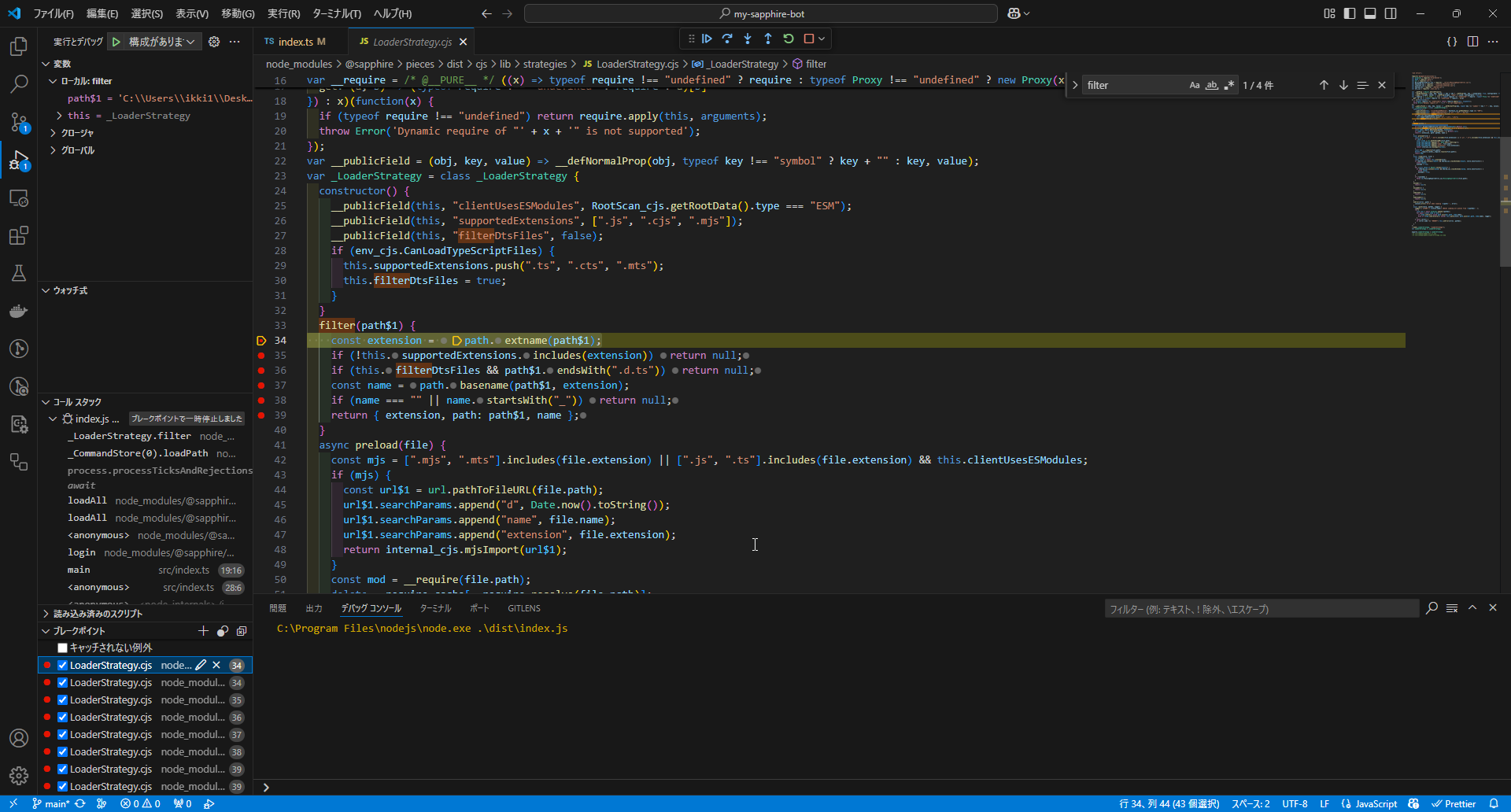
Yes!
Look for the value of
path$1
in the top leftYou can expand it to see the full path
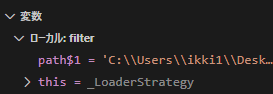
btw to make it easier, remove all the breakpoints except line 34
That way when you hit that button, it'll jump to the next file
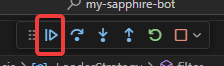
How about this?
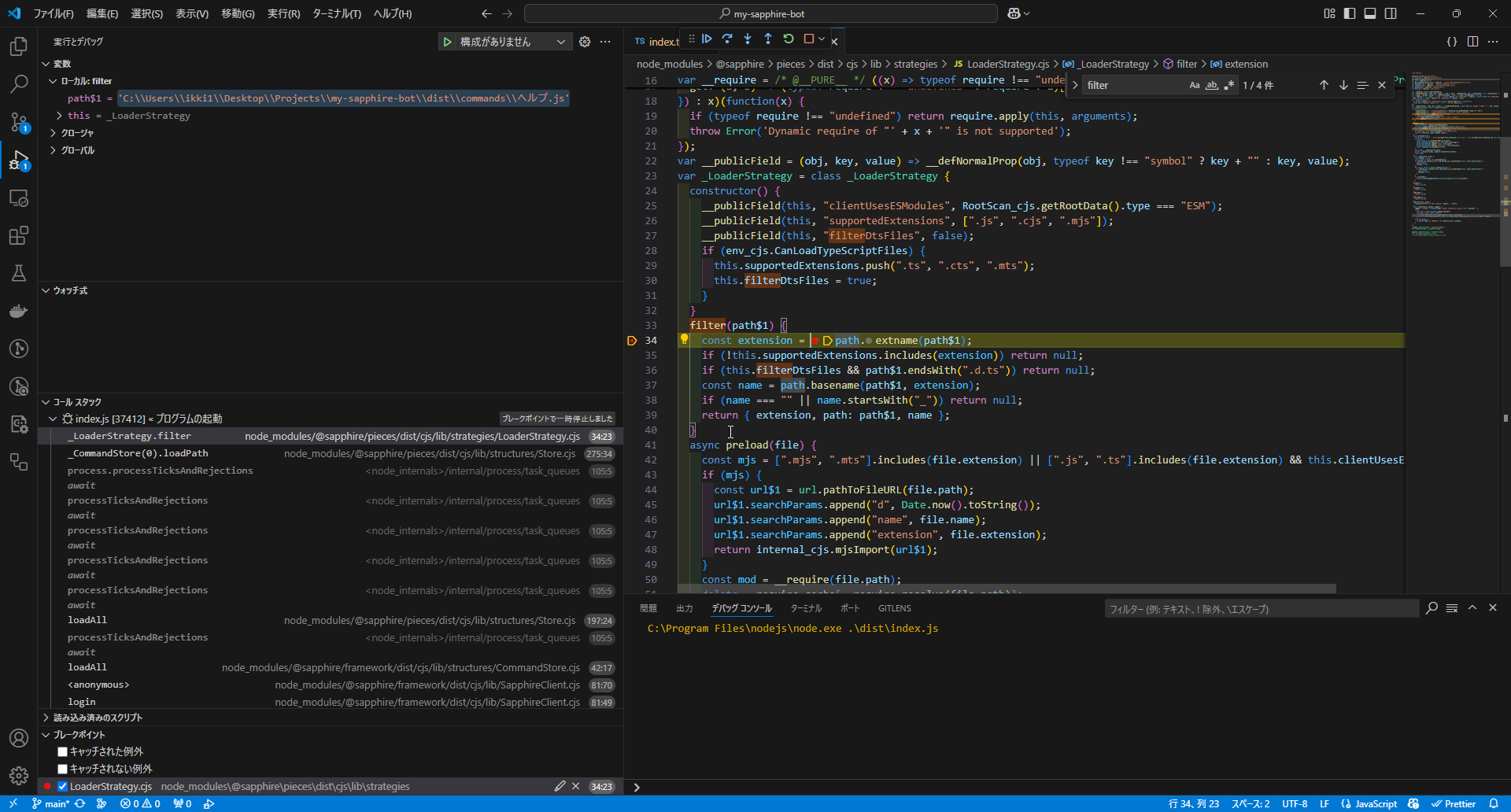
Yes! Now press this button
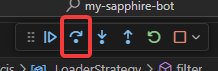
That'll move it to the next statement
I would like to see if
extension
and name
are assigned correctly
They should be .js
and ヘルプ
respectivelyIs this correct?
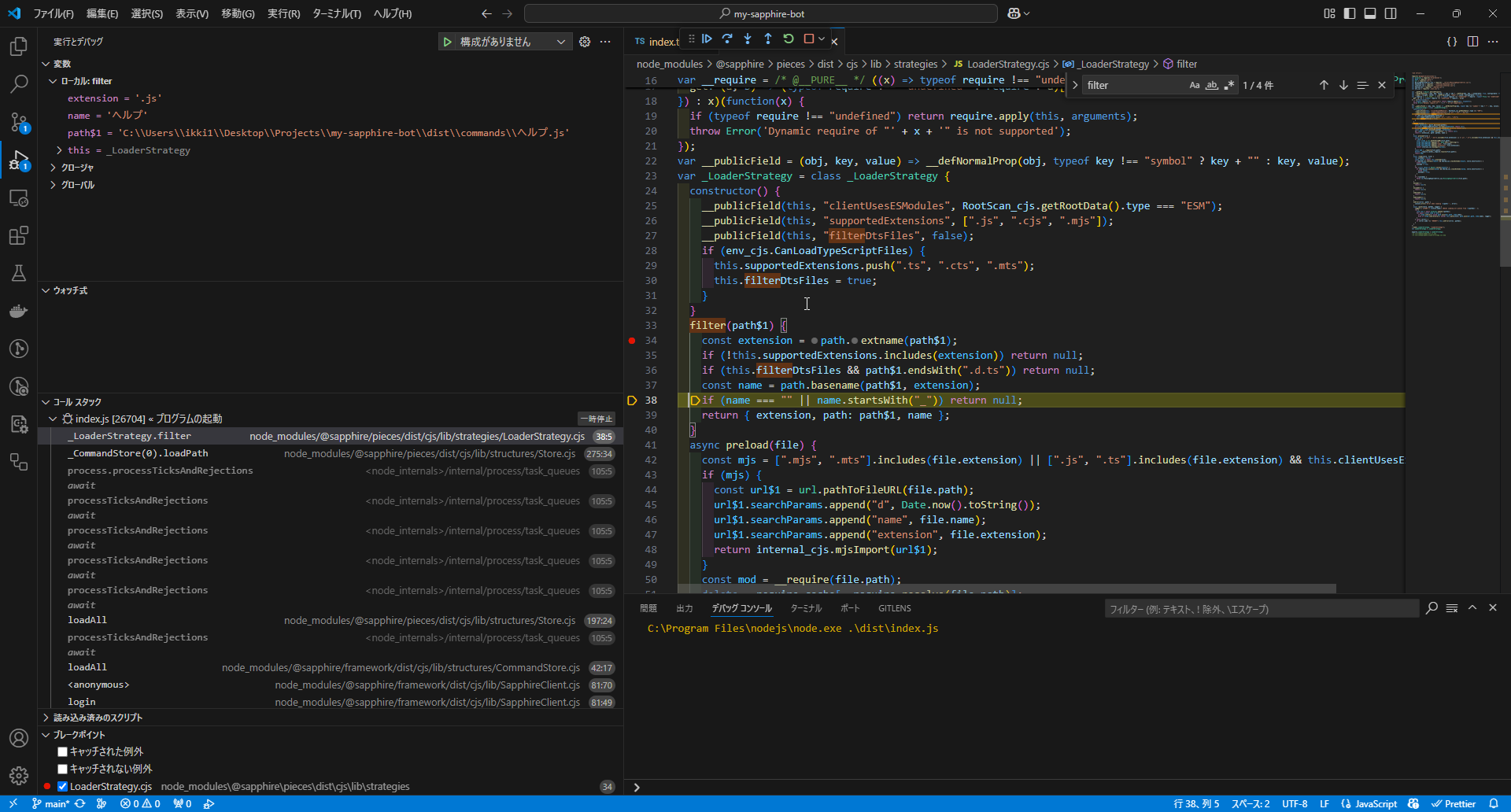
Yes :)
And I know that line 38 won't return null, so next relevant bit is a breakpoint on line 50 until you find the same file, remember to remove the breakpoint from filter ^^
Is this okay?
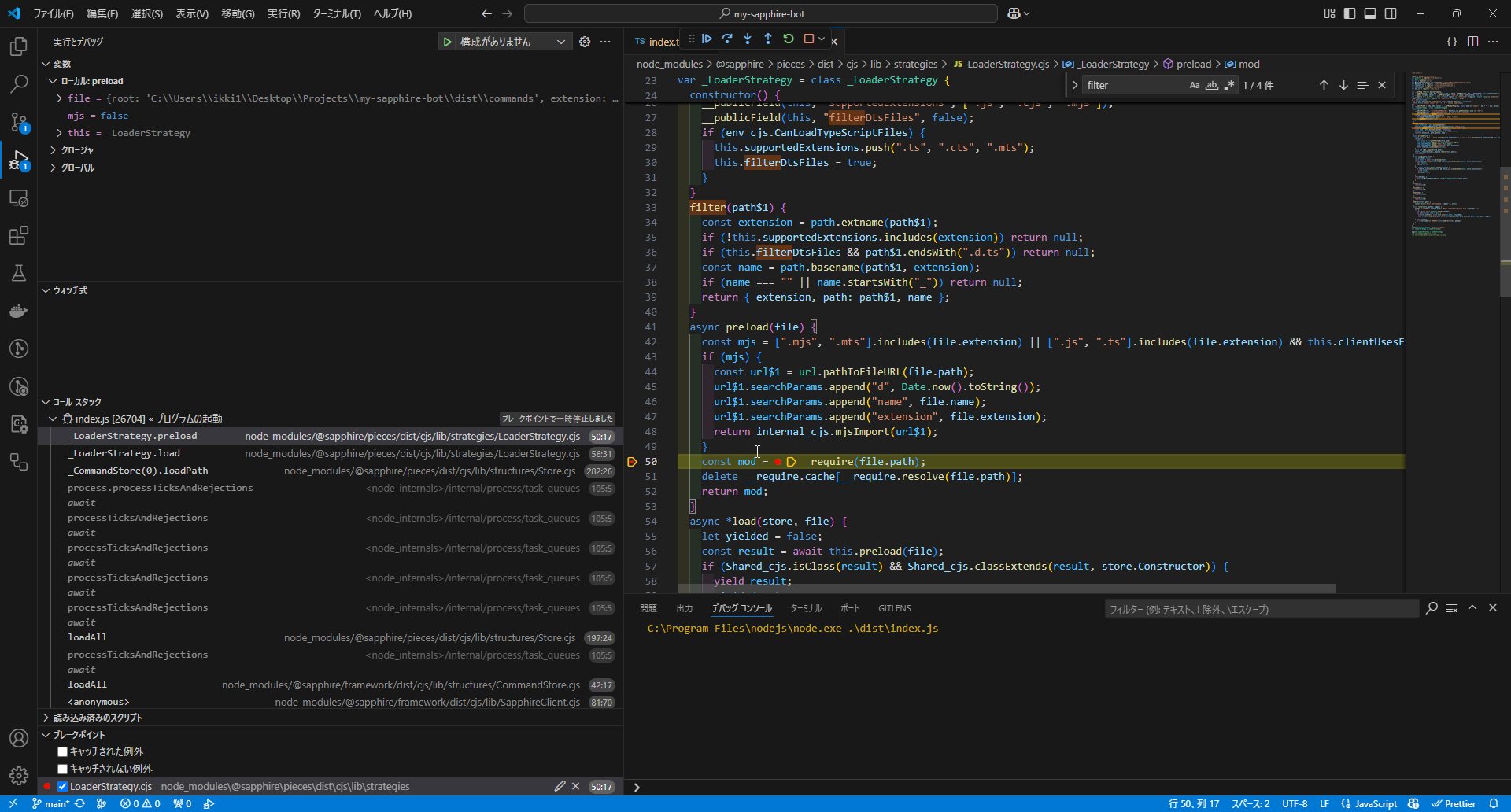
You can expand
file
in the variables to see which file it's on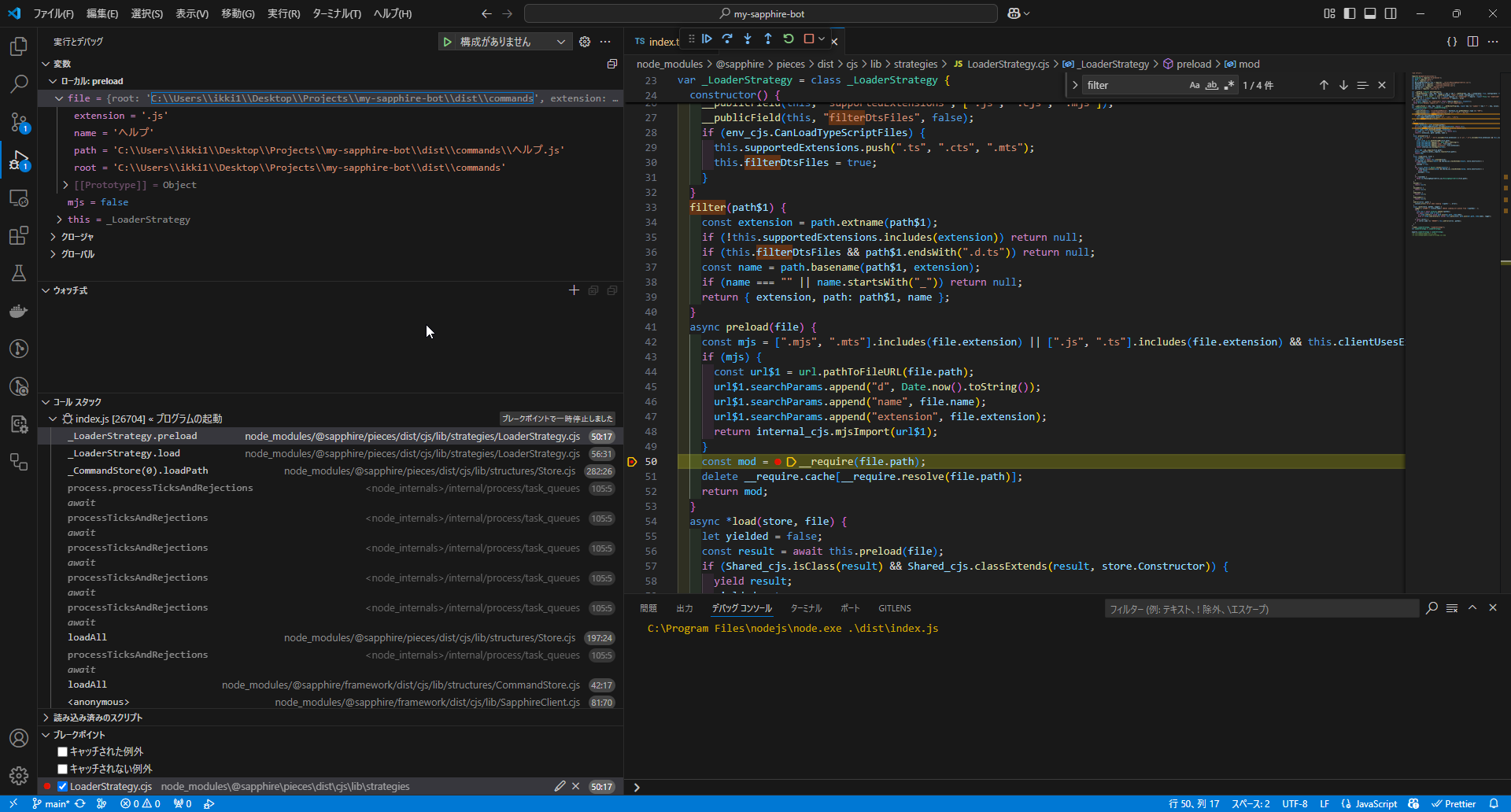
Or under ウォッチ式, add a new variable of
file.name
Oki, that looks good
Go to the next statement to see if it loadsError
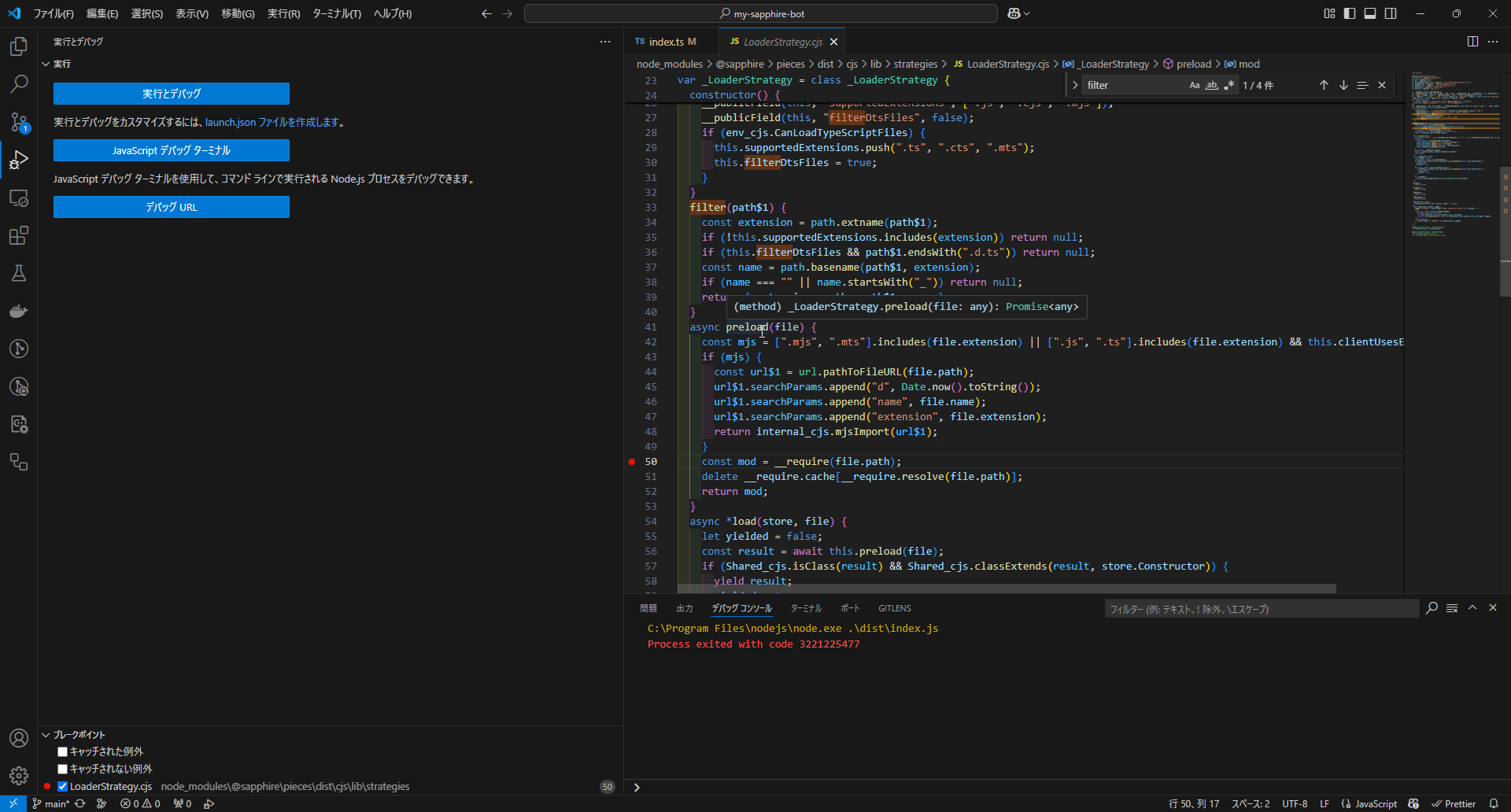
Are you saying that the cause is the current location?
Uhmmmmmmmmmmmm this is interesting
Could you show your screen in a voice channel? #Coding 0 for instance, you can keep yourself muted or even deafened if you want to, it's mostly to see it live and guide you a little better and faster than screenshots
Okay, I'm in another voice chat now, so can we do this a little later?
Sure :)
Thank you very much! We apologize for the inconvenience.
Ping me when you're available for debugging more please
I really hope this isn't a bug from Node.js 😅
I transferred the issue to /pieces and wrote a status message on it, @Lami
As a heads up :)
Thank you!
@kyra 🩵🩷🤍🩷🩵
It is possible now!
But I am very poor in English, so can we communicate by text while sharing the screen?
Sure :)
Alright, can you debug again until it reaches the same file? No need to repeat filter
Alright, now instead of the 2nd button, press the 3rd
Uhm... can you go to the built JS file and place a breakpoint there as well? Just in case
index.js?
In dist/commands/
Yes that one, just a breakpoint on line 2
Uhmmmmmmmmmm
Can you copy the path and do
require()
with it?
Somewhere in your index, to see if Node.js can load it
That won't quite work
You need \\
Breakpoint on line 15 just in case
And that'll be good to go :)
Ok, Node.js can't load the file at all
Lemme find a way to convert it to CP932 so we can load it much like the OS is expectingOkay!
Replace the name with
ヘル�
No no, in your terminal
You don't need to replace the file name or something
I'm re-encoding the string to see what can load it
I pretty much got those:
- ƒwƒ‹ƒv
- 荷莋荶�
- ヘル�
- 飣莫�
So I would like to try if any of the 4 options can load ヘルプ.js
You can do that in your terminal, no need to run VSCode's debugger for this :)
Uhm...https://github.com/nodejs/node/issues/55773
I did a little searching and found this issue on Node
Does it seem relevant?
GitHub
node.js responds nothing and even no error when executing *.js file...
Version v22.5.0 Platform Microsoft Windows NT 10.0.19045.0 x64 Microsoft Windows NT 10.0.22631.0 x64 Subsystem No response What steps will reproduce the bug? Run a cmd.exe. Run command mkdir "...
Yeah I saw, uhmmmmmmmmmmmm
It's starting to look like a Node.js bug :(
And it doesn't look like we can work around it by pre-recoding the path so when Node.js re-encodes it, it turns out fine
What Node.js version are you on? I saw 22. something
22.11.0!
Ok, I will try it!
But I don't think I have a version control tool installed, so it may take a bit of time to change the version!
I will try to install Volta.
You can try to install Volta later, that needs you to uninstall Node.js in the first place 😅
To test it quickly you can update to 22.13.0, see if it's fixed in that version
From what I'm seeing it's C++
std::filesystem::path
's fault, and latest versions remove it from Node.js's code
https://github.com/nodejs/node/issues/56049#issuecomment-2507881729
UhmmmmmmmmmmmmmmmmmmmmmmmmmI'll try 23.6.0.
s!ev const b = buffer.Buffer.from(
C:\\path\\to\\ヘルプ.js
, 'utf8');
const decoder = new util.TextDecoder('shift_jis');
[decoder.encoding, buffer.Buffer.from(decoder.decode(b), 'binary').toString('binary')];Output: ⏱ 427.64μs
Yeah... I don't think I can make this work :(, this looks like a Node.js bug
Okay, thank you for seeking a solution!
Just knowing the cause was helpful.
There are two workarounds available from what I have seen
Although I don't know what implications it has to switch that... probably wouldn't touch that without a backup
I'm curious whether Deno can run that :iara_think_thonk:
Sapphire supports Deno and Bun too, if Node.js can't, maybe you can try those
Ahhhhhh, I used to use UTF-8 in my system, but I am very unsure if I should activate that setting, as it caused enormous bugs in my old software.
Yeah... probably not a good idea, so we're left to the last workarounds of using either Deno or Bun and see if either can make it work
Okay, I'll try it!
Alright let's see if it loaded the command+
Progress made!
0 commands though :iara_think_thonk:
Why is it using require
Add
"type": "module"
in your package.json
Yeah that needs a rebuild... or run the TS code
It's trying to walk over the JS files
OH
package.json's main
Replace dist with src
Yes!!!
There we go!
Working!
:LenaEcstatic:
We finally did it
So it is indeed a Node.js bug
Thank you very much!
You tried with both Node.js v22.13.0 and 23.6.0, right?
Yes
I can file the issue for you if you want, and send you the link so you can subscribe to updates
i would like to subscribe
Thank you for letting me help you with this, it would have been much harder to find out the issue without the screen share :LenaSmile:
I'll prepare some food and then write the issue + send it here :)
Thank you very much! I am going to get some sleep now as it is already morning before I know it.
I will check the issue when I wake up!
Alright, have a nice sleep!
There it is :) https://github.com/nodejs/node/issues/56670?
GitHub
fs: can't require non-ASCII file paths in Shift-JIS filesystem · Is...
Version v22.13.0, v23.6.0 Platform Microsoft Windows NT 10.0.26100.0 x64 Subsystem fs What steps will reproduce the bug? Use Japanese Windows with Shift-JIS (aka CP932) Load a non-ASCII file path w...
Thank you!
I think we can pretty much close this thread? Not much we can do and nothing we have to do once Node fixes the problem.
If @Lami is fine with us marking this as "solved"/closing the thread, yeah
No problem!