Block an array of dates from Calendar
Hi everyone, this is my first time to the world of Nuxt. I'm here off the back of this tutorial;
https://www.youtube.com/watch?v=DvcHPR0CVpc
I've now learning about input Date, and have attempted the following to block specific dates from being selected. Max and Min works, and I can't pick dates as expected. But I'd also like to load an array of dates that are also blocked.
Keen to understand tutorial resources for this kind of question too.
John Komarnicki
YouTube
My Favorite Way To Build Forms With Validation In Nuxt
π Nuxt Course: https://www.learnnuxt.dev/
π Save 15% at checkout with code NUXT_UI
Video Resources
https://ui.nuxt.com/
https://zod.dev/
In this video, learn how to create a basic form with validation using Nuxt UI and Zod.
Other Resources
π Vue 3 Course: https://johnkomarnicki.gumroad.com/l/tqrmw
π€ Website: https://johnkomarnicki.com/
π¦ Twit...
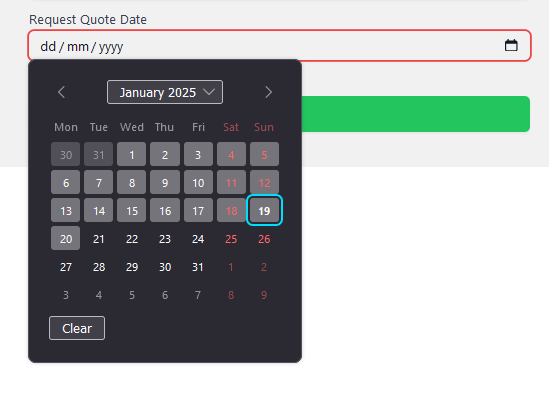
2 Replies
Hi @Karlstens. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the knowledge sources provided, I don't have specific information about blocking an array of dates in a Nuxt calendar component as you've described. However, I can provide some guidance based on the DatePicker component from Nuxt UI, which might be helpful for your use case.
The Nuxt UI library includes a DatePicker component that is built with v-calendar. While the exact implementation you're looking for isn't shown in the provided sources, the DatePicker component does offer customization options that might help you achieve what you want.
Here's an example of how you might use the DatePicker component from Nuxt UI:
In this example, we're using the
disabled-dates
prop of the DatePicker component to pass a function that checks if a date should be disabled. This function checks if the date is included in the blockedDates
array.
Please note that this is a simplified example and may need to be adjusted based on the exact implementation of the DatePicker component you're using.
For more detailed information and examples, you can refer to the Nuxt UI DatePicker documentation.
As for tutorial resources, the Nuxt documentation is generally a good place to start. However, for this specific use case with date pickers and blocking dates, you might need to look into the documentation of the specific date picker library you're using (like v-calendar in this case).
Remember that you'll need to install the necessary dependencies like v-calendar
and date-fns
as mentioned in the DatePicker installation section.
I hope this helps point you in the right direction. If you need more specific information, you might want to check the documentation of the date picker library you're using or ask a more targeted question about that library.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@501199901963583489> Kapa.ai is still learning and improving, please let me know how I did by reacting below.