How to update mysql server data?
Hi, I'm building todo list project server.
I wanna update database but have got 500 error.
30 Replies
What's the actual error?
Here is error.
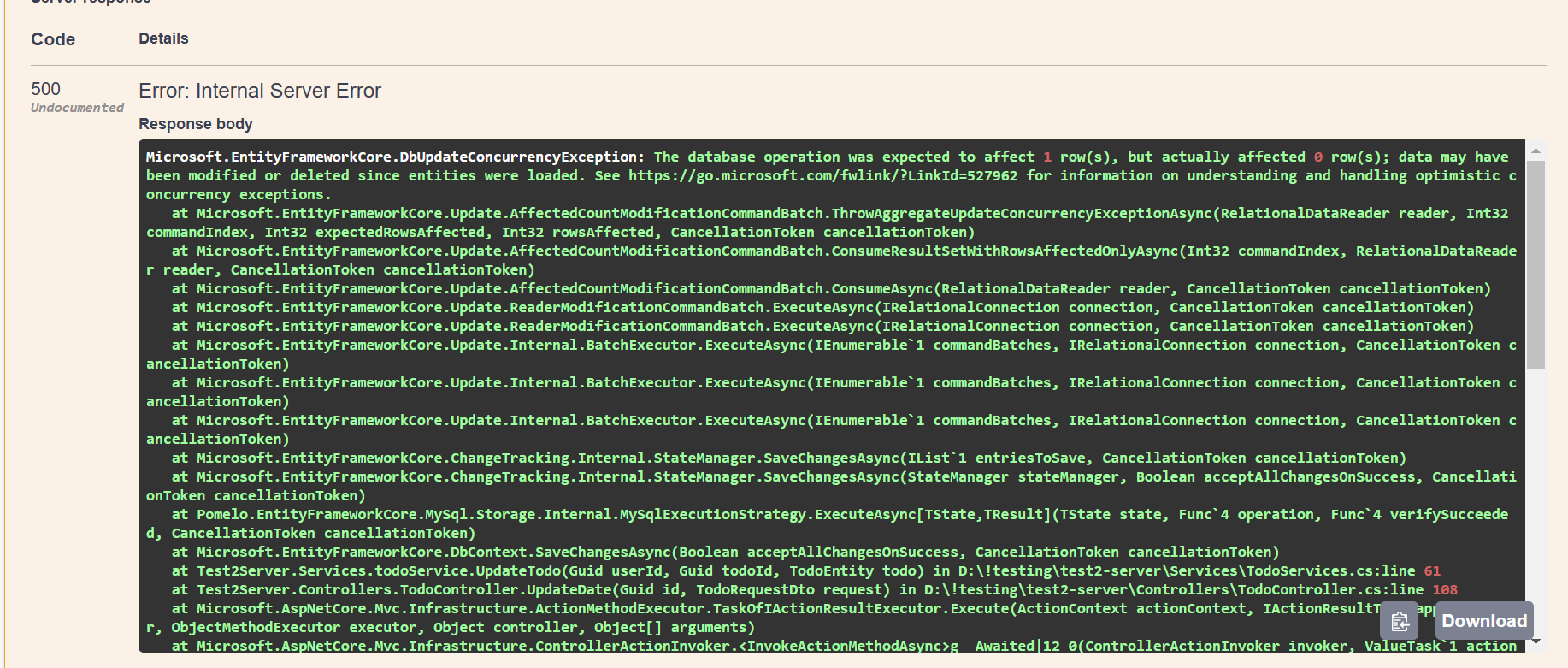
There's no need for that
_dbContext.todos.Update(existingTodo);
, and your controller doesn't use async methods where it should... but other than that everything seems fine
Place a breakpoint and step through the code, see where exactly it breaksIm beginner of C#. This is my first server.😅
So can you review my code?
On second look... it seems fine
Could be simplified a tad, I guess
For debugging tutorial, check $debug
Tutorial: Debug C# code and inspect data - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
It will teach you how to set breakpoints, step through code, etc
Thanks your help.
Also, show your
TodoEntity
I assume that's the database model
The error you got could pop up when it doesn't have a primary key set, IIRC
Config for that model too, if it's not in the attributes@Angius Sorry for the inconvenience.
But I have got also Internal Server Error.
If you don't mind, could you take a look at this code?
Yes, that's what error 500 is
GitHub
GitHub - SecretariatV/testing
Contribute to SecretariatV/testing development by creating an account on GitHub.
Sorry and thanks your help.
Huh, everything seems fine
oh, 😭
Thanks, 👍 😭
dbcontext has the relationship between TodoEntity and TodoItemEntity commented out, and that relationship is backwards, no? (it's been a while since I entity framework'd)
shouldnt it be
.Entity<TodoItemEntity>.WithMany(TodoItemEntity)
?I dont know how to use this code.
its from chatgpt.😅
🤔
😢
I had built todo list server using Express and now migrate ASP.NET.
you can paste the code back in chatgpt and tell it to explain it to you
chatgpt is 💩
can you review my code?
I did, and this is what you need to learn to fix it. You have to define the relationship between TodoEntity and TodoItemEntity
https://learn.microsoft.com/en-us/ef/core/modeling/relationships
Introduction to relationships - EF Core
How to configure relationships between entity types when using Entity Framework Core
Thanks
You don't have to. EF works by convention
Having a
List<Bar> Bars
property in Foo
, and Foo Foo
property in Bar
will create many-to-many for examplebut that's IF you have the navigation props in the entity types, which he doesn't.
Ah, then yeah
Didn't notice the missing props
neither did ChatGPT hehe.
LLMs being LLMs
this is why I'm not concerned about losing my job to AI.
Assuming the props were not altered post.