Need help inferring types of nested model
I'm new to drizzle, so obvious skill issue here, but how can I infer types from models with specific relations selected?
I know of
InferSelectModel
but I don't see a way to include relations.
Ideally I'd want to infer the type i get from something like this:
6 Replies
Have you specified relations?
yes I have
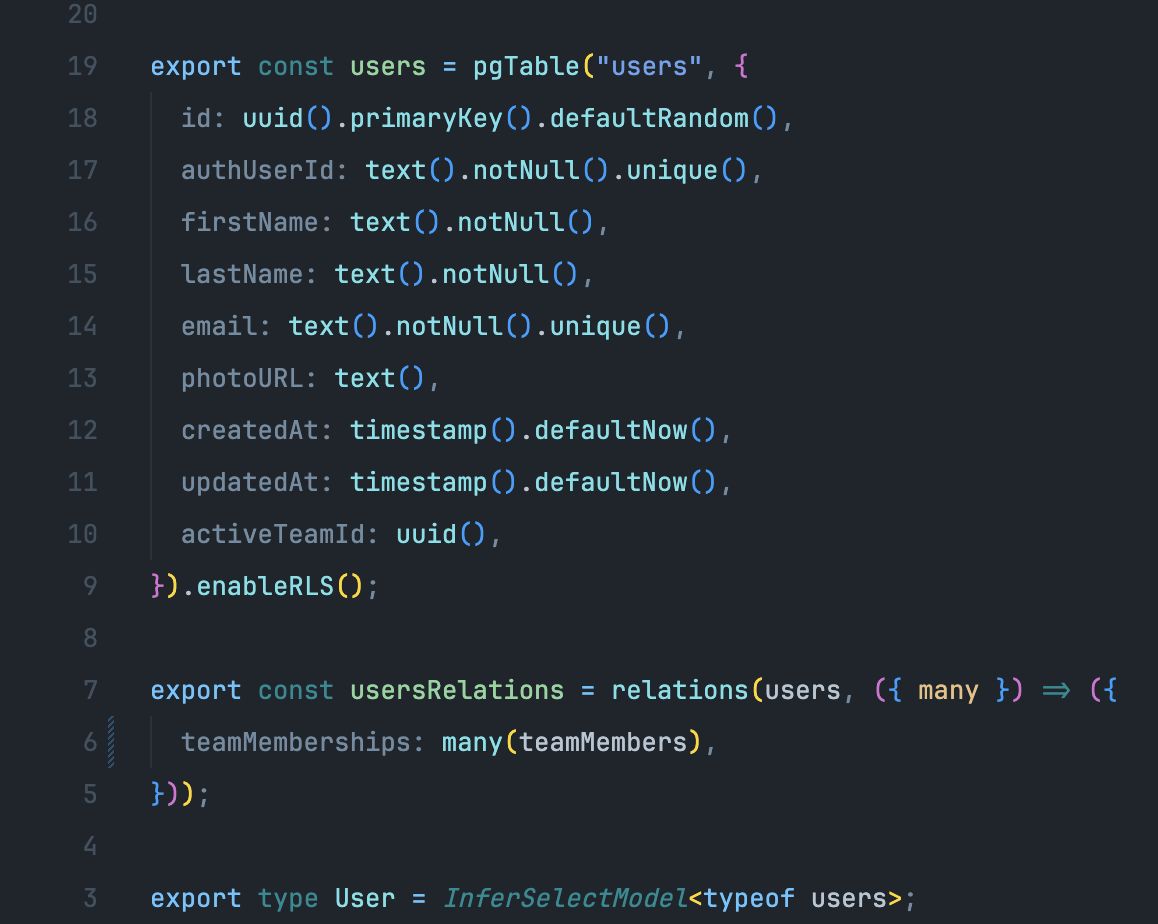
Is this that you mean?
GitHub
Implement infering table model with relations · Issue #695 · drizzl...
Prisma API: import { Prisma } from '@prisma/client' // 1: Define a type that includes the relation to
Post
const userWithPosts = Prisma.validator<Prisma.UserDefaultArgs>()({ include...Ohhh very interesting!
I'll try this
Thanks for the link as well :boneZone:
It works, thank you
I went with the expanded version.. allowing column inclusion/exclusion