How can edit the register account?
Hello friends, I am modifying the register a little, I want to save the plan relationship with the user but for some reason it does not take the onSubmit method, I don't know if this method is correct or not?
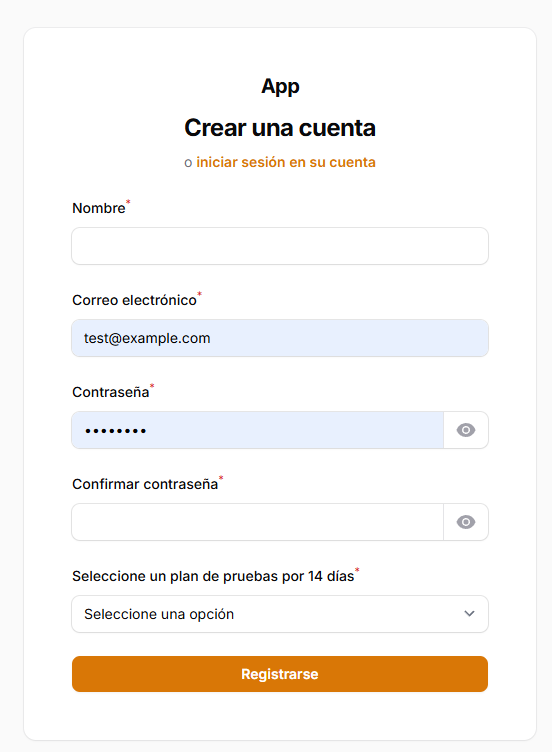
6 Replies
any idea guys?
Hi. You can use
protected function handleRegistration(array $data): Model
to manage you register process and not use onSubmit
(does not exist here).
For example :
You can also use relation on your form with the plan.what is the plan relationship?
here is the plan relationship in User Model
if you are using belongsToMany, your select should have multiple I think..
if you need to customize the creation process, use
Than you guys @LeandroFerreira and @Julien B. (aka yebor974) for the tips and answer...