Cannot read properties of undefined (reading 'referencedTable') for many-to-many
Hi Drizzle folks,
I'm new to drizzle and want to create a many-to-many relationship to model user and teams. It more or less the same as the many-to-many example in the documenation: https://orm.drizzle.team/docs/relations#many-to-many . Only difference is that I'm using UUIDs as primary key for both
user
and team
.
I've organized my schema into different files, created an index.ts
barel file and initialized Drizzle
Init of the drizzle config looks like this:
When I now do th following query in drizzle studio:
I get the error:
However the following works:
And also Drizzle Studio looks fine as far as I can tell.
Any idea what's going wrong here?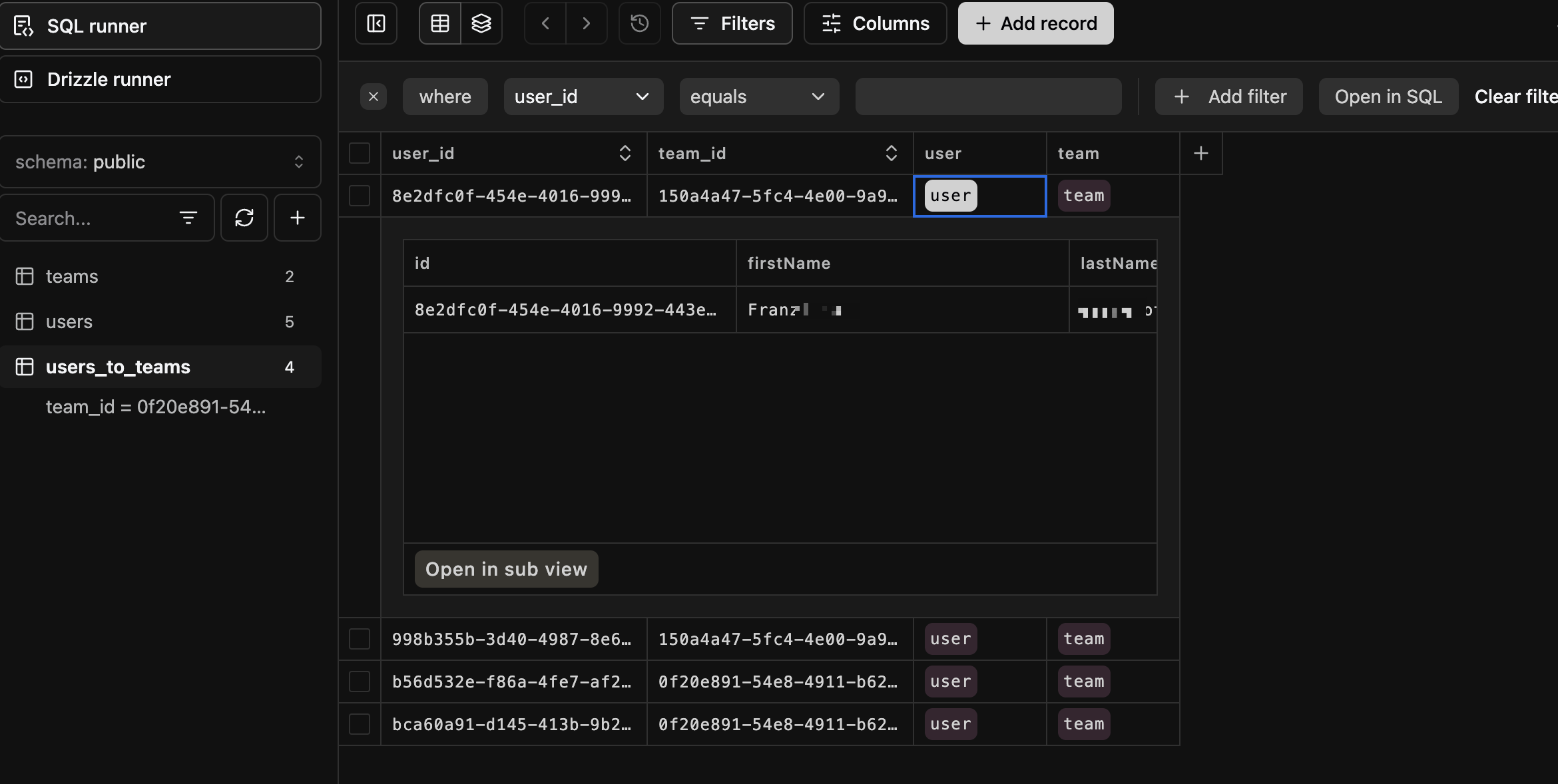
13 Replies
Can you send files where you defined tables and relations? These files to be specific:
(ping me when you reply)
Hey @Tenkes , sure. In my first version of the post I already had the content in the post but couldn't send it due to length limitations.
ans then when you want to loop through them you would do:
Hey @Tenkes . Tried it but same error π¦
Interesting, everything looks good... I'm only skeptical about the way you're exporting from your barrel file and about the fact you're default-exporting your tables when you're already exporting them. Maybe remove
export default
from all your schema files and then your barrel file should look something like:
@Andreas
Yeah that might be it. Or more precisely it might be because in your barrel file you're renaming default to user
instead of users
:
Same for teams. So either rename it or do what I said in previous message (I think it would be cleaner that way)Any further recommendations how I could track down the issue (besided form debugging the drizzle code π
)
Drizzle Run
Drizzle Run
Or try to reset stuff, clear cache, generate and migrate db etc...
Nice, I'll try that. Let's see if I can find the issue
@Tenkes Ok, that's kind of strange. I moved the example (with slight differences) to drizzle.run and it is working as expected https://drizzle.run/tjfaup6m3q9z8iys5o2bgf2k. One of the differences is, that I'm using a single schema file instead of multiple files. I'll try this now on my local machine.
Drizzle Run
undefined - Drizzle Run
Ok, never mind. I think its a bug in Drizzle Studio :(. I created a simple
testDB.ts
with
And I got the expected result. This is kind of annoying and I lost 3 hours :(.That really sucks, hope you at least figured it out