how to add inheritance between two c# files?
ive created tw c# files one called Pirate.cs and Gang.cs . I have John Adams in Gang.cs and i want pirate.cs to print it out but it doesnt work. could anyone help me find out the proper way to do inheritance in c# and how to get my code to work?
Pirate.cs
using System;
namespace PirateApp
{
class Pirate : Gang
{
public string Name { get; set; }
protected bool HasEyepatch { get; set; }
private DateTime Birthday { get; set; }
public Pirate(string name)
{
Name = name;
}
public void Swear(string[] swearWords)
{
foreach (var word in swearWords)
{
Console.WriteLine($"Arrr! {word}");
}
}
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine($"The name of the pirate is {Pirate.Name}");
}
}
}
Gamg.cs
namespace PirateApp
{
public class Gang
{
private string _territory = "Caribbean";
public string Name { get; set; } = "John Adams";
public int MemberCount { get; set; } = 30;
public string Territory
{
get => _territory;
protected set => _territory = value;
}
public Gang(string name, int memberCount, string territory)
{
Name = name;
MemberCount = memberCount;
_territory = territory;
}
public void UpdateTerritory(string newTerritory)
{
_territory = newTerritory;
}
}
}
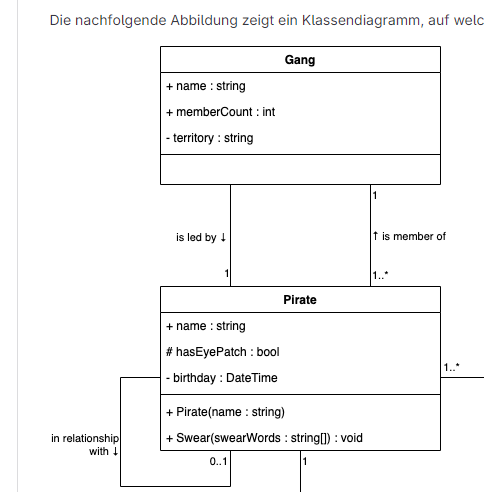
29 Replies
What "doesn't work?"
the data from gangs.cs i want it to be printed out in Pirate.cs but it doesnt work
class Pirate : Gang
means a pirate IS a gangInheritance is between classes, not files
Just so we're clear
you need to instantiate a
Pirate
for you to be able to print any information about a Pirate
also, your program will "start" in
Main
so the only thing it does atm is that console writeline
your Gang and Pirate classes are just that - class declarations
they dont actually do anything in your current code, as you dont use thoses classesand
Main
will of course not compilewhat i want from the console is it to write 'The name of the pirate is John Adams' the probelm is im not sure how can i make Pirate.cs get the value name from gang.cs and print it out.
I mean, first, stop focusing on the files and filenames
Is the pirate a whole gang?
Like, a gang of dwarves in a trench coat with a pirate hat?
"pirate.cs" is just a file, it doesnt do anything on its own. Just like your pirate class is just a class, it doesnt do anything on its own either
so it cant "get a value"
no its just a name
based on the UML you posted above, I think
Pirate
is supposed to be an individual pirate.just so you guys know im trying to implement this class uml from my school
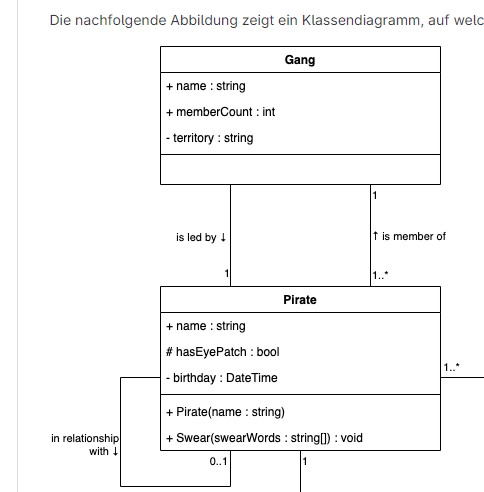
mhm
oh okay
there is no inheritance specified in that UML
and you have missed a few things, such as "is led by" and "is member of" etc
remember, inheritance is for x IS A y relationships
but doesnt the arrow mean in the uml that Pirate inherits from gang?
class
Goat : Animal
for example
no
it just shows a relationship
in this case, "is led by" would probably be represented by a public Pirate Leader {get; set;}
property
makes sense?kind of
so i would have to encapsulate the name in Pirate Leader and then send it to Pirate ?
?
lets take a step back
do you know how to create instances of a class?
yes
Can you show me how?
like this?Person person = new Person();
excellent
so, how do we make a pirate? maybe one who will be the leader of a gang?
also: $codegif
ok thanks now i understand
i just have one question how would i make this ?
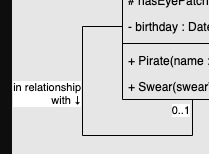
A pirate has an (optional) relationship to another Pirate