Table must not be accessed before initialization
Hello,
I'm encountering an issue with the dynamic visible function in my code. Whenever I select a value on input_1, I receive the following error:
Typed property App\Livewire\TagsManagerComponent::$table must not be accessed before initialization.
Here is an example of code to reproduce the problem:
TagsManagerComponent.php :
View :
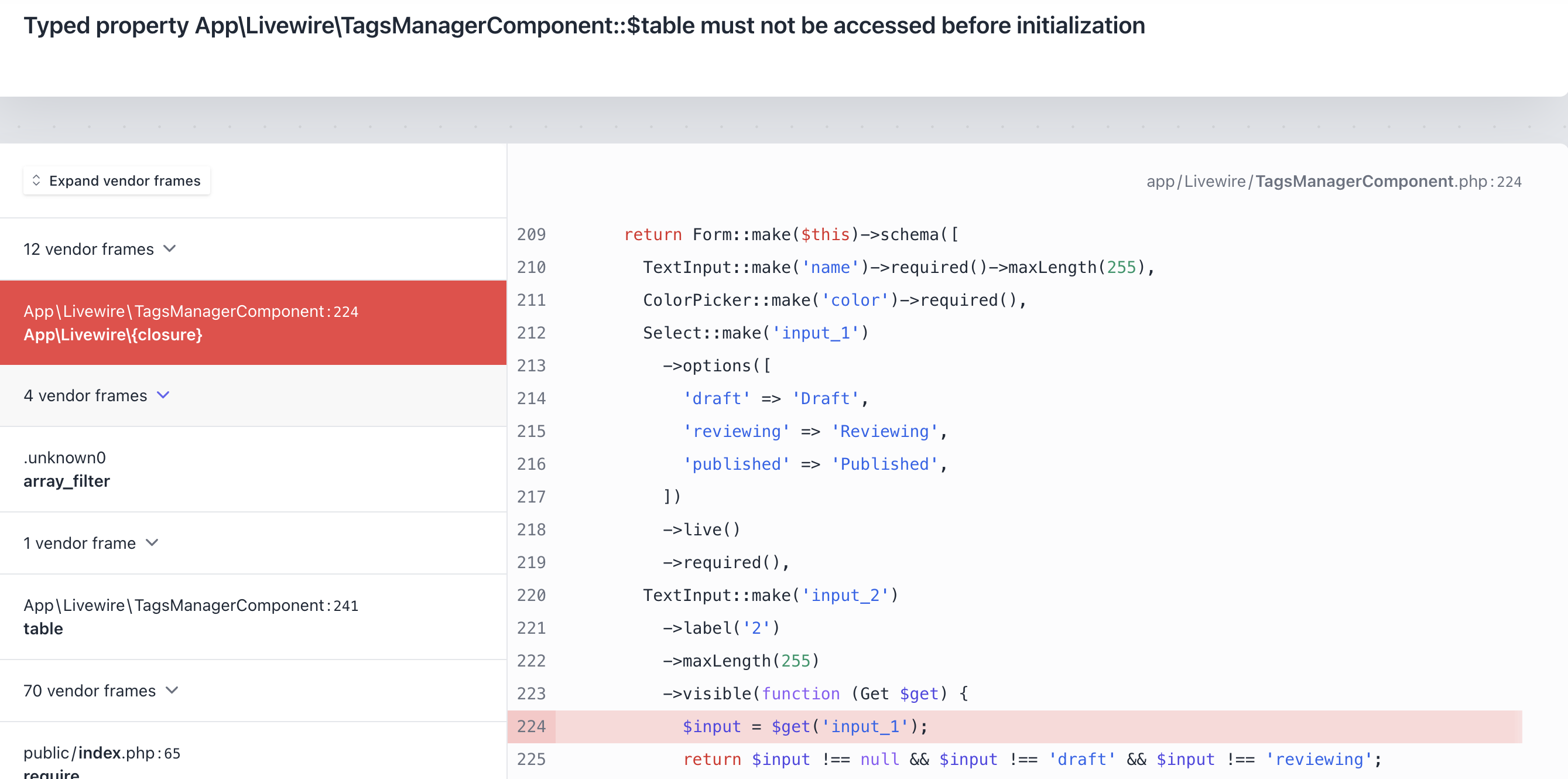
Solution:Jump to solution
likely has to do with
$this->makeForm()->getComponents()
which you call when you make your action
I'd refactor your makeForm()
method to just return an array of components
```php
private static function getFormComponents(?params): array...3 Replies
Solution
likely has to do with
$this->makeForm()->getComponents()
which you call when you make your action
I'd refactor your makeForm()
method to just return an array of components
you dont really need
$this
for your form,nor do you need the entire form for your action, just the schemaThank you ,it fixed the problem !