Issues with Middleware and Authentication in NextAuth.js with Drizzle and SQLite
Hi,
I'm working with NextAuth (Auth.js) and Drizzle for the first time. I've successfully implemented OAuth for Google and GitHub, and I added the DrizzleAdapter and schema by following the NextAuth documentation.
Now, I want to set up middleware to differentiate between protected and public routes based on whether the user is logged in. However, I'm running into a couple of issues:
1. The middleware check never seems to run.
2. !!auth?.user always returns false, even when the user is logged in.
I've tried using the
authorized
method in the callbacks within NextAuthConfig
. I’ve also watched a few YouTube videos, but for some reason, their approaches don't seem to work for me.
I’m not sure what I might be doing wrong. Any guidance would be greatly helpfull!
For context, I'm using SQLite with the better-sqlite3
library.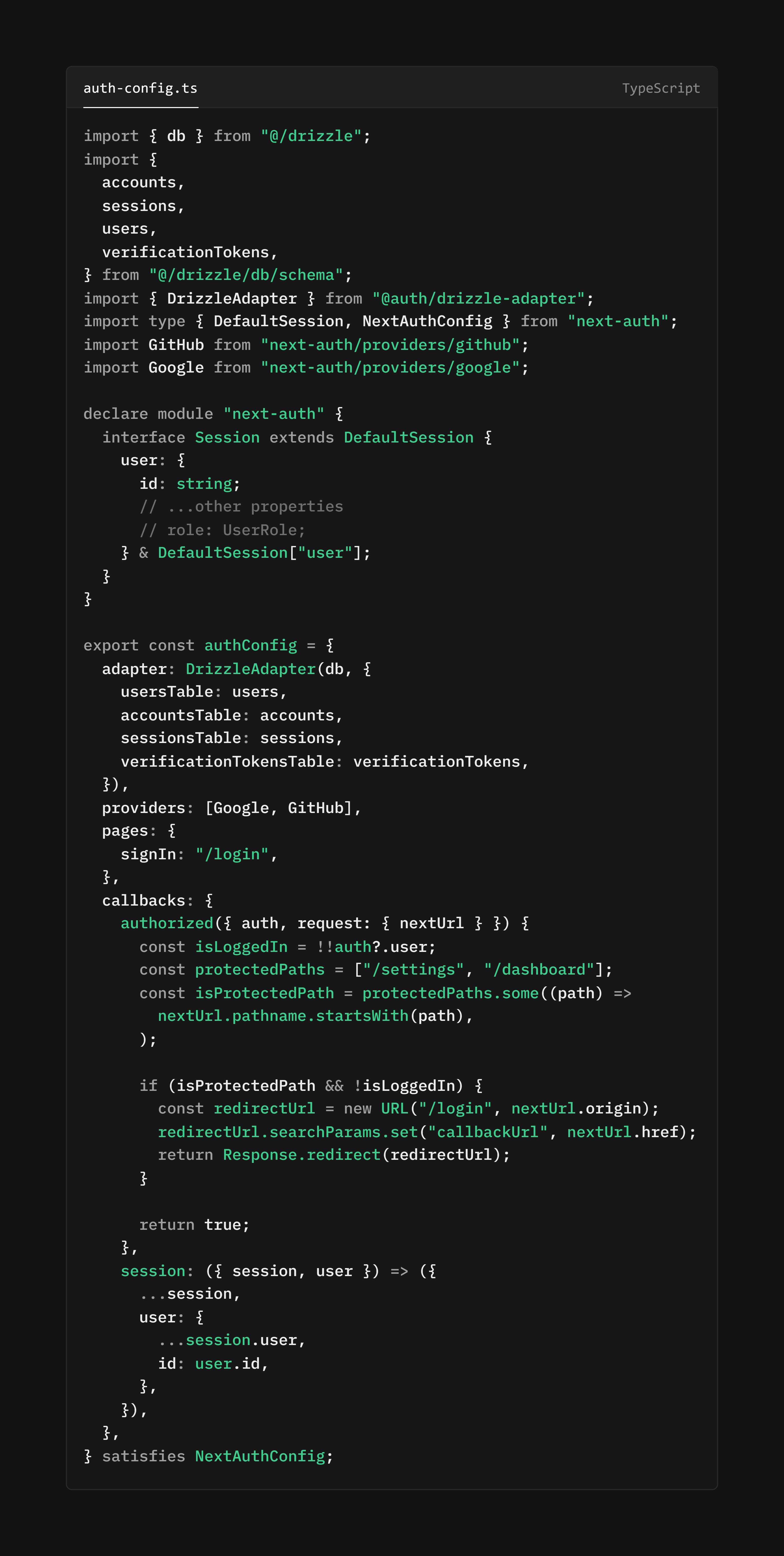
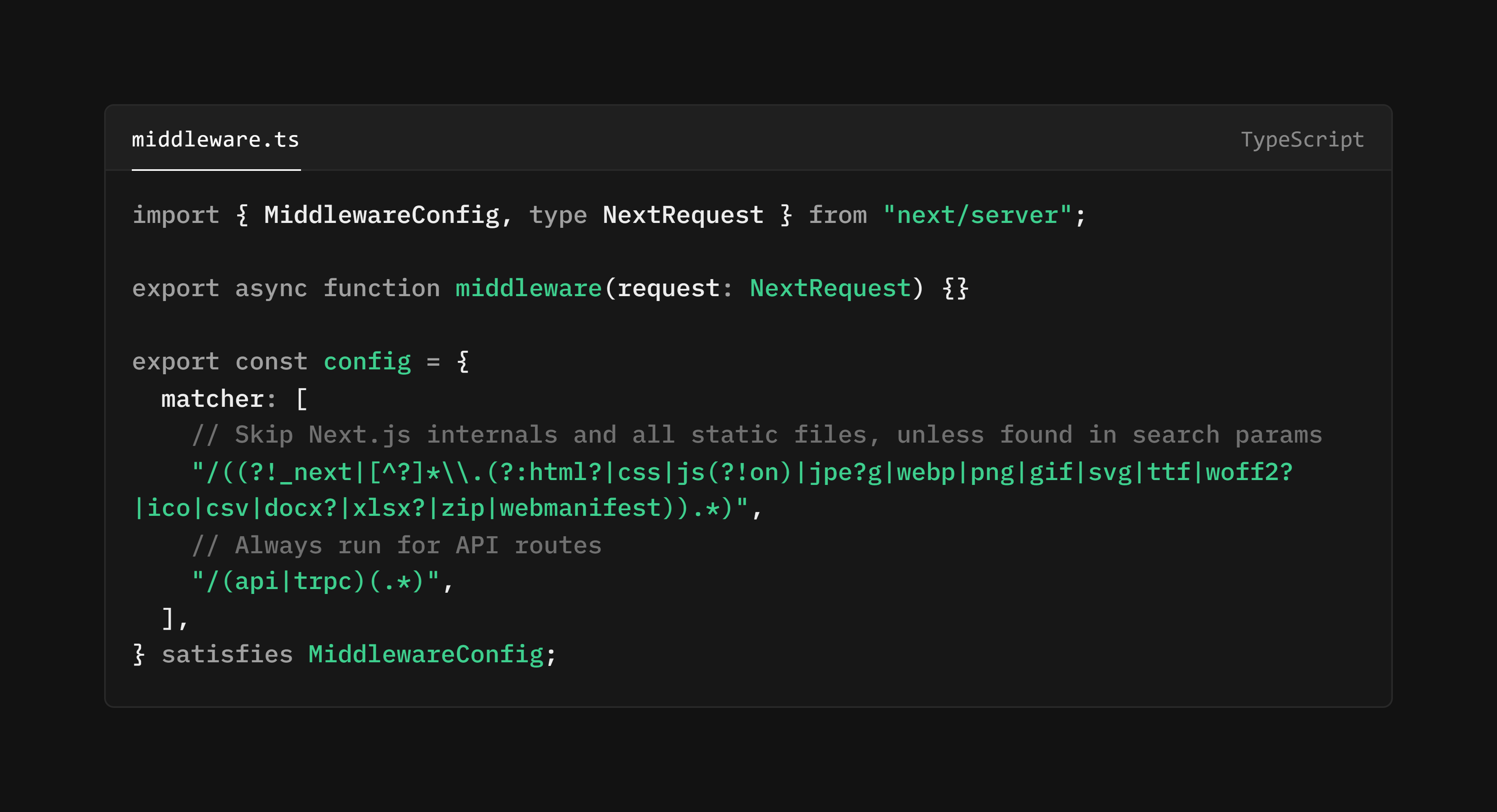
14 Replies
Auth.js | Protecting
Authentication for the Web
Tried this, always getting this error.
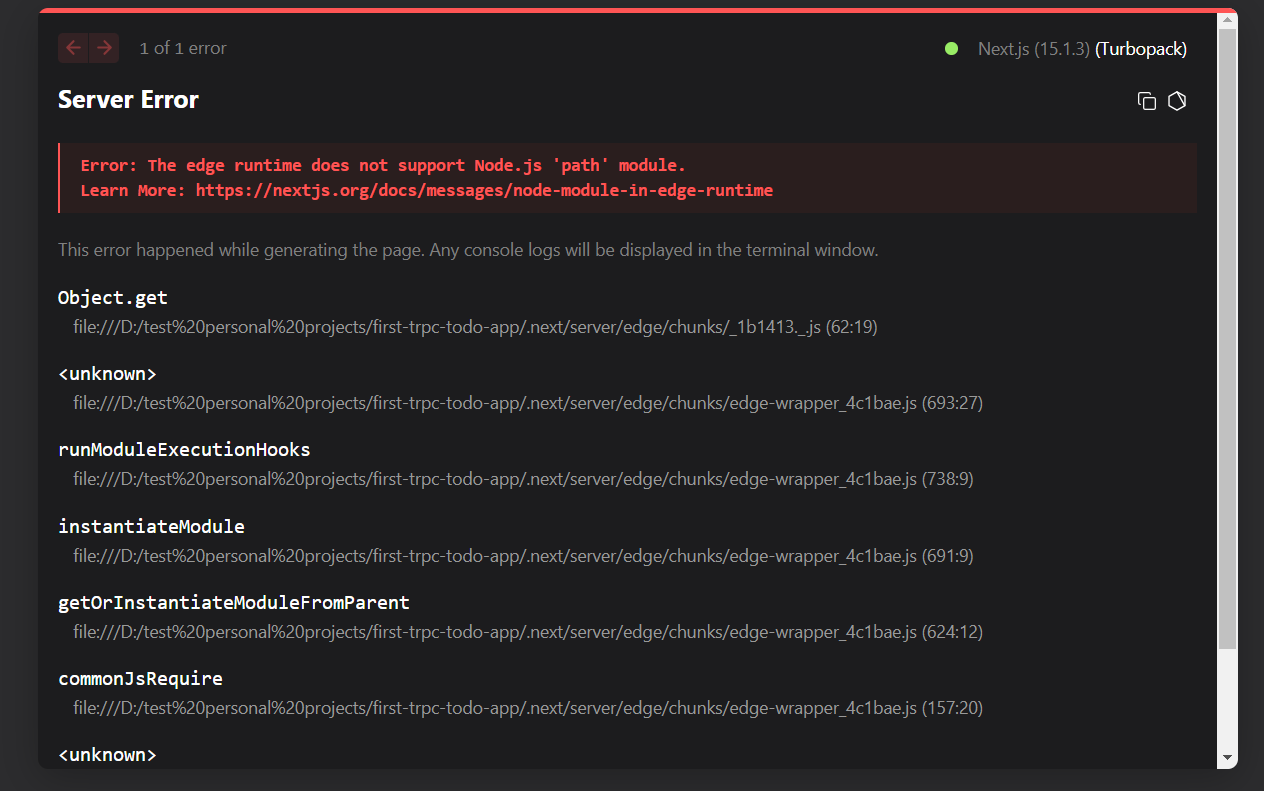
I have seperated the auth config and the main auth file.
Question: Is this because, I am using SQLite (better-sqlite3) driver, which seems to not be in edge compatible driver.
Drizzle ORM - Drizzle with Vercel Edge Functions
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
Need to see middleware.ts
Okay, I shifted from better-sqlite-3 to libSql from turso (which had edge compatible driver). Now that edge runtime error is resolved but no matter what how many times I login, it always redirect to
/login
route, and always return false for !!auth
.
middleware.ts
auth-config.ts
route.ts
removing runtime export don't seem to have any effectIs it just auth or is it auth.user?
just auth
I think, its working now
Cool. If not, I think I'd need a simple codesandbox recreation to help anymore.
Okay, it doesn't work. 🫠
For some reason, if we sign in for the first time, it works. However, if we sign out and then sign in with the same account, it doesn't do anything and returns false for !!auth?.user. Now, it doesn't work at all, not even the first time.
Codesandbox link
For some reason, installing dependencies in sandbox was failing continuesly.
here's the list of dependencies:
pnpm add @auth/drizzle-adapter @libsql/client drizzle-orm next-auth
What service are you using for db url and auth token? I need to get my own env vars
Although, after the last message, I went ahead and rewrote the entire schema and auth configuration from scratch. I dropped all the data from the database for a clean start, and now it's working exactly how I wanted it to.
I'm not entirely sure, but I think it was a schema issue since I was using database-based sessions and not cookies. Nonetheless, thank you for helping! ❤️
perfect no problem