NUXT3: CORS error
when i am using the $fetch method within handleCourse handler then the browser is giving the CORS error otherwise without handleCourse handler, if I use it openly it doesn't give any errors.
How to fix it?
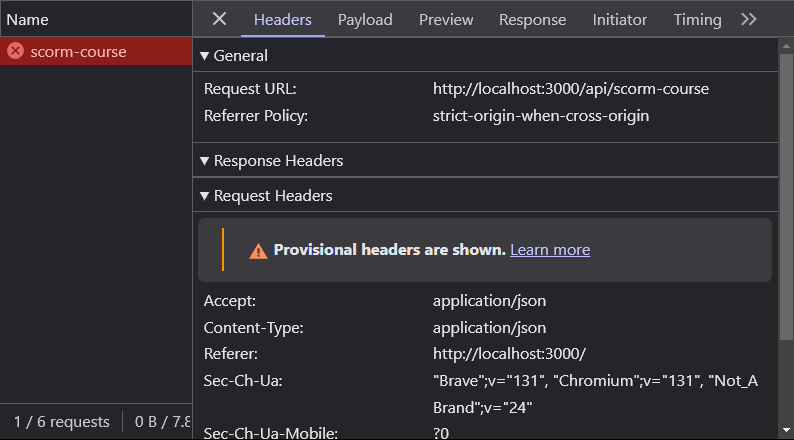
8 Replies
Hi @Azaan. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To address the CORS error you're encountering when using
$fetch
within the handleCourse
handler in Nuxt 3, there are a few potential solutions:
1. Server-side fetching:
The most recommended approach is to make the API call on the server-side using useAsyncData
or useFetch
. This avoids CORS issues altogether as the request is made from your server, not the client's browser.
Nuxt 3 Data Fetching (https://nuxt.com/docs/getting-started/data-fetching) provides more details on this approach.
2. Proxy the request:
If you must use $fetch
on the client-side, you can set up a proxy in your Nuxt configuration to route the request through your server. This way, the browser makes a request to your own domain, avoiding CORS issues.
In your nuxt.config.ts
:
Then use $fetch('/api/your-endpoint')
in your code.
3. CORS configuration on the API:
If you have control over the API, you can configure it to allow CORS requests from your Nuxt application's domain. However, this might not be possible if you're using a third-party API.
Remember, using $fetch
directly in client-side code can lead to issues like the one you're experiencing. It's generally better to use useAsyncData
or useFetch
for data fetching in Nuxt 3, as they handle both server-side and client-side scenarios more efficiently.
Nuxt 3 $fetch documentation (https://nuxt.com/docs/api/utils/dollarfetch) provides more information on the proper use of $fetch
.
If these solutions don't resolve your issue, you may need to provide more details about your specific setup and the API you're trying to access.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1041598174751621173> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai
And here is my frontend code:
<script setup lang="ts">
import { ref } from 'vue';
import type { Course } from './scorm/scorm-course-zod-schema';
const course = ref<Course | null>(null);
const error = ref<string | null>(null);
const isLoading = ref(false);
const handleCourse = async () => {
try {
isLoading.value = true;
error.value = null;
const { data, error: fetchError } = await useFetch<Course>('/api/scorm-course', { method: 'POST', body: { input: "I want a very good course to train my employees about prospecting" } }); if (fetchError.value) { throw new Error(fetchError.value.message); } course.value = data.value; } catch (err) { error.value = err instanceof Error ? err.message : 'An error occurred'; console.error('Error:', err); } finally { isLoading.value = false; } } </script> <template> <div class="bg-gray-900 h-screen flex items-center justify-center flex-col text-white gap-6"> <h1 class="text-3xl text-white">Course Generator</h1> <button @click="handleCourse" :disabled="isLoading" class="bg-blue-500 px-4 py-2 rounded hover:bg-blue-600 disabled:opacity-50" > {{ isLoading ? 'Generating...' : 'Generate Course' }} </button>
<div v-if="error" class="text-red-500 p-4 bg-red-900/50 rounded"> {{ error }} </div>
<div v-if="course" class="max-w-2xl"> <pre class="whitespace-pre-wrap">{{ JSON.stringify(course, null, 2) }}</pre> </div> </div> </template> tell me how can i fix that cors error
const { data, error: fetchError } = await useFetch<Course>('/api/scorm-course', { method: 'POST', body: { input: "I want a very good course to train my employees about prospecting" } }); if (fetchError.value) { throw new Error(fetchError.value.message); } course.value = data.value; } catch (err) { error.value = err instanceof Error ? err.message : 'An error occurred'; console.error('Error:', err); } finally { isLoading.value = false; } } </script> <template> <div class="bg-gray-900 h-screen flex items-center justify-center flex-col text-white gap-6"> <h1 class="text-3xl text-white">Course Generator</h1> <button @click="handleCourse" :disabled="isLoading" class="bg-blue-500 px-4 py-2 rounded hover:bg-blue-600 disabled:opacity-50" > {{ isLoading ? 'Generating...' : 'Generate Course' }} </button>
<div v-if="error" class="text-red-500 p-4 bg-red-900/50 rounded"> {{ error }} </div>
<div v-if="course" class="max-w-2xl"> <pre class="whitespace-pre-wrap">{{ JSON.stringify(course, null, 2) }}</pre> </div> </div> </template> tell me how can i fix that cors error
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the code you've provided and the context of your question, it appears that you're already using
useFetch
in your Nuxt 3 application, which is the recommended approach for making API requests. The CORS error you're experiencing is likely not due to the frontend code, but rather a server-side configuration issue.
Here are a few steps you can take to address the CORS error:
1. Server-side handling:
If the /api/scorm-course
endpoint is part of your Nuxt application, you shouldn't encounter CORS issues. Make sure your server-side API route is correctly set up in the server/api
directory of your Nuxt project.
2. Proxy configuration:
If the API is on a different domain, you can set up a proxy in your Nuxt configuration. Add the following to your nuxt.config.ts
:
Replace '<https://your-api-domain.com'
> with the actual URL of your API. This will proxy requests to /api/*
through your Nuxt server, avoiding CORS issues.
3. API CORS configuration:
If you have control over the API server, ensure it's configured to allow CORS requests from your Nuxt application's domain. The exact configuration depends on your backend framework.
4. Error handling:
Your current error handling looks good, but you might want to log the full error object for debugging:
5. Check network requests:
Use your browser's developer tools to inspect the network requests. Look for any preflight OPTIONS requests and their responses to get more information about the CORS issue.
6. Ensure correct API URL:
Double-check that the API URL in your useFetch
call is correct. If you're using a relative URL (like '/api/scorm-course'), make sure it's pointing to the right place.
If you've implemented these suggestions and are still encountering CORS errors, you may need to provide more information about your backend setup and the exact error message you're receiving.
Remember, CORS errors are security features implemented by browsers, and the solution often involves proper server-side configuration rather than changes to the frontend code.
Stack Overflow: CORS error solution (https://stackoverflow.com/questions/78633717) provides additional context on handling CORS errors in Nuxt applications.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1041598174751621173> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai how to use useAsyncData instead of $fetch here:
const handleCourse = async () => {
try {
isLoading.value = true;
error.value = null;
const { data, error: fetchError } = await useFetch<Course>('/api/scorm-course', { method: 'POST', body: { input: "I want a very good course to train my employees about prospecting" } }); if (fetchError.value) { throw new Error(fetchError.value.message); } course.value = data.value; } catch (err) { error.value = err instanceof Error ? err.message : 'An error occurred'; console.error('Error:', err); } finally { isLoading.value = false; } }
const { data, error: fetchError } = await useFetch<Course>('/api/scorm-course', { method: 'POST', body: { input: "I want a very good course to train my employees about prospecting" } }); if (fetchError.value) { throw new Error(fetchError.value.message); } course.value = data.value; } catch (err) { error.value = err instanceof Error ? err.message : 'An error occurred'; console.error('Error:', err); } finally { isLoading.value = false; } }
Let me check the knowledge base for you again. One moment! :books:
----------------------
To use
useAsyncData
instead of useFetch
in your code, you can modify the handleCourse
function as follows:
Here's what changed:
1. We replaced useFetch
with useAsyncData
.
2. useAsyncData
takes two arguments:
- A unique key for caching ('course' in this case)
- A function that returns the fetch promise
The $fetch
function is used inside the second argument of useAsyncData
to make the actual API call.
This approach using useAsyncData
provides more control over caching and can be beneficial for server-side rendering scenarios. It's important to note that useAsyncData
is typically used at the top level of a component setup function for automatic server-side rendering. In this case, since it's inside an event handler, it will only run when the function is called.
For more information on using useAsyncData
, you can refer to the Nuxt 3 useAsyncData documentation (https://nuxt.com/docs/api/composables/use-async-data).
Remember, if you're calling this function in response to user interaction, you might want to consider using $fetch
directly or useLazyAsyncData
instead, as useAsyncData
is primarily designed for data that needs to be available immediately when the component loads.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1041598174751621173> Kapa.ai is still learning and improving, please let me know how I did by reacting below.