Why is it when I refresh the page I get api calls error?
This is the code on me
dashboard.vue
/composables/auth.ts
39 Replies
Hi @o.m. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the information provided in your question and the knowledge sources available, it appears that you're experiencing an issue with API calls when refreshing the page in a Nuxt application. This problem is likely related to how data fetching and caching work in Nuxt 3. Let me explain the possible reasons and suggest some solutions:
1. Caching behavior:
By default, Nuxt caches the results of data fetching composables like
useFetch
and useAsyncData
. When you refresh the page, Nuxt might be using the cached data instead of making a new API call. This can lead to errors if the cached data is stale or if the API is expecting fresh authentication.
2. Server-side rendering (SSR) vs. Client-side rendering:
The error might occur because the API call is being made differently on the server-side compared to the client-side, especially if you're using authentication headers that are not available during SSR.
To address these issues, you can try the following solutions:
1. Use useFetch
with a unique key:
Instead of using $fetch
directly, wrap your API call in useFetch
and provide a unique key. This will help Nuxt manage the caching more effectively:
2. Disable caching:
If you always want fresh data, you can disable caching by setting cache: false
in the useFetch
options:
3. Use refreshNuxtData
:
If you need to manually refresh the data, you can use the refreshNuxtData
utility:
4. Handle server-side vs. client-side:
Ensure that your sessionHeader
is available on both server and client. You might need to use useRequestHeaders
to properly handle headers during SSR:
5. Use useLazyFetch
:
If you want to fetch data only on the client-side, you can use useLazyFetch
:
These suggestions are based on the information provided in the Nuxt 3 Data Fetching documentation (https://nuxt.com/docs/getting-started/data-fetching) and useFetch documentation (https://nuxt.com/docs/api/composables/use-fetch).
Remember to handle loading and error states appropriately in your component when using these data fetching methods.
If you're still experiencing issues after trying these solutions, you might need to provide more details about the specific errors you're encountering and the version of Nuxt you're using.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@674984670156423171> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai this is the error I get
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the error message you've provided, it seems that the API endpoint
/api/auth/user
is returning a 404 "User not found" error. This suggests that the issue is not directly related to Nuxt's data fetching or caching mechanisms, but rather to the API itself or the authentication state. Let's explore some possible reasons and solutions:
1. Authentication State:
The most likely cause is that the user's authentication state is not being properly maintained or passed to the API. This could happen if:
- The user's session has expired
- The authentication token is not being sent correctly in the request headers
- The server-side session management is not working as expected
2. API Route Implementation:
There might be an issue with how the /api/auth/user
route is implemented on your server.
To address this issue, you can try the following:
1. Check Authentication Headers:
Ensure that you're sending the correct authentication headers with your request. You can log the headers to verify:
2. Verify Session State:
Make sure your session is still valid. You might need to implement a session refresh mechanism if it's expiring too quickly.
3. Handle Unauthenticated State:
In your getCurrentUser
function, add logic to handle the 404 error specifically:
4. Check API Implementation:
Verify that your server-side API route for /api/auth/user
is correctly implemented and is able to identify the user based on the session or token you're sending.
5. Use Error Handling in Component:
In your dashboard.vue
, you can add error handling:
6. Implement Auth Middleware:
Consider using Nuxt middleware to check authentication status before rendering pages that require authentication. This can help prevent the 404 errors by redirecting unauthenticated users:
Then apply this middleware to your dashboard or protected routes.
Remember, the 404 "User not found" error is coming from your API, not Nuxt itself. So, it's crucial to ensure that your backend authentication logic is working correctly and that you're sending the proper authentication credentials with each request.
If you continue to face issues after trying these solutions, you may need to investigate your server-side code and authentication mechanisms more deeply.
Nuxt Authentication (https://nuxt.com/docs/getting-started/authentication) documentation might provide additional insights into setting up authentication in your Nuxt 3 application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@674984670156423171> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai the error happens on the server side not on the network logs or console of dev tools
Let me check the knowledge base for you again. One moment! :books:
----------------------
Thank you for providing that additional information. It's important to understand that the error is occurring on the server side rather than in the browser. This changes our approach to troubleshooting. Let's focus on server-side issues in Nuxt.
Based on the knowledge sources available, here are some potential reasons and solutions for your server-side error:
1. Server-side rendering (SSR) issues:
The error might be occurring during the server-side rendering process. Nuxt performs initial data fetching on the server, which could be causing this issue.
2. Middleware execution:
If you have any server-side middleware, it might be interfering with the request or not properly handling authentication.
3. API route implementation:
The problem could be in how your API route for
/api/auth/user
is implemented on the server.
Here are some steps you can take to address this:
1. Use setResponseStatus
to handle the error:
In your API route or server middleware, you can use setResponseStatus
to properly set the error status. This will help Nuxt handle the error correctly during SSR.
This approach is documented in the H3 Utils documentation (https://h3.unjs.io/utils/response#setresponsestatusevent-code-text).
2. Implement error handling in your API route:
Make sure your API route is properly handling cases where the user might not be authenticated or found.
3. Use createError
for better error handling:
Nuxt provides a createError
utility that can be used to create errors with additional metadata. This can be helpful for debugging and handling errors consistently across your application.
This approach is documented in the Nuxt Error Handling documentation (https://nuxt.com/docs/api/utils/create-error).
4. Check your server middleware:
If you have any server middleware, ensure it's not interfering with the authentication process. You might need to add some logging or debugging to your middleware to see if it's being executed as expected.
5. Verify server-side environment:
Make sure your server has access to all necessary environment variables or configuration needed for authentication.
6. Use Nuxt's built-in error handling:
Nuxt provides a way to handle errors globally. You can create an error.vue
file in your project root to handle all errors, including 404s:
This is described in the Nuxt Error Handling documentation (https://nuxt.com/docs/getting-started/error-handling).
Remember, server-side errors in Nuxt can be tricky to debug. You might need to add more logging on the server side to get a clearer picture of what's happening during the request lifecycle. If you continue to face issues, you may need to provide more details about your server setup and any custom server-side code you're using.
Stack Overflow: Nuxt.js localhost redirects to /login automatically, resulting in 404 error (https://stackoverflow.com/questions/78746436) suggests that in some cases, this behavior might be caused by default settings in modules like Supabase. If you're using any authentication modules, make sure to check their configuration as well.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@674984670156423171> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
I do not understand what is the cause of this weird behavior when I only called this api once but for some reason I first get empty values then later the logs have values?
On the backend I get this error
First the value is null then it returns a value
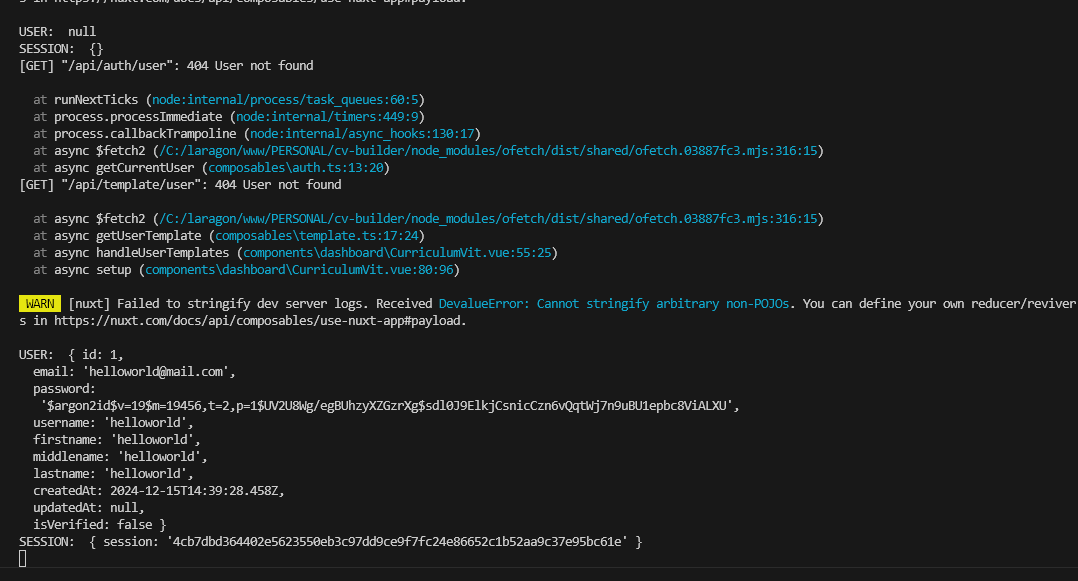
@kapa.ai whats the cause this issue ?
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the information provided in our conversation history and the available knowledge sources, there are several potential causes for the server-side 404 "User not found" error you're experiencing. However, without more specific details about your server-side implementation, it's challenging to pinpoint the exact cause. Here are the most likely reasons:
1. Authentication Issues:
The error suggests that the server is unable to find or authenticate the user. This could be due to:
- Expired or invalid session tokens
- Incorrect handling of authentication headers on the server
- Issues with server-side session management
2. API Route Implementation:
There might be a problem with how the
/api/auth/user
route is implemented on your server. The route might be failing to correctly identify or retrieve the user based on the provided authentication information.
3. Middleware Interference:
If you have server-side middleware in place, it could be interfering with the authentication process or not properly passing authentication information to your API routes.
4. SSR (Server-Side Rendering) Complications:
The error occurs during server-side rendering, which suggests that the initial data fetching on the server is failing. This could be due to differences in how authentication is handled during SSR compared to client-side rendering.
5. Environment Configuration:
There might be missing or incorrect environment variables on the server that are necessary for authentication.
6. Module Configuration:
As mentioned in the Stack Overflow post about Nuxt redirects (https://stackoverflow.com/questions/78746436), some authentication modules (like Supabase) have default behaviors that can cause unexpected redirects or 404 errors. If you're using any authentication modules, their configuration might be contributing to this issue.
To determine the exact cause, you would need to add more detailed logging on the server-side, particularly in your authentication logic and API route handlers. This would help trace the exact point where the user authentication is failing.
Remember, this error is coming from your server-side code, not from Nuxt itself. To resolve it, you'll need to focus on debugging your server-side authentication implementation and API routes.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@674984670156423171> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
$fetch request don't take context of your request on server side
Use useRequestFetch()('/api')
Nuxt
useRequestFetch · Nuxt Composables
Forward the request context and headers for server-side fetch requests with the useRequestFetch composable.
And you have 2 requests for same endpoint?
First on server and second on client?
I only have one request for 1 endpoint
There are so many api call function use cases its making me crazy
But how you get 2 outputs in console?,
Xd
I dont know. I only called it once here
Looks like 1 request made on server and second on client
Show me the code again
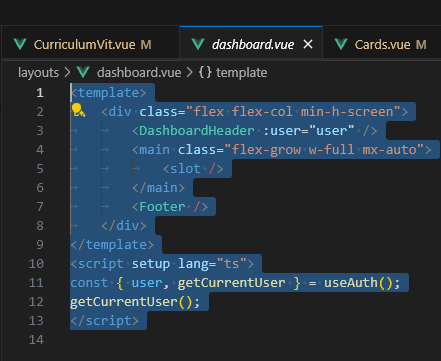
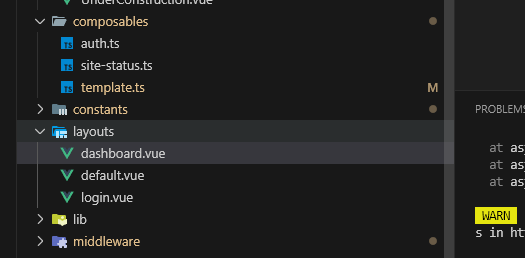
so basically I only ran this one once
You better to use it inside a plugin
Like getting user
https://discord.com/channels/473401852243869706/1318526910703075399/1318532786914590751
Second link
It's a bit complex but you need to get the idea
I'm trying to get the idea
Do I have to do it like this ?
export default defineNuxtPlugin(async (nuxtApp) => {
my useAuth()
is basically jsut a composableInside a plugins folder
Yes
Yes
You leave your composable as it is
Then create a plugin
Nuxt
plugins/ · Nuxt Directory Structure
Nuxt has a plugins system to use Vue plugins and more at the creation of your Vue application.
And use your composable here
To get the user
From server
And use user object here
Whats the purpose of plugin?
But without get current user
to initialize the user?
I've send you the docs
Yes
Not sure if this is the right approach but here is what I did
/layouts/dashboard.vue
I get hydration error after the implementation
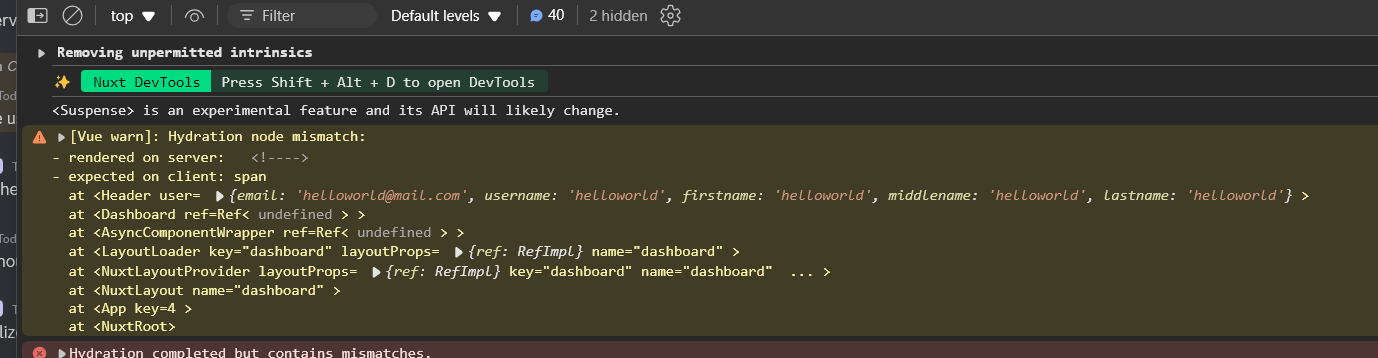
The
const requestFetch = useRequestFetch();
really solved the backend errors
Thanks a lot
You are life savior. Thank you very much for your guidance!Don't need to check it just call the method
You can also call that composable inside a dashboard header component
It's still there?
the hydration error is fixed
This seems to fix the issue
const requestFetch = useRequestFetch();
yea
Plugin runs in the client side ?
both