Running into some type errors with query.where
I have a massive query that grabs rows from a table called
transfer_portal_entries
and joins them with a couple other tables to get the players information back that is needed to populate a page. However, when it comes to the if statements around the filters, query
is underlined saying
However, I am not entirely sure how to resolve this.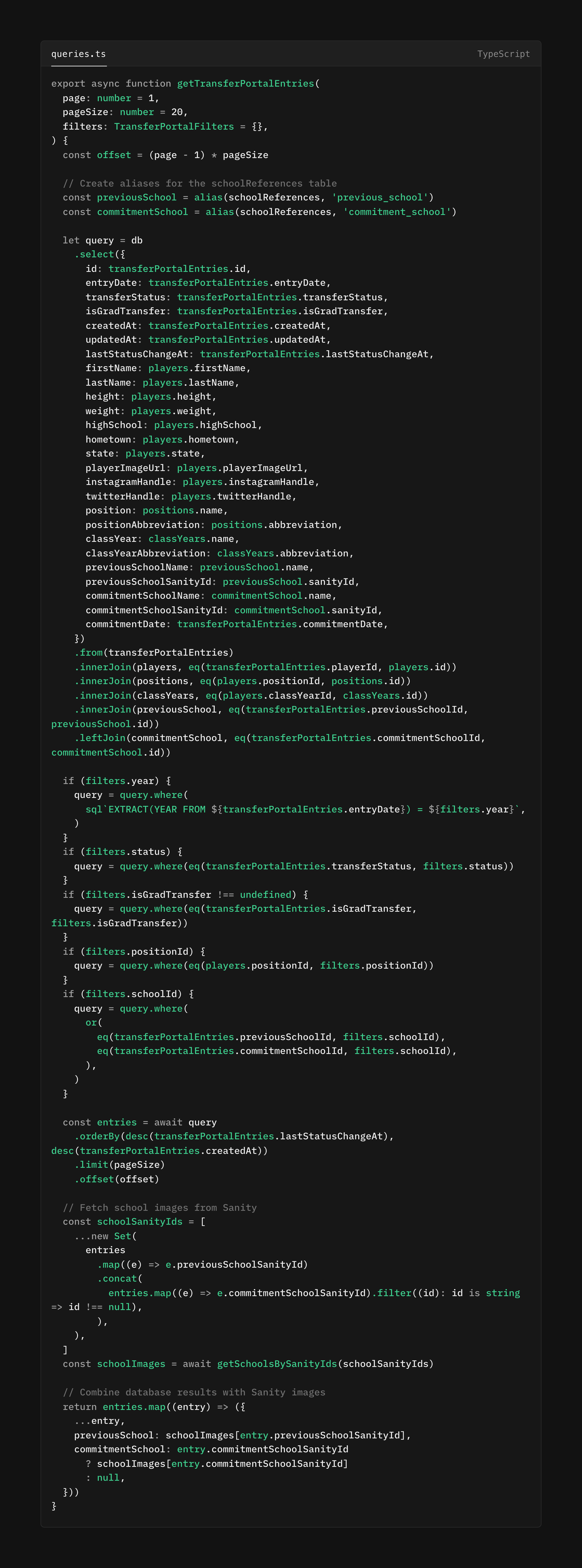
7 Replies
Here's how you solve this:
1. Create an empty array like this:
2. Add your filters before building the query by doing
filters.push
.
3. Spread the array in the where
method of the query (.where(...filters)
)Is that before building the original query?
Yes, before building
query
Here are some docs that go in a bit more detail:
https://orm.drizzle.team/docs/guides/conditional-filters-in-query
Drizzle ORM - Conditional filters in query
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
Odd, I did
And nothing returns, if I remove the
.where()
it returns data. The current filters are { filters: { year: 2025, status: 'All' } }
being passed in.
I also have an issue where status: 'All'
won't return becuase of
So need to figure that out as wellIt may have to do with the logic rather than being something syntactical
Have you pushed the latest changes to your DB?
Yea I have done
drizzle-kit push
for my schemas or else the original query minus the .where()
wouldn't return anything. It returns data if I remove the .where()
So I think it's something with
Added a different filter and that works just fine
And that schema for transferPortalEntries
looks like
So there is an entryDate