ImportAction filling relational data from multiple columns
HI, I am making an importer for standard company table of my projects.
Each company has an invoice and regular address.
I saw the resolveUsing but this only allows you to resolve using the state of one field. For address relation I need to check for multiple fields.
I tried resolving the following:
However this still tries to insert all the ImportColumns into company, even street, number etc... while these fields are not in my company model. Am I missing something here? I thought reading the docs very carefully that this was the way to go. I inserted a screenshot with my import columns.
In my log file it tries to insert all the data found and returns the following update:
Clearly mixing the address table and company table. Does anyone have something like this working? Thanks in advance!
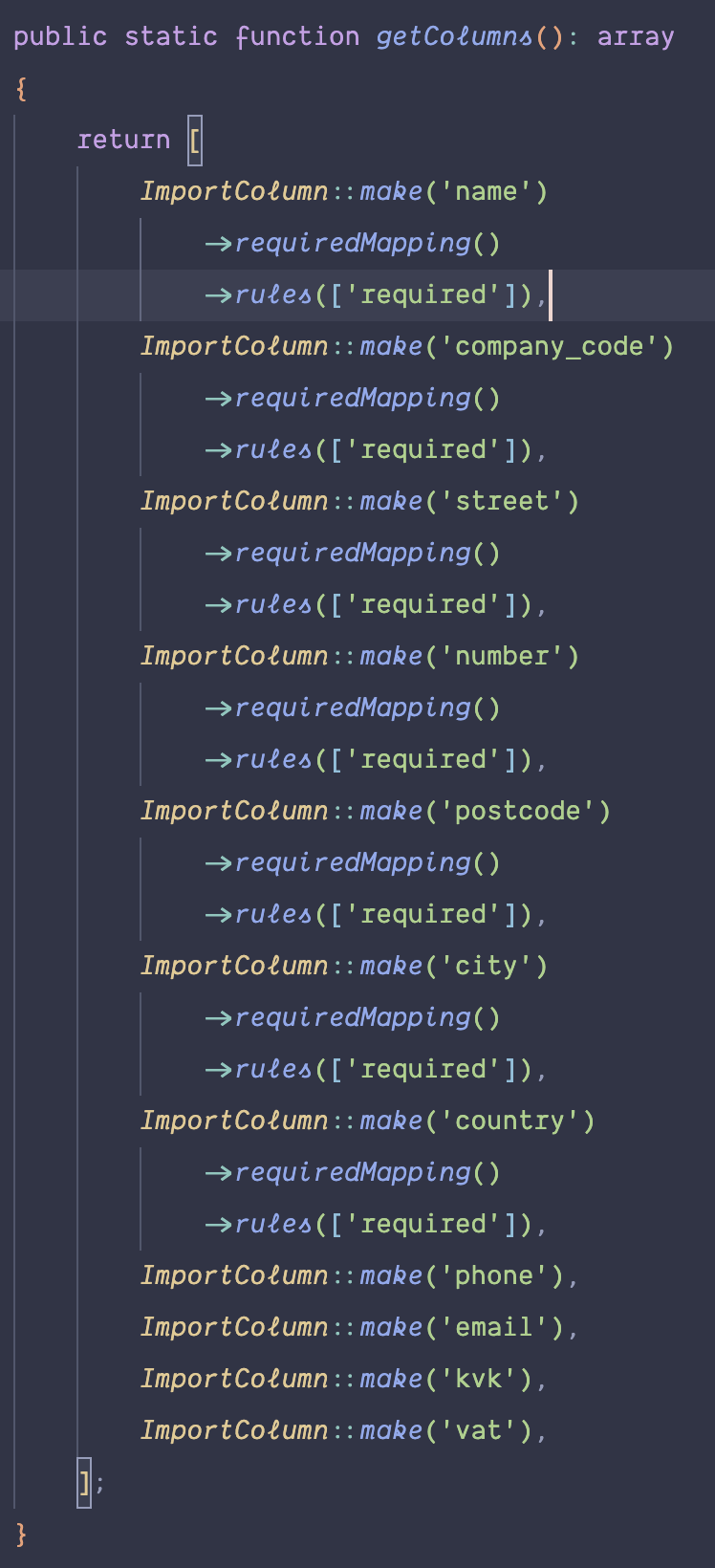
2 Replies
it is failing in the queue. But it is not giving any Validation Errors in the failed_import_rows

I would do: