Server Side Fetching
Hi I want to fetch data from server side and render the title based on it's content like in PHP.
I want to execute the fetch request to api from server side because I want to render the page to client when fetch is ready.
Do someone could provide an example script?
30 Replies
If you use createResource and deferStream thats what will happen
Ok but where do I have to write it?
Ok never mind now I can see that
Just read the docs
Thank you I got what I needed
I made example route to see how it will execute
It still shows me that
texturepack()[0]
is undefined
, but when I open the api page with the same id I get json as expected.
I expect it to be ready after fetching it to use itThe data from
createResource
will be undefined
initially, deferStream
doesn't stop that, but it will stop the HTML response from being sent until the data has been fetched and the <Title>
updates
if the html being sent in the response is correct then it's functioning as intendedyeah but it trows error cuz the first element is
undefined
Use optional chaining:
texturepack()[0]?.title
ok
but would it work on link preview like so in like meta tags?
I want to include it to my project
But they have to be rendered before the response i think so
yeah as long as
deferStream
is there the response will be delayedOh ok thanks!
I will tell you if it works when I finish that part
Do you know maybe why i get this getter error?
Cannot set property image_url of #<Object> which has only a getter
Route code
MetaPageProvider code
because you're writing to
props.image_url
like i said before, define a separate function
Oh now I see it
a) writing to props is bad, and b) components only run once so if you read props or signals in the component body it won't be reactive
sure
I just wanted to do not create another variable to handle the image_url
you could inline the string into the
href={}
oh yeah sure
I thaught this way it would be cleaner
how can i navigate to 404 when api result is an empty array?
that should work, i think you misspelled
length
thooh ok ok
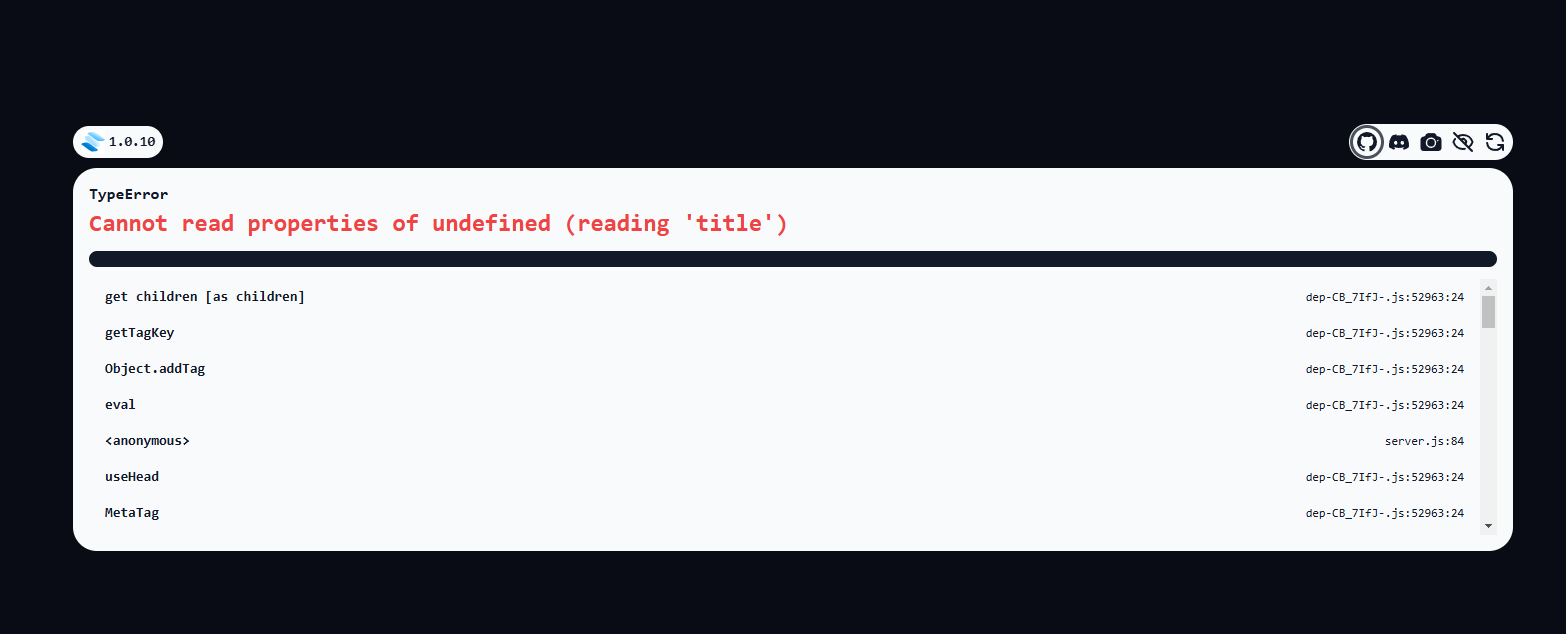
nah
you're only checking that
texturepack()
is undefined in the <Show>
, you should check for [0]
too
when={texturepack()?.[0]}
ok like so:
texturepack()?.[0]?.description
ok thanks
it works
:ryan:
https://lisia-nora.pl/testroute?id=24746dd2-b3fe-11ef-a5c9-3cecef0f5064
It does not show the meta content
:((
Like image etcthis how it looked like with using static json file
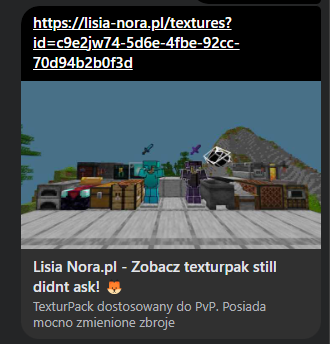
on messanger
how is
deferStream
getting passed to createResource
?I maybe found the error
I put
requestOptions ? [...]
instead of resourceOptions ? [...]
ahh yep
a small mistake
haha
i think you should be fine to just do
createResource(fetchMethod, resourceOptions)
?oh yeah good point
I tried that before and it throw error at me cuz i probably used the other param
as always
Zobacz texturepack Sukuna [32x]! 🦊
TexturPack dostosowany do PvP. Pozytywnie wpływa na twoją wydajność.
Oh yeah now it works
It's in Polish btw
Thanks man for helping me figure it out