not rerendering when changing ref value in async watcher
Hello!
as you can see in the code I have a ref called "dashboard" which would change upon tap changes and get different results, however it doesn't re-render until I refreshh the page. how to fix it?
18 Replies
Hi @Kyoko. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To address the issue of a ref not re-rendering when its value changes in an async watcher, there are a few potential solutions and considerations:
1. Ensure proper reactivity:
Make sure you're modifying the ref correctly. When working with refs, you should always use
.value
to access or modify their values. For example:
2. Use watchEffect
or watch
correctly:
If you're using a watcher, ensure it's set up properly. Here's an example of how to use watch
with a ref:
The deep: true
option ensures that nested changes are also detected Nuxt 3: Avoid losing Reactivity in your Vue Application (https://www.youtube.com/watch?v=sccsXulqMX8).
3. Consider using useAsyncData
or useFetch
:
If you're fetching data asynchronously, Nuxt provides composables like useAsyncData
and useFetch
that handle reactivity and re-fetching automatically. For example:
This setup will automatically re-fetch the data when currentTab
changes Docs > Getting-started > Data fetching > Watch (https://nuxt.com/docs/getting-started/data-fetching#watch).
4. Ensure SSR compatibility:
If you're using SSR, make sure your state management is SSR-friendly. Nuxt provides useState
for creating reactive and SSR-friendly shared state Docs > Getting-started > State Management (https://nuxt.com/docs/getting-started/state-management).
5. Check for reactivity loss:
Sometimes, reactivity can be lost when passing data between components or composables. Make sure you're not breaking the reactivity chain Nuxt 3: Avoid losing Reactivity in your Vue Application (https://www.youtube.com/watch?v=sccsXulqMX8).
Without seeing your specific code, it's difficult to pinpoint the exact issue. If you're still facing problems after trying these solutions, you might want to create a minimal reproduction of your issue and share it for further assistance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@322468991115198464> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
any help
1. what is
state
, how is it changed inside serviceStore
?
2. watch is not deep
by default, so if the state
is an object or an array, your watch handler won't be triggeredwatch is getting triggerd
each time
and dashboard is changed but its not reflected
What is not reflected @Kyoko
Umm if possible can you share a gif ??
Or screenshot?
the dashboard
Using nuxtlink
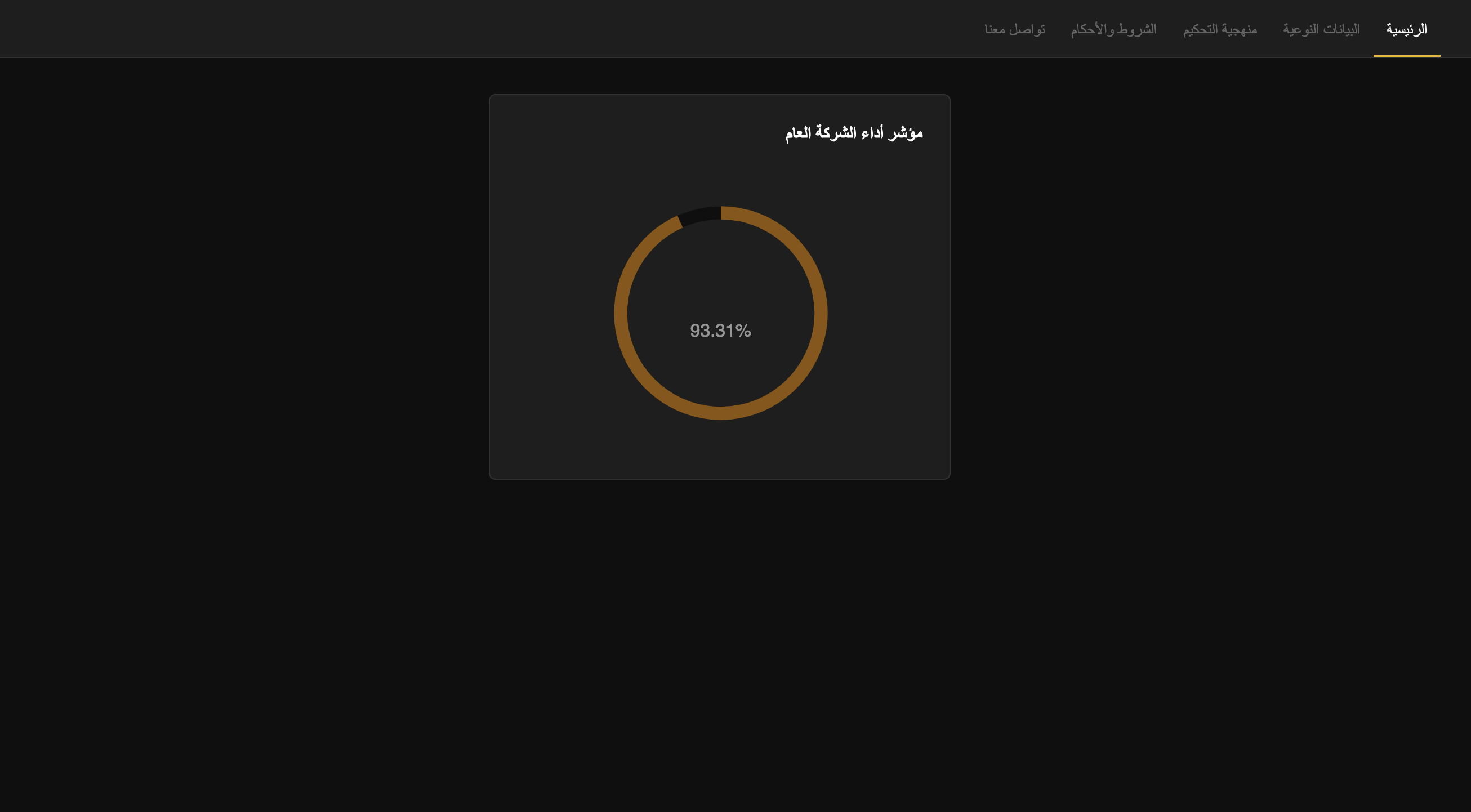
As you can see second pic
its not reflecting dashboard change
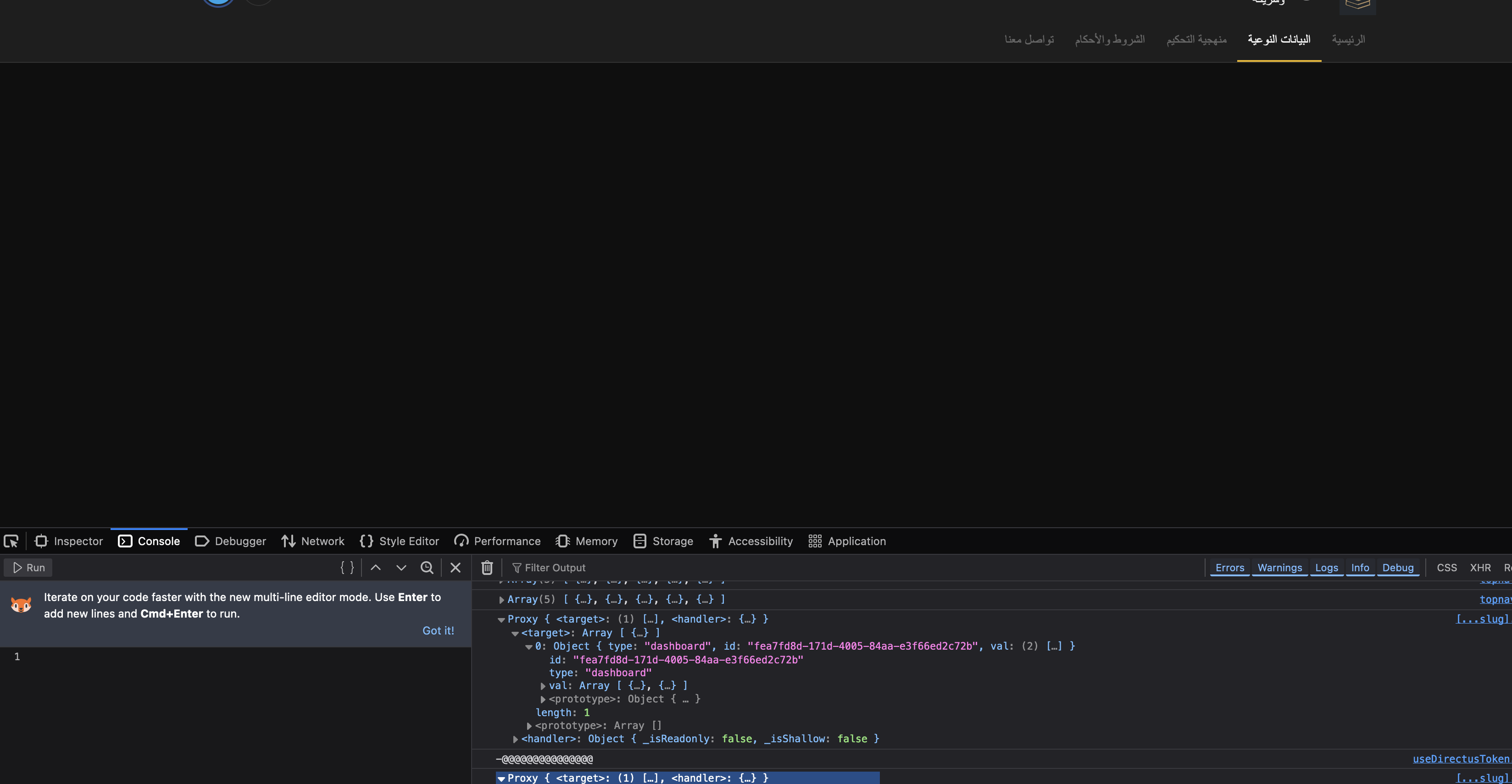
When I refresh is working
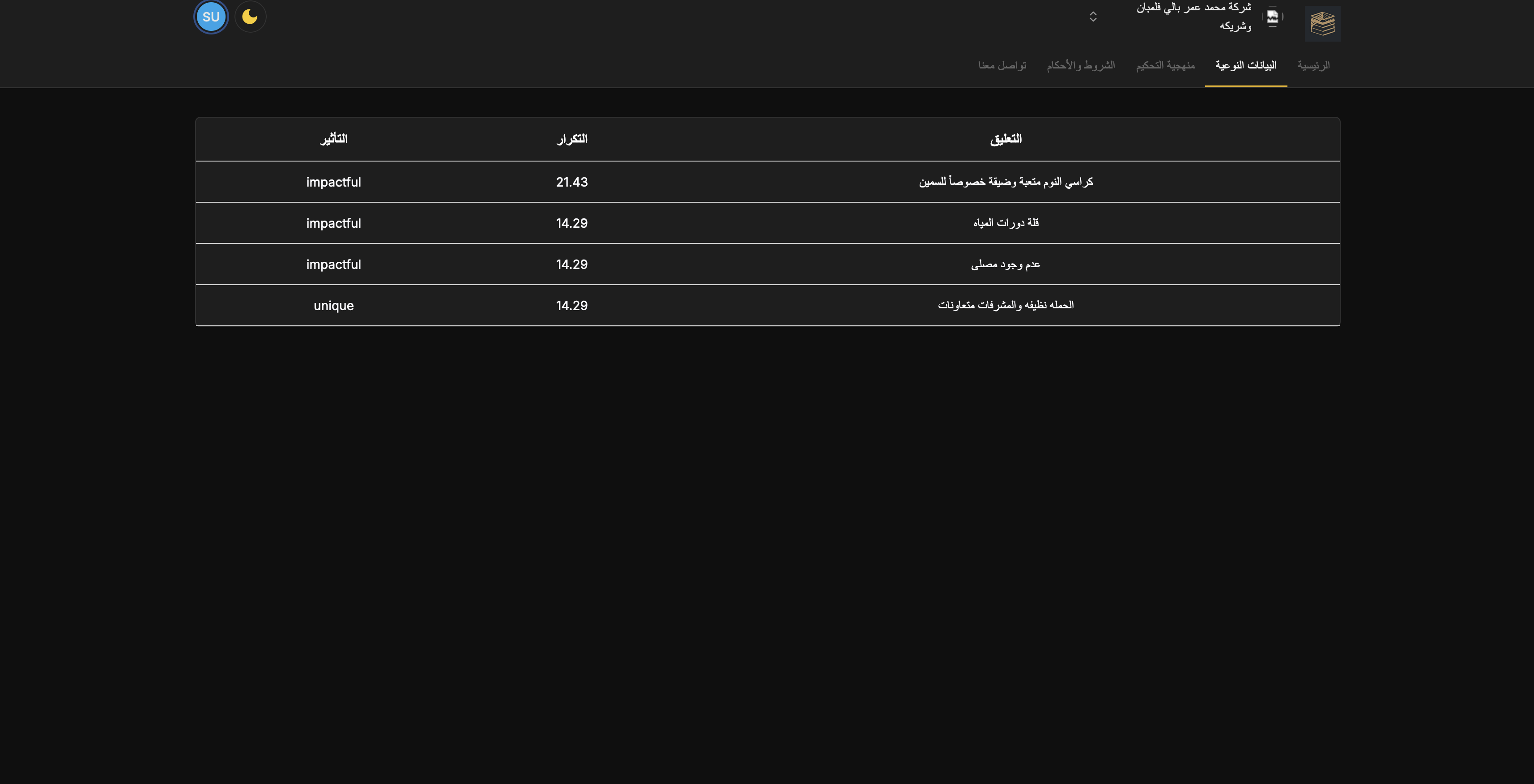
nuxtLink
@kiri
@Kyoko can you change immediate to true and try
??
same problem
Watch is triggered when navigating
dashboard is changed
but not reflected
Can you do {{ dashboard}}
On template and see if it is updating
@Kyoko
@kapa.ai the dashboard.value is updated ,it's not rendered in the ui untill refresh
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on your additional information, it seems the issue is not with updating the ref value itself, but with the UI not reflecting the changes immediately. This could be due to a few reasons:
1. Reactivity loss:
Sometimes, reactivity can be lost when passing data between components or when using certain operations. Make sure you're not accidentally breaking the reactivity chain Nuxt 3: Avoid losing Reactivity in your Vue Application (https://www.youtube.com/watch?v=sccsXulqMX8).
2. Computed properties:
If you're using computed properties that depend on
dashboard.value
, ensure they are set up correctly to react to changes.
3. Deep reactivity:
If dashboard
contains nested objects or arrays, you might need to use deep reactivity. You can force this by creating a new reference:
4. Asynchronous updates:
If the update is happening in an asynchronous context, you might need to wrap it in nextTick
:
5. Key attribute for v-for:
If you're rendering a list based on dashboard
, ensure you're using a proper key
attribute in your v-for
directive. An incorrect key can cause rendering issues Stack Overflow: Nuxt 3/Vue 3 Reactivity issue, while rendering component in v-for loop (https://stackoverflow.com/questions/77337404).
6. Watchers:
If you're using watchers, make sure they're set up correctly. For example:
7. Composables:
If you're using custom composables, ensure they're returning reactive references correctly.
Without seeing your specific implementation, it's challenging to pinpoint the exact cause. If you're still facing issues after trying these solutions, consider creating a minimal reproduction of your problem in a sandbox environment for further assistance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1077914005206736946> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@Kyoko please try all the above and see if that fixes it ..😊