TableWidget: Modal in View Actions empty data
What I'm trying to do: Show a modal when clicking on "View" within a TableWidget.
What I did: I created a custom widget that extends TableWidget. I added a view action that shows a modal for a user record. Even though the modal shows form elements, they are empty.
Code:
Additional Info:
I know I can just redirect the user to the /users/{id} route to view the record, but I really like the modal popup (it keeps them on the dashboard).
Question:
So is there a way I can bind the record to the form?
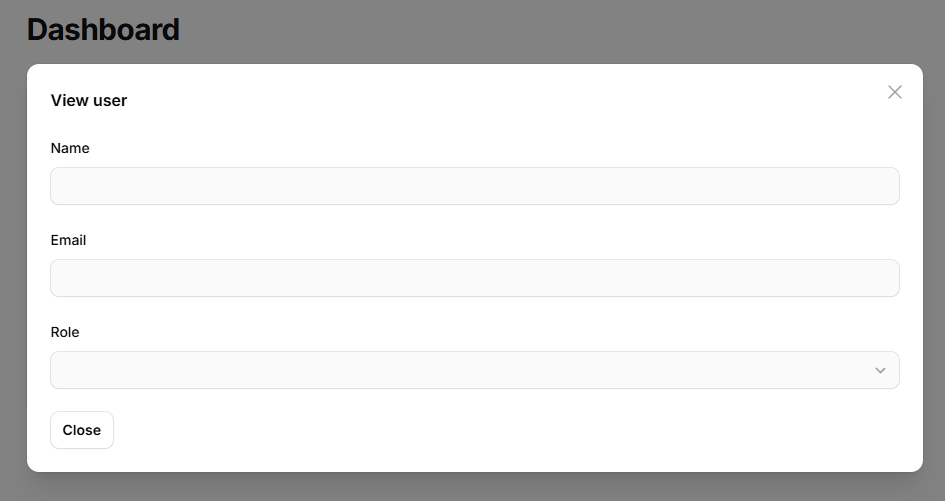
Solution:Jump to solution
Hopefully this helps someone - I was able to solve this by passing
->statePath('state')
, this also requires that the Widget has this state defined as public
, it will not work if not defined in the class.
This is the GenericTableWidget
```php
<?php...3 Replies
Solution
Hopefully this helps someone - I was able to solve this by passing
->statePath('state')
, this also requires that the Widget has this state defined as public
, it will not work if not defined in the class.
This is the GenericTableWidget
Now you can extend this class to show a specific resource such as Users:
Make sure that in your UserResource
you have defined the static function getTableColumns
:
You can now add your widget onto the dashboard with:
If you have done everything correctly, you should now see the User table (with actions that appear as modals) on the dashboard.
I copied this exactly and am getting
Typed static property App\Filament\Widgets\GenericTableWidget::$resourceClass must not be accessed before initialization
... any ideas?