80 Replies
It's a good way to make sure people aren't doing silly things. If you use Func/Action then you can pass anything with the same parameters and return type, even though it could do something wildly different. Restricting arguments to a specific type of delegate means whoever's using that function has a sanity check to make sure they're not misusing it
Func/Action/Delegate are also part of the base class library whereas delegate is just a part of the language, though I can't think of many situations where that'd matter
Oh when, not why, sorry
Though the same logic applies
Func
and Action
are very simple. Those are the types I expect as input, this is (or isn't) the type I will give as an output.
Delegates can be generic, can have named parameters, can have ref
parameters, pretty sure out
as wellCustom delegates also allow optional arguments, and I sometimes like to use them because naming them is often helpful for readability
So instead of an Action<T> I could have a "XXXCallback" in the codebase
What about using the Delegate type directly?
Never used it
like this
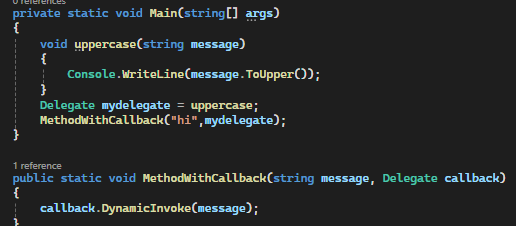
Makes no sense to me over using an
Action<string>
It smells of using an object
or dynamic
that's basically "whatever lmao"Delegate is the base class for Func and Action iirc
i thought they were defined using the
delegate
keyword
i understand that but isnt that the same as Action<T>?
they're both genericFunc and Action are delegate types in the base class language and are delegates, the
delegate
keyword defines a new delegateAction<int>
will take only a void
method that takes one single int
parameter
Delegate
will take anything
void Foo(int x)
? Sure. string Bar(int a, int b, Person c)
? Why not
(int a, string b, Func<int, Person> c) Baz(IQueryable<Dictionary<string, int>> quaz)
? Sure, fuck me upwell
handleEvent("click", callbackgoeshere)
what if i want literally any callback
I dont really wanna impose a restrictionHow do you reason about it?
When you invoke it, what do you give it as parameters?
i want to let the user handle the event hwoever they want? if the click event gets fired they can chose any behavior they want
What do you expect in return?
they could launch a new process. they could print to the console. they could close the current process. idk
Right, so, the user passes a delegate with this signature:
how do you invoke it?
delegate.Method.Invoke()?
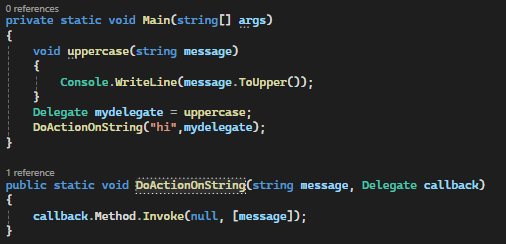
And what parameters you give it, if it can be anything?
well Invoke takes object[]
so anything...
not sure how to answer that
Yeah, and what will be inside of it?
Exactly
The user can still do anything they want inside of an
Action
No parameters, void return type
It still captures outside variables, for examplesure, i understand that
i see action has some syntactic sugar to let you invoke it as if it was a method
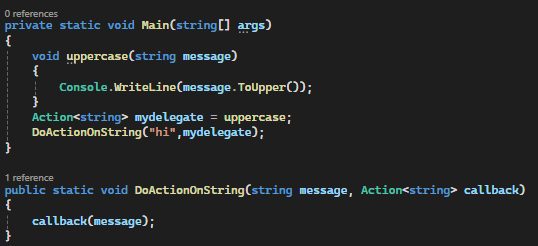
Yep
plus,
Delegate
doesn't actually give you a free-for-all, you just get a runtime error if the arguments don't match those expected by the actual argument provided
so you're essentially breaking out of the type system for zero gain
in much the same way as passing object
s aroundare you two acquainted with
object.addEventHandler
in JS?yes
What it expects is a function that takes an
Event
and returns nothing
So an Action<Event>
in C# termsthe way this works is that
object.addEventHandler(eventName : string, callback : Function)
will add the callback instead of overwriting. Meaning that you can do that multiple times for multiple functions and trigger all of them when the event fires
Is this what multicasting is in C#?What you're looking for is events, then
Or just a list of
Action
s you can keep adding to and execute them in a loopI dont quite get this then
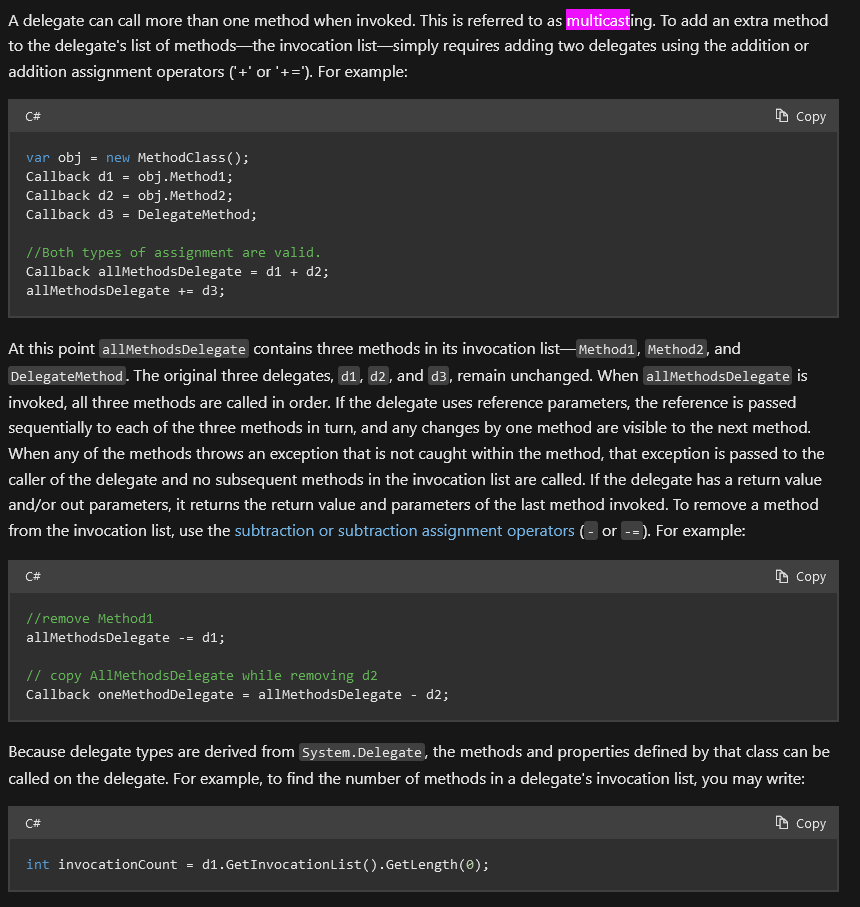
multicast delegates aren't quite the same as event handlers, no
Uh, I guess another weirdness of
Delegate
s...?You're casting multiple methods to the delegate type, then when you invoke that delegate it sequentially invokes each method assigned to it
I won't be of much help here, I literally never seen bare
Delegate
used anywheremulticast delegates are essentially like adding multiple delegates together so that you can refer to the invocation of both (in the case of two) in one place
you can do events that way, but C# has a convention with events using the
event
keyword
Because all delegates have invocation lists, not just events
the convention is you define your delegate type for the event, say
then you can subscribe to that eventyou can have a multicast delegate without having anything to do with events, whereas
addEventListener
is specific to events on an instancenah this is also applicable to
Action
sbut you can just as well have
MyEventDelegate MyEvent
on its own and subscribe to it, you just won't have a couple of extra protections, bells and whistles that come with events specificallyfrom what i understand i can do
and when the delegate gets invoked as a callback it will execute the three methods in order, correct?
When you invoke any delegate type, whether it be Func, Action, Delegate, Event, etc it just invokes every method added to its invocation list sequentially, and if that delegate type has a return type, it returns the result of the last delegate in its invocation list
Correct, yeah
got it
if that was
Func a = func1 + func2 + func3;
with a return type, it'll return the result of func3
only, not a sum of them, but 99% of the time that doesn't matterwhy are people saying that events should be avoided and only delegates should be used?
Nick Chapsas
YouTube
Are events in C# even relevant anymore?
Check out my courses: https://dometrain.com
Become a Patreon and get source code access: https://www.patreon.com/nickchapsas
Hello everybody I'm Nick and in this video I will explain why I think that events as a feature in C# is obsolete and how we can implement the same event-like mechanism in a modern and elegant way.
Timestamps
Intro - 0:00...
For content
ic
honestly, beats me. you can use delegates in a similar way to events but there are distinct differences.
i was wondering if there was any merit to this
to me that video is pure bunk. someone linked it earlier as reasoning behind abandoning events and the conclusion of that video is to use MediatR. it's wild.
alright, so i'm a typescript/angular dev right. you would use C# events/delegates if you wanted to implement the subscriber/observer pattern?
not that there's anything wrong with MediatR but you certainly wouldn't use it in a lot of cases you'd use events.
if i recall its called something else in the C# world, publisher/subscriber i think
if you're used to JS events, they're pretty much a direct analogue
i see
do people use libraries for this kinda stuff?
there's just some syntactic sugar around adding/removing event handlers
is rx a thing in the C# ecosystem
i.e.
x.addEventListener("click", () => { ... })
becomes x.Click += () => {...}
that is oddly wpf'esque
I remember trying to make a WPF desktop application when i was younger, i remember doing that for some buttons. little did i know i was using delegates this whole time
well they're events but yes, events are delegates with some additional rules
yeah i hate the world
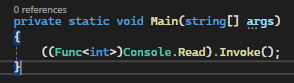
what's there to hate?
but hold on
i can use the delegate keyword to create anonymous methods?
why has no one told me this
vs
are both of these "anonymous methods"?
yeah they're both anonymous functions
you could also do
Action<string> = Console.WriteLine
in this caseI think you can only do that if the method group has only one overload
in this case it might be confused as to which WriteLine you're talking about
unless it infers it from the
<string>
?they're both anonymous methods
can confirm, it does infer it
it's only an issue if you do
someone could help me with a save botton?
syntax in the second came about in a later version of C#
they are called lambdas?
lambda expressions, yeah
is it necessary to instanciate a delegate type?
what's the difference between
and
no difference
thank you
anyhow I'm off, good luck in your delegate travels
<3 take care
if you're using += then yes, but if you're assigning to md then no
Multicast delegates is how events are implement in C# as far as I know.
Delegates are not that often used, but they are valuable if you go deeper into the functional style of C#.
https://www.youtube.com/watch?v=2TXvwUgaMHs&t=1s
Zoran Horvat
YouTube
Master the Design of Functional Behavior in C#
How do we implement functions in a class-based programming language, such as C#? The latest C# programming language specification offers a rich syntax that supports functional programming in this versatile language.
Functional design is known for its simplicity and the short code it produces compared to other design techniques we apply. It is ba...