cookies not send to external api
Hello, does anyone know why my req.cookies is empty when my cookie is sent to my REST api?
back-end :
front-end :
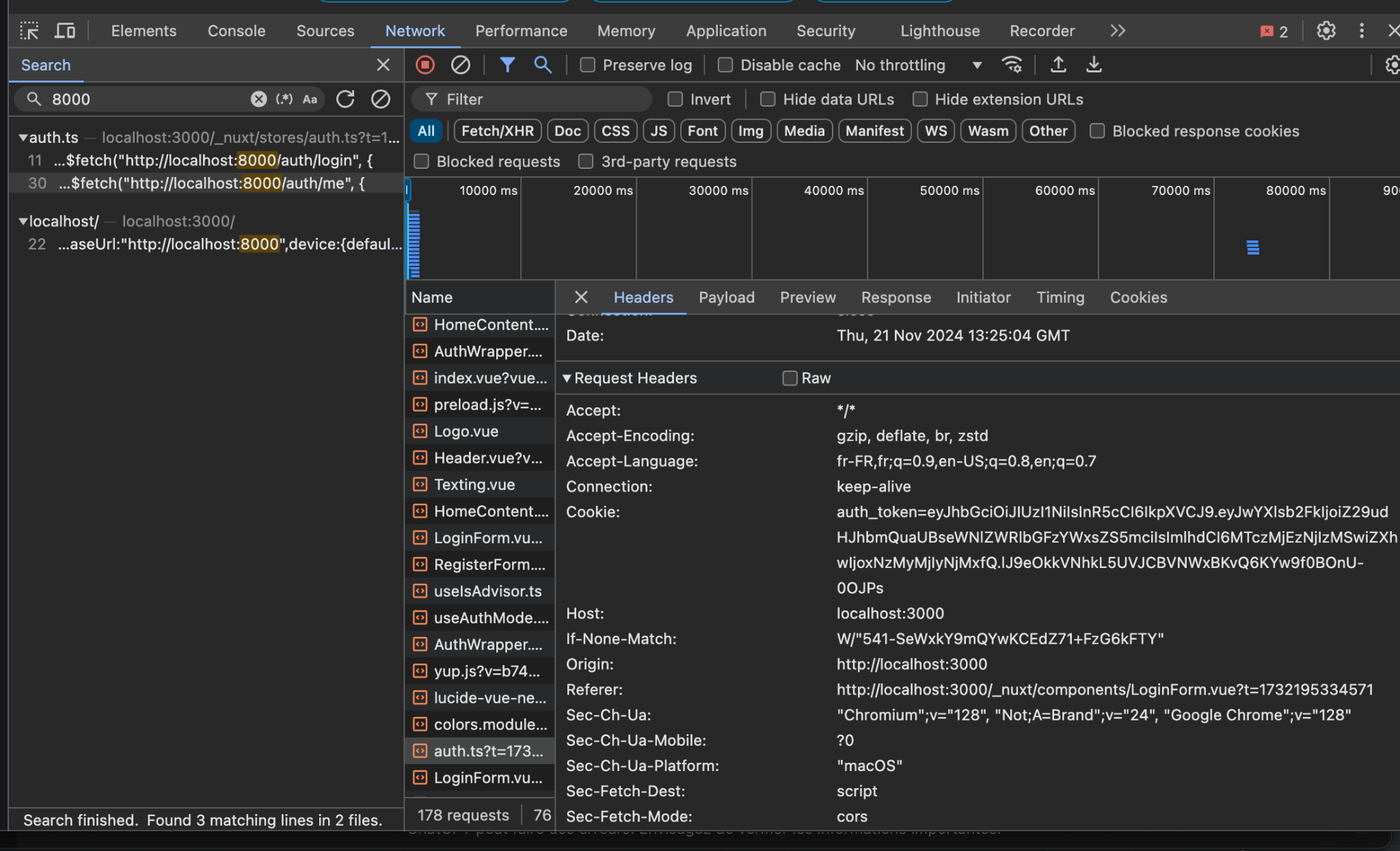

14 Replies
Hi @Abou. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the information provided in your question and the knowledge sources available, it appears that you're having issues with cookies not being sent to an external API when using Nuxt. Let's address this problem:
1. Server-side vs. Client-side requests:
When making requests from the server-side in Nuxt, cookies are not automatically forwarded. This is because the request is made internally within the server, and it doesn't include the user's browser cookies by default.
2. Forwarding cookies:
To solve this issue, you need to manually forward the cookies from the client to the server-side request. You can use the
useRequestHeaders
composable to access and proxy cookies to the API from the server-side.
Here's how you can modify your fetchUser
function to forward cookies:
This modification uses the useRequestHeaders
composable to include the 'cookie' header in your request Docs > Getting-started > Data fetching > Passing Headers and cookies (https://nuxt.com/docs/getting-started/data-fetching#passing-headers-and-cookies).
3. Using useRequestFetch
:
Alternatively, you can use the useRequestFetch
composable, which automatically forwards the request context and headers for server-side fetch requests:
This approach uses useRequestFetch
to automatically forward the necessary headers Docs > Api > Composables > useRequestFetch (https://nuxt.com/docs/api/composables/use-request-fetch).
4. External API considerations:
Since you're making a request to an external API (http://localhost:8000), make sure that your API is configured to accept cross-origin requests and handle cookies properly. You might need to set up CORS on your backend to allow credentials and specific origins.
By implementing one of these solutions, you should be able to successfully send cookies to your external API from both server-side and client-side requests in your Nuxt application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@811720203531976714> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai but it give me all cookies, i just want one in particular (auth_token), like i want the value not a list of all cookies
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you want to retrieve a specific cookie value (auth_token) rather than all cookies. Let's modify the approach to focus on getting just that particular cookie value. Here's how you can do it:
1. Using
useCookie
:
The simplest and most straightforward way to get a specific cookie in Nuxt 3 is by using the useCookie
composable. This method works on both client and server side:
This approach uses the useCookie
composable to directly access the 'auth_token' cookie Docs > Api > Composables > useCookie (https://nuxt.com/docs/api/composables/use-cookie).
2. If you need to access the cookie specifically on the server side, you can use the getCookie
function from the h3
package in a server route:
This method is useful when you're working within server routes or API endpoints Docs > Api > Composables > useCookie#cookies-in-api-routes (https://nuxt.com/docs/api/composables/use-cookie#cookies-in-api-routes).
3. If you're using useRequestHeaders
and still want to extract just the 'auth_token', you can parse it manually:
This approach parses the cookie string to extract just the 'auth_token' value.
By using one of these methods, you can retrieve and use the specific 'auth_token' cookie value in your API requests, rather than sending all cookies.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@811720203531976714> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
but why i can't just get req.cookies on my external api ? @kapa.ai
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the information provided in the knowledge sources and our previous conversation, I can explain why you can't directly access
req.cookies
on your external API when making requests from Nuxt:
1. Cross-Origin Requests:
When you're making a request from your Nuxt application to an external API (in your case, <http://localhost:8000
>), you're dealing with a cross-origin request. By default, browsers do not send cookies with cross-origin requests due to security reasons.
2. Credentials Setting:
Although you've set credentials: "include"
in your fetch options, which should allow cookies to be sent with cross-origin requests, there are additional steps needed on both the client and server side to make this work.
3. Server Configuration:
Your external API needs to be configured to accept and handle cookies from cross-origin requests. This involves setting up proper CORS (Cross-Origin Resource Sharing) headers on your server.
To resolve this issue and access cookies on your external API, you need to:
1. On the Nuxt (client) side:
Continue using credentials: "include"
in your fetch options.
2. On your external API (server) side:
- Enable CORS and configure it to accept credentials.
- Set the Access-Control-Allow-Credentials
header to true
.
- Specify the allowed origin(s) in the Access-Control-Allow-Origin
header. Note that when allowing credentials, you can't use the wildcard *
for the origin.
Here's an example of how you might configure your Express.js server to accept credentials:
After making these changes, your external API should be able to access req.cookies
.
Remember, handling cookies in cross-origin requests requires careful consideration of security implications. Always ensure you're following best practices for secure cookie handling and cross-origin resource sharing.
Stack Overflow: Laravel Sanctum 419 "CSRF token mismatch" error with Nuxt (https://stackoverflow.com/questions/78543475) provides an example of dealing with cross-origin cookie issues, which might be helpful in understanding the complexities involved.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@811720203531976714> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
i already configure my cors @kapa.ai :
import express, {Express} from 'express';
import {configDotenv} from "dotenv";
import cors from 'cors';
import cookieParser from 'cookie-parser';
import mongoose from "mongoose";
import emailRoutes from "./routes/emailRoutes";
import authRoutes from "./routes/authRoutes";
const app: Express = express();
configDotenv();
app.use(cookieParser());
app.use(express.json());
app.use(cors({
origin: 'http://localhost:3000',
credentials: true,
}))
/app.use((req, res, next) => {
console.log('Cookies: ', req.cookies);
next();
})/
mongoose.connect(process.env.MONGO_URI '', {}).then(() => {
console.log('Connected to the database');
app.listen(PORT, () => {
console.log(
\x1b[32mServer is running on port ${PORT}\x1b[0m
);
});
}).catch((error) => {
console.error('Error connecting to the database', error);
})
const PORT = process.env.PORT 3000;
app.use('/auth', authRoutes);
app.use('/email', emailRoutes);Let me check the knowledge base for you again. One moment! :books:
----------------------
Thank you for providing your server configuration. It appears that you have already set up CORS and cookie parsing correctly. Let's analyze your configuration and suggest some potential solutions:
1. CORS Configuration:
Your CORS setup looks correct. You've set the origin to 'http://localhost:3000' and enabled credentials, which should allow cookies to be sent from your Nuxt application.
2. Cookie Parser:
You're using the
cookie-parser
middleware, which is good. This should parse the cookies and make them available in req.cookies
.
Given this configuration, req.cookies
should be populated when requests are made from your Nuxt application. If you're still not seeing the cookies, here are a few things to check:
1. Cookie Setting:
Ensure that the cookie is actually being set correctly. You can check this in your browser's developer tools, under the Application or Storage tab.
2. Cookie Properties:
Make sure the cookie is set with the correct properties, especially:
- httpOnly: false
(if you need to access it via JavaScript)
- secure: false
(for localhost testing)
- sameSite: 'lax'
or 'none' (be cautious with 'none' in production)
3. Request Headers:
In your Nuxt application, ensure you're including credentials in your fetch requests:
4. Debugging:
Uncomment your middleware that logs cookies to see if they're being received:
5. Check for Proxy Issues:
If you're using a proxy (like in development with Nuxt), ensure it's configured to forward cookies correctly.
6. Browser Security Settings:
Some browsers have strict security settings that might prevent cookies from being sent in certain scenarios. Try testing in a different browser.
If after checking these points you're still not seeing the cookies in req.cookies
, the issue might be in how the cookie is being set or sent from the client side. You might want to double-check your Nuxt application's cookie-setting logic.
Remember, for security reasons, cookies with the HttpOnly
flag set cannot be accessed by client-side JavaScript, so make sure your 'auth_token' cookie doesn't have this flag if you need to access it on the client side.
Stack Overflow: How to use Node.js with Nuxt.js? (https://stackoverflow.com/questions/77716684) provides some additional context on setting up Express with Nuxt, which might be helpful in troubleshooting your setup.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@811720203531976714> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
when i do this :
app.use((req, res, next) => {
console.log('Cookies: ', req.cookies);
next();
})
i've the cookie, but not inside my req.cookies
and btw, my cookie is set correctly with what u said
@kapa.ai
idk why i still don't have my cookies inside req.cookies
Let me check the knowledge base for you again. One moment! :books:
----------------------
This is an interesting situation. It seems that your cookies are being received by the server, but they're not being parsed correctly into
req.cookies
. Let's explore a few potential reasons and solutions:
1. Cookie Parser Middleware Order:
The order of middleware in Express is important. Make sure that the cookie-parser
middleware is applied before your custom middleware and routes. Try moving the cookie-parser
middleware to the top of your middleware stack:
2. Cookie Parser Secret:
If you're using signed cookies, make sure you're providing the same secret to cookie-parser
that you used to sign the cookies. If you're not using signed cookies, you can try passing an empty secret:
3. Manual Cookie Parsing:
If cookie-parser
is still not working as expected, you can try parsing the cookies manually to see what's being received:
4. Check for Cookie Encoding:
Ensure that the cookies are being properly encoded when set. If they're not, it might cause issues with parsing.
5. Use h3
Package:
Since you're working with Nuxt, you might want to try using the h3
package's getCookie
function, which is designed to work well with Nuxt's server-side environment:
This method is recommended for Nuxt applications, as mentioned in the H3 documentation on handling cookies (https://h3.unjs.io/examples/handle-cookie#get-a-cookie).
6. Debugging cookie-parser
:
You can try to debug the cookie-parser
middleware to see if it's functioning correctly:
Run your application with DEBUG=cookie-parser node your-app.js
to see the debug output.
If after trying these solutions you're still having issues, it might be worth checking if there's any conflict with other middleware or if there's an issue with how the cookies are being set on the client side.
Remember, cookies can be tricky when dealing with cross-origin requests, so ensure that all CORS settings are correct on both the client and server side.
Stack Overflow: Using cookies in Nuxt 3 APIs and Middlewares (https://stackoverflow.com/questions/77590950) provides some additional context on handling cookies in Nuxt 3, which might be helpful in troubleshooting your issue.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@811720203531976714> Kapa.ai is still learning and improving, please let me know how I did by reacting below.