How to Set Width of a New Container Element to Match an Existing Element's Width in JavaScript?
I’m working on a project where I need to dynamically create a new container element and append an existing element into it. My goal is to ensure that the new container element has the same width as the existing element, regardless of how that width is defined (e.g., auto, max-content, inline styles, or specific pixel values).
here's what I have done done so far
How can I obtain the width of
containerElement
regardless of how it’s styled (e.g., using max-content
, auto
, or explicit pixel values)?26 Replies
element.offsetWidth
I think
I also want to get a value of
auto
if there's no specific width set to an element, and also for max-content
, min-content
, fit-content
etc..Have a look into this
MDN Web Docs
Window: getComputedStyle() method - Web APIs | MDN
The
Window.getComputedStyle() method returns an object
containing the values of all CSS properties of an element, after applying active
stylesheets and resolving any basic computation those values may contain.
offsetWidth should return u the width even if it is set to auto
lemme check again
I believe it just gives the width of the element
As in, even if it’s auto, the intrinsic size is still going to be a certain amount of px wide
So it’ll return that. I don’t think it looks at keywords
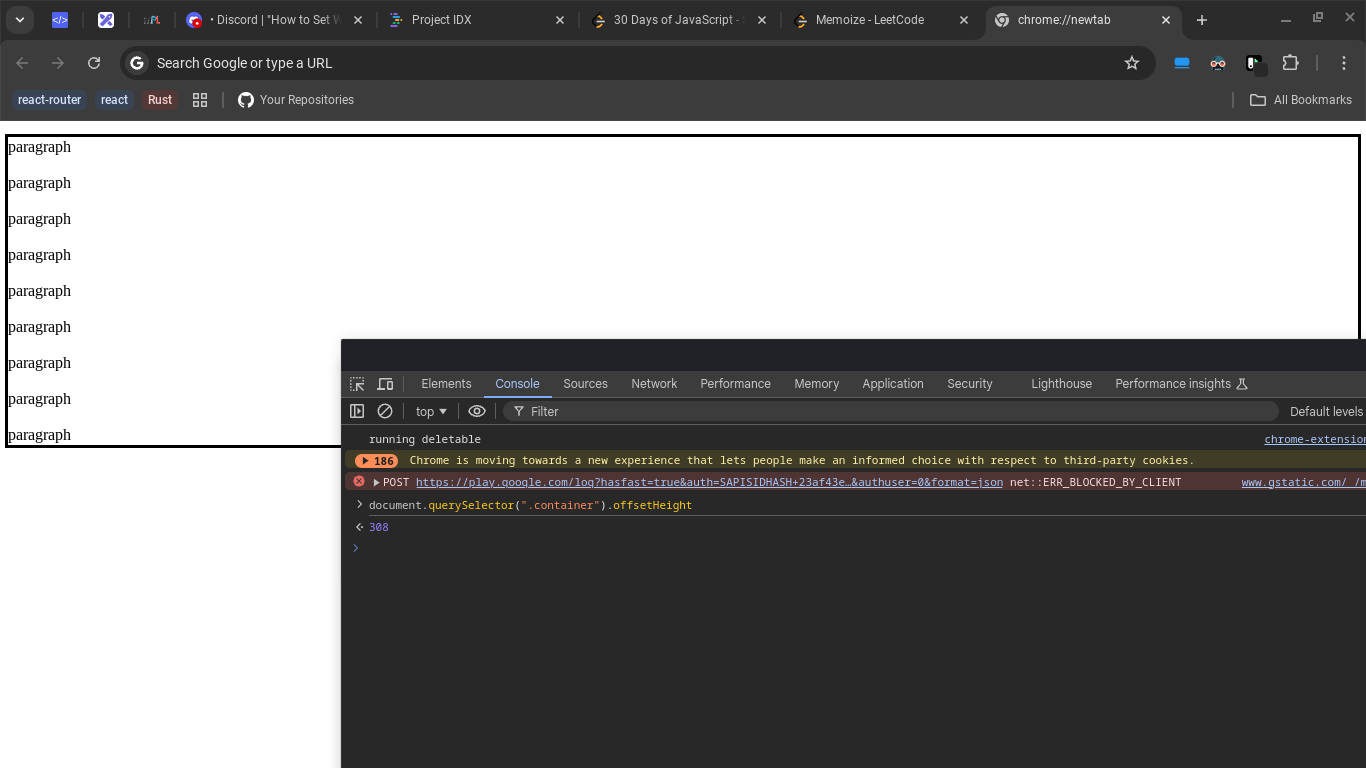
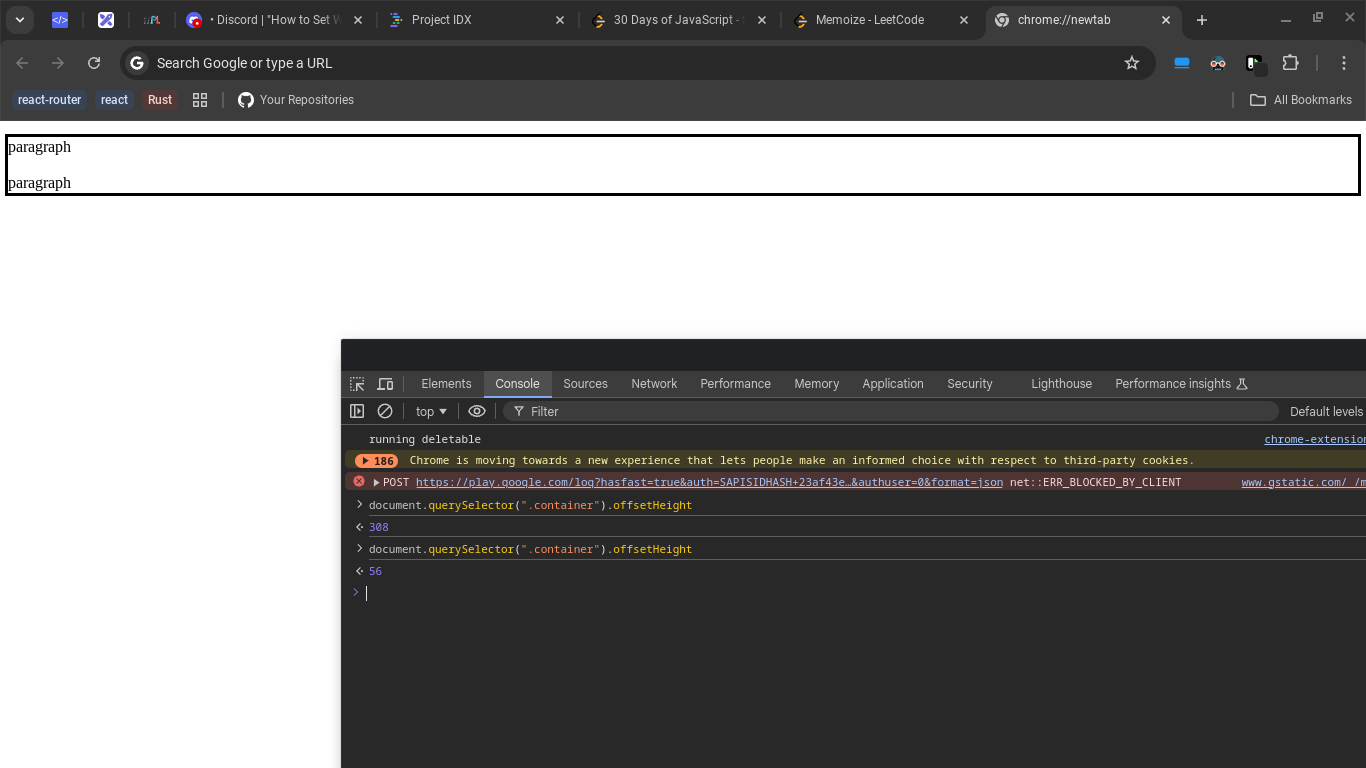
i mean it does return u the height in px
the height of the div is set to auto
so what is the issue with offsetWidth or height
I think if there’s a set width they want that number
And if not they want auto or fit content etc
Like the actual value of the variable to be ‘auto’
But only they can confirm
hm
i don't think he wants the calculated size. he wants the property value as declared in css or else default. don't think it is possible without reading the whole style sheet and trying to find it
yep
I recommended get computed style
I think that should do it right?
nah i think that returns u the value
similar to offsetWidth or height
computed style will return the exact size of the rendered element. so no,
Ah I see then
u do need to read the stylesheet
ig just use a variable
css variable
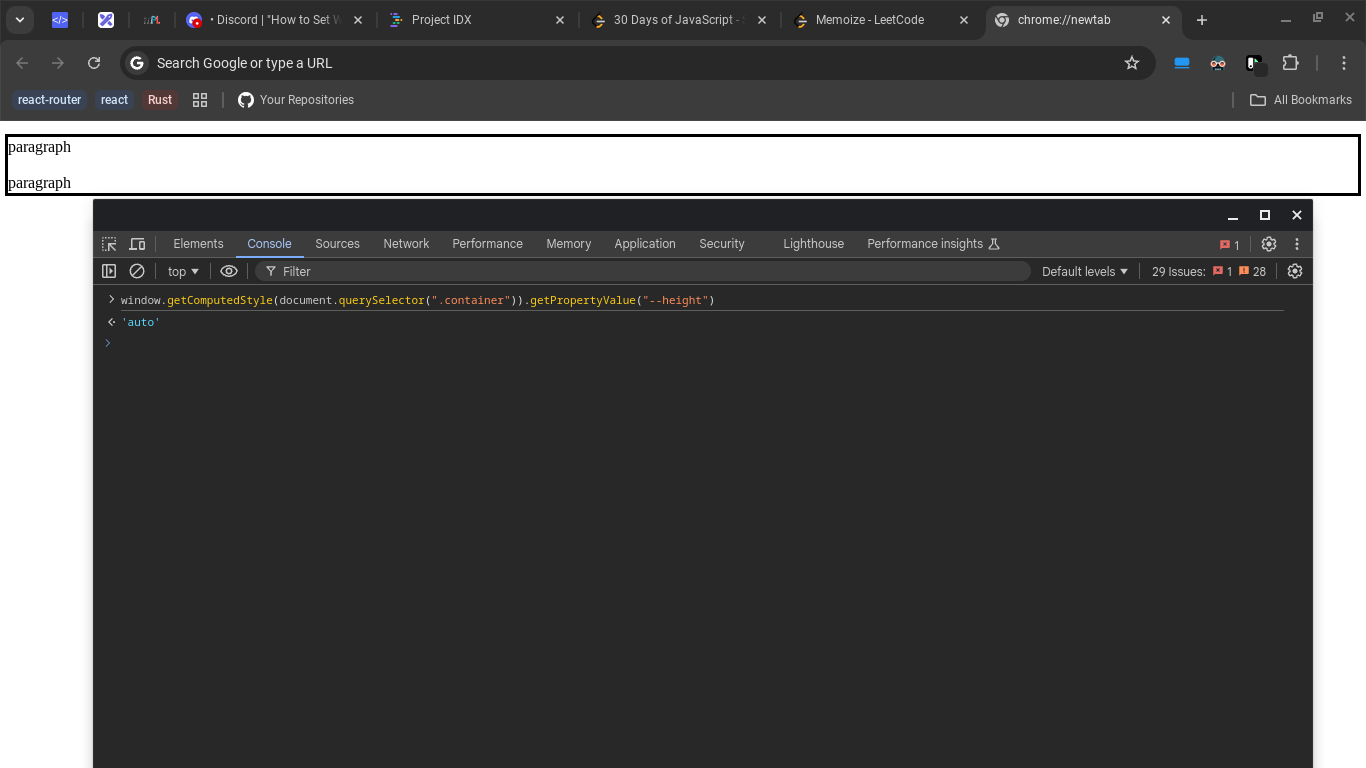
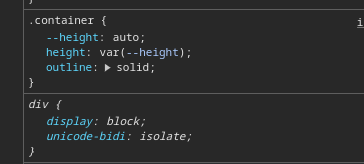
I think this would work with reading the style sheet (it does need the exact selector though)
--edit: optimized
had some help from the interwebs to put it together
Thank you for your feedback really appreciate it, but, there are several problems with this function. First, there are specificity issues it might return the first match, which may not be the most specific style applied to the element. Also, if an element has inline styles, the function does not acccount for those, as well if the application has a lot of stylesheets, iterating through all of them may not be efficient. I also want the selector matching to be more configurable, as some of the users utilize combinators or attribute selectors for their elements. im trying to use this in a javascript library that im writing so i want to find a soluion that is more flexible, if there is no other way im just gonna set a default width to the new containerElement or just allow them to set a specific width to it
Yea, it clearly isn't the best approach, you've mentioned the catches.
i wonder how the devtools show the properties/values on element inspection. There must be something we're missing
if only there is similar method to
getComputedStyle
, but instead of returning the computed style value of an element, it returns the value that was set for the property, If no value was set, it would return the default value of that property.
For example:
im still searching for a way similar to this but still no luckStill trying it with my approach. First I check for inline styles, then the matched selector, and if not found the computed value (you can change to 'auto')
For the matched selector, specificity is a hassle, i tried to calculated the selectors, but for complexer ones, it's really hard (lot of regex)
here a pen you can play with https://codepen.io/MarkBoots/pen/rNXJVow?editors=1111
I know this is not usable for production, but it's a nice puzzle
thanks again, i'll play around with this