Losing reactivity when importing a signal from a library that uses SolidJS
Hey 👋
I have a library that uses SolidJS, and a few projects that import said library.
I have the following code in one of my library's util files:
and the Vec2 class that I'm using (with all the other irrelevant methods removed, to keep the code snippet short):
The code above works as expected inside the library; When the window is resized, the signal updates, and the console.log effect gets called.
The issue I have is inside another project, that imports my library. The effects there doesn't get called after the initial render.
Here's my
index.tsx
entrypoint in the example project, it's more-or-less the default one when setting up a new SolidJS project:
And the App.tsx
:
Any hints on why the effect inside the example app only gets called once (on load), while the one inside the library works as intended?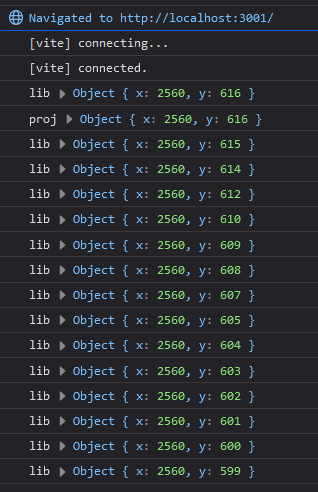
10 Replies
You're showing code, but you're not showing where, or how it's imported. Neither your
index.tsx
nor App.tsx
appear to have any imports that relate to the code defined at the top.I removed parts of the code that I thought were irrelevant to keep the messages shorter. I edited the original message with all the imports added.
Vec2 has some more stuff, but none of it should relate to SolidJS.
As for the exporting, give me a sec...
in the library, in
src/index.ts
(the entrypoint), I'm exporting everything from my utils
dir
and then in the src/utils/index.ts
im exporting the screen-utils:
You can also use this primitive which helps to set up a resize observer
https://primitives.solidjs.community/package/resize-observer#makeresizeobserver
Solid Primitives
A library of high-quality primitives that extend SolidJS reactivity
The full repo is available here, at least for the library. The example app I'm using is not on the repo:
https://github.com/LordDeatHunter/nodeflow
Thanks, I'll probably look into that, but I'm still curious as to what causes the reactivity issue in my scenario in the first place.
Well... the answer is, something outside of what you've posted.
Solid Playground
Quickly discover what the solid compiler will generate from your JSX template
Works just fine in the playground.
Yes, but you have all 3 files in the same project. It works fine if everything is in a single project, but that's not the case for me.
In my case, I have the screen utils and Vec2 class in 1 library project, and I have that installed on a 2nd example app (the index.tsx and Apps.tsx files are here), which is where the problem occurs.
@Emily here's a minimally reproducable version:
https://codesandbox.io/p/devbox/practical-vaughan-lwjs4f
the main directory is the library containing the screen size logic
the
example/
directory contains the currently running project
first text is the imported signal
2nd line is a component that does the same, but inside the libraryMy best guess is that the bundling process is messing something up, since importing from a clone of the source repo works fine, and importing from the npm package doesn't. Exact same test code, just toggling the path of the import statement.
Unfortunately that's a little beyond my knowledge to debug.
Alright, thanks anyway. I'll see if I can zero in on exactly what's causing the issue. hopefully its just a bundler misconfiguration and not an issue with solidjs
I found a solution by adding the following to my
build.rollupOptions
in vite.config.ts
:
Hopefully this can help someone else with the same troubles 🙂