use `configureUsing` to avoid repetition
I have many password fields in my application so i'd like to use
Problem is this is the boot function runs BEFORE the component is created i want it to execute after so i don't have to manually add
->revealable(config('panel.password.revealable'))
to every TextInput of the password kind.
I tried usingand it will always return
false
same goes for
it will always return text
even though its a password
, email
or alphanumeric
field.
I don't want to make my own component just for passwords cause i want to apply similar edit to other components as well
Thanks for your help 🙂21 Replies
I had a similar issue a while back. Try putting it in the register() function instead.
Not sure im doing this quite right where should i put the register() method ?
The PanelProvider that gets made using the artisan commands don't put it there by default but any ServiceProvider can have a register() method since they all ultimately should be extending Illuminate\Support\ServiceProvider. If it's not there, just add it.
And those things can be in any ServiceProvider. For maintenance simplicity, I created a generic ConfigurationServiceProvider (basic Laravel - nothing Filament specific) and put all my global configureUsing items in there.
Sometimes boot() works fine but sometimes that gets hit too soon and register() works better.
I tried putting it under
AppServiceProvider::register()
seems like it still is too early
Do you need to import the ConfigurationServiceProvider
somewhere else for it to get called later or bootstrap/providers.php
should do the job ? cause mine is still too early just like if i made that under boot no change at allYeah, it needs to be in
I haven't seen it where one of those wouldn't do the trick. If it's still not working, my next try would be to throw some debug lines (log, dd, ray, whatev) into the main method and also into the configureUsing closure to verify if it's actually getting called or not. (Note: I'm not a Filament pro by any stretch.)
bootstrap/providers.php
.I haven't seen it where one of those wouldn't do the trick. If it's still not working, my next try would be to throw some debug lines (log, dd, ray, whatev) into the main method and also into the configureUsing closure to verify if it's actually getting called or not. (Note: I'm not a Filament pro by any stretch.)
It gets called out i see the dump on the page but it still gets called before the component is actually made
it goes on and on but i cut it so i don't flood
Thanks for trying to help me still
According to the docs, you can also put global configureUsing() in a middleware. I've never tried that but it's worth a shot
Ok so i made a middleware with a
configureUsing()
method and added it to $panel->middleware([])
inside AdminPanelProvider but the debug no longer appears on the page so it doesn't seem like it cares one bit
Moved it to handle(), debug is back but it still always returns falseDid you ever overcome the issue yourself with either of those methods ?
Im afraid i'll have to use something like
Edit macros doesn't work for that either
All I needed to do was move from boot() to register() and magic happened
Is your code opensource so i can take a look at it ?
It's not open source or posted anywhere but I can share the config stuff. It's pretty basic, though. I'll pop it up here in just a sec.
bootstrap/providers.php
app/Providers/ConfigurationServiceProvider.php
In this app, the RichEditor config works great. But the logo change wasn't happening when it was in boot(). Moved it to register(0 and it worked fine.
I see, that's what i'v done without success, it works for you cause its setting "hardcoded" stuff whereas i need to check if the $component has the
bool isPassword = true
Also you should put
under your PanelProvider instead of a register method
@see
Assuming you are using Filament 3.xD'oh! I mentally glossed over the part where you were trying to use the component object. Sorry for that irrelevant wild goose chase. (facepalm)
I may make that change when I pick that project back up. It's not my active one at the moment. But it's working for now.
Don't worry we've tried stuff and even though it didn't work we both learned move Filament so i guess kinda win ?
Yeah if it ain't broke don't fix it 🤪
Well lets forget the whole override thing imagine i want to use instead
the fields still aren't revealable() even though that var is true
@see
Also i don't know why but they are not revealable() by default unless i tell the component to be even if (bool) $panel->arePasswordsRevealable = true
I had similar use case, I tried to reveal input text by using type as password, but the problem then causing google chrome asking to save password all the time. Then I decided to reveal in different way. I set macro in AppServiceProvider register function `
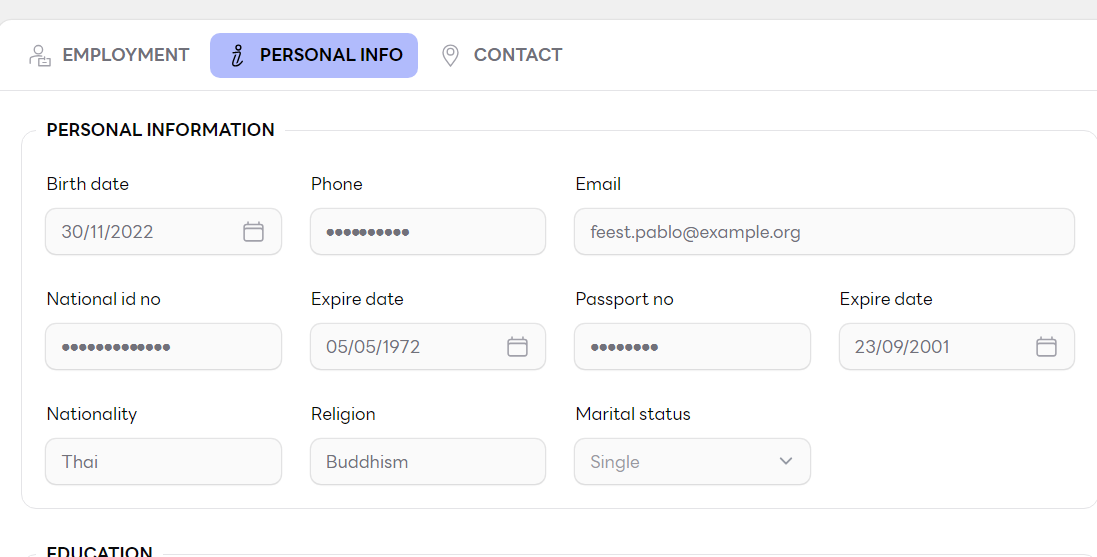
@LeandroFerreira eu preciso definir um logo diferente na tela de login customizada. Alguns exemplos que encontrei dizem que na tela "customizada", basta sobrescrever o método , mas ele não está disponível. Eu preciso diferenciar, pois o logo do Login é maior e se defino no provider, ele quebra o CSS da tela.
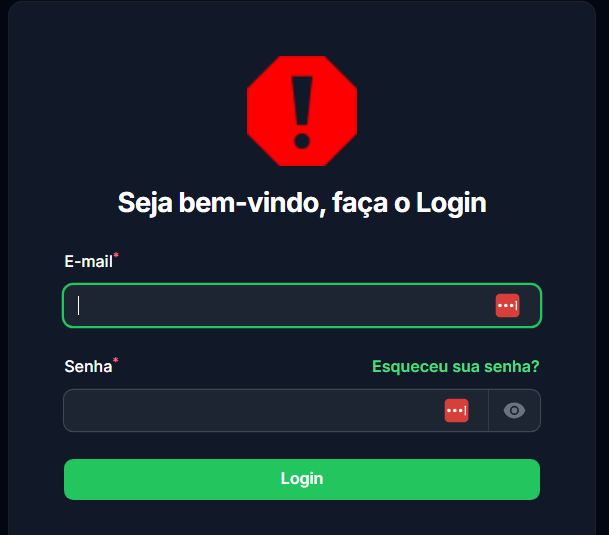
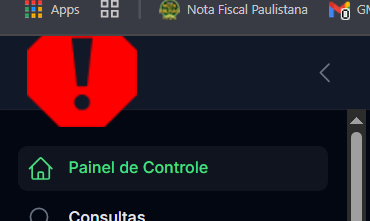
Meu login customizado.
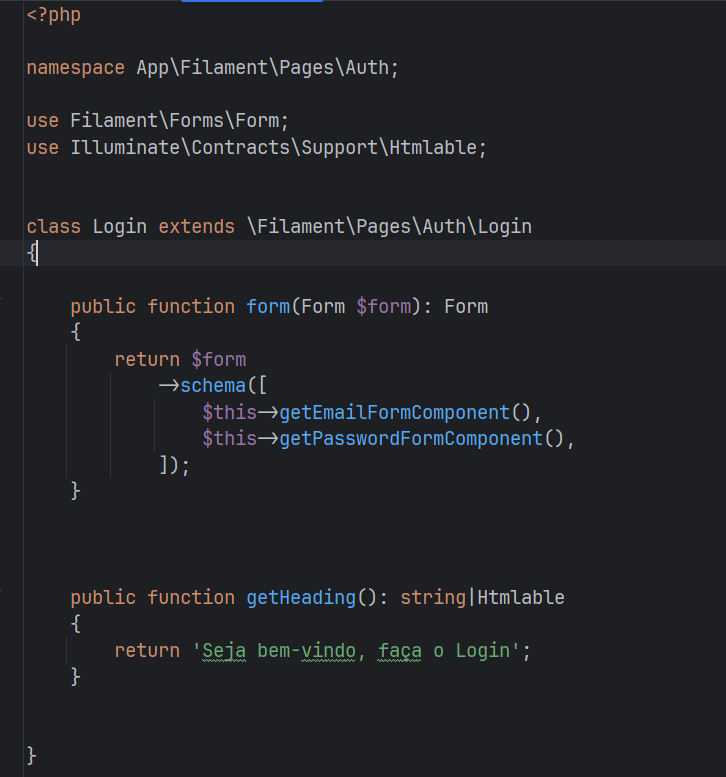
Mas o método getBrandLogo não tem na classe pai.
Pode me ajudar com dica de como resolver isso?
Valeu