checking if a string is empty.
Hey, i had a string that started off with the value
""
and wanted to check if it was empty. I first tried if (xyz.length <= 0) {...}
but found out that ""
equates to null
and that it wouldn't work due to this. i researched it and found you could do if (xyz)
which ended up working, this resource said that that tests for a value but i always thought that sort of if statement was for checking booleans (if (xyz)
being "if xyz = true").
Is this a recommended way to check for an empty string and is that a recommended check to do if it's not checking for a boolean? thanks in advance.17 Replies
If, when you run,
xyz.length
, it tells you "xyz
is null
", xyz
is not an empty string. It is null
.
''.length === 0
. Try opening the developer's tools console in the browser and pasting ''.length
in.
As for the if('')
thing, read up on "falsy values".to add to Dys's excellent answer, the answer here really is It Dependsβ’οΈ
falsey values are super important to understand though
Ah okay i see. Since
ββ
is considered falsey it worked in that context.
So according to dysβs link. If a falsey value is anything ranging from ββ
to null
to undefined
etc, itβs valid to test for it by doing if (xyz)
? The same with truthy values I guess?
It told me it was undefined. But i declared the variable as an empty string at the start of the script like let var = ββ
. So that means Iβm essentially declaring it as null if itβs considered not an empty string?
it really depends on what you want to accomplish down the line with that value
they all behave differently in different situations, so if you really have to know if something is an empty string, you use
value === ""
if you don't care, and it's just a check to see if something is vaguely false-like, then yeah, just checking the falsey value can be fineeven tho
if(string)
works fine, idk why i still find more peace at doing if(string && string.length > 0
π
when u do
if(something)
rather than any direct comparison (for example if(a === b)
) which just returns a boolean value), js converts the something
to boolean value
empty string is a falsy value hence if u do
the do_something()
won't runFair enough haha. Wouldnβt the string.length > 0 be redundant at that point though?
i mean that's the whole point that i was tryna make lol
Oh right i see now
it does become redundant but it still feels safer and gives internal peace π
Oh, tired brain haha. Canβt argue with that π
lol. no prob
js auto conversion is quite weird
there's stupid cases with falsey values, just beware
lol
i literally was just messing and found out
Number(["11"])
yields to number 11 π
2 step conversion
Hell yajust be really careful with how loosey goosey JS is with its types. There's a famous video that shows a bunch of dumb examples: https://www.destroyallsoftware.com/talks/wat
the JS part starts at 1:20-ish
that's interesting haha
thank you everyone for the help
it's possible for something to be truthy and have 0 length, like an array
empty arrays are truthy and falsey, so, checking the length isn't overkill
and since javascript doesn't have good type checking, it's possible to take an array of strings and your code wouldn't even notice it
as you can see, an empty array can be falsey, when compared to a boolean (if i had to bet, it's being converted to
[].join()
which is ""
) and it's truthy when using double-negative to type-duck it into a boolean (all objects are truthy, except null
)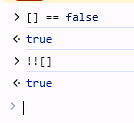