I have an excess of queries in my Filament Table.
I'm bulding a product manager for my application. I have two tables: products and variants. One product has many variants. I'm using Filament 3.2.118 on Laravel 11
And I have a resource Product:
`
In the model variant, I have a method thats format the price and more.
When I do this, Filament makes many queries to database: 37 (see image attachments).
It makes the correct queries with eager loading. But then, for each product row it lists in the table, it fires three queries to retrieve the relationship (I don't know why).
While I could set up my column like this:_
It doesn't work for me, because my Variant model has the methods to format the product price according to taxes and the type of currency associated with the product (currency and tax).
What could I be doing wrong or is there any other way to solve this?
Best,
Pablo
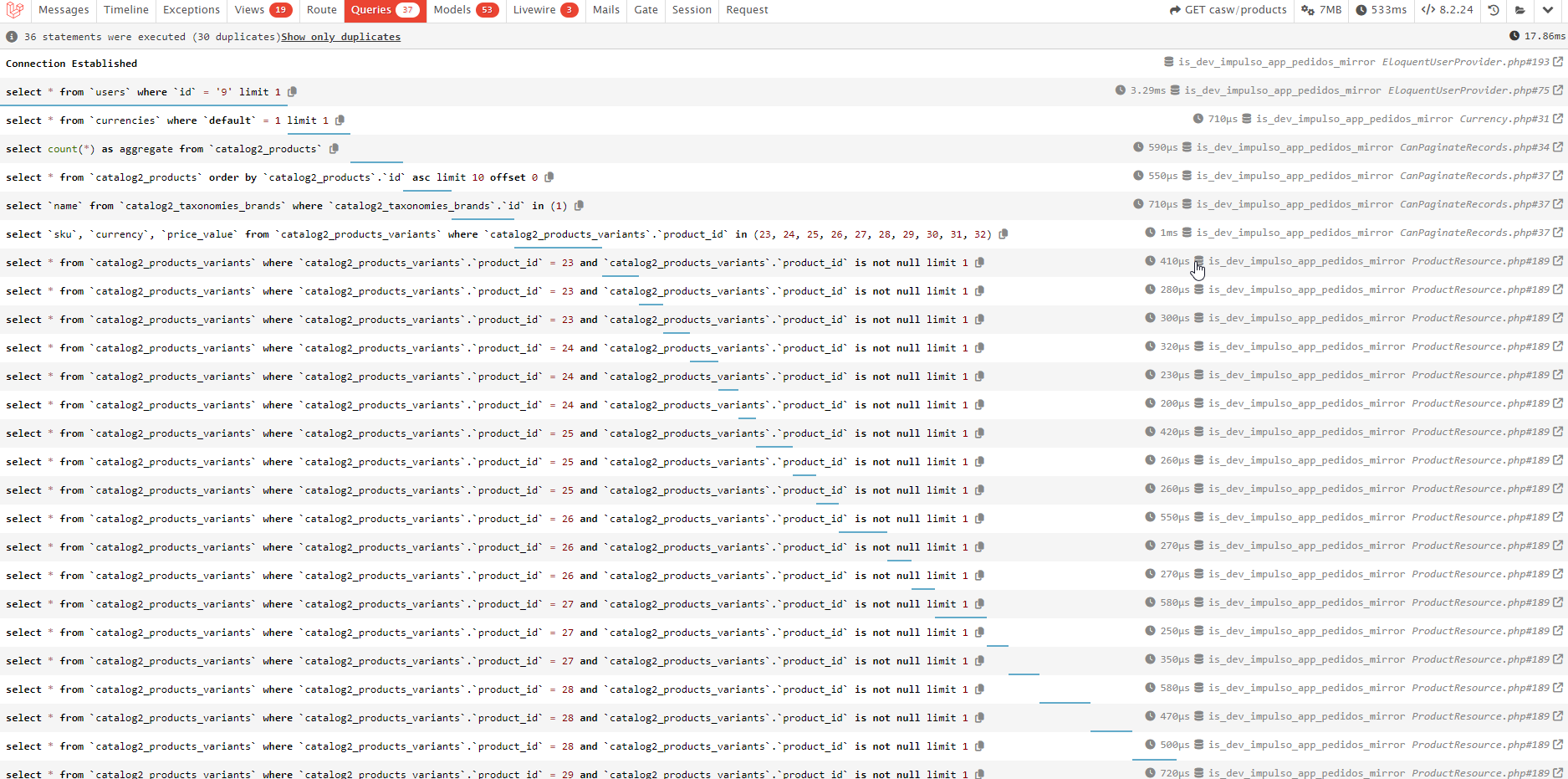
Solution:Jump to solution
I found the problem 🤦♂️:
I'm using:
```php
$record->variants()->first()->price_value...
2 Replies
You are calling them constantly with your state method....
surely you want:
or similar, it would depend what your priceFormatted did... but the above should be used to fetch the relationship and then formatState if you want to re-format the price display?
Also you can edger load the relationships.
Solution
I found the problem 🤦♂️:
I'm using:
instead of
In the first case, I'm getting a new instance of Query Builder for each row...
In the second case, I'm access to property variants with the related records loaded previously.